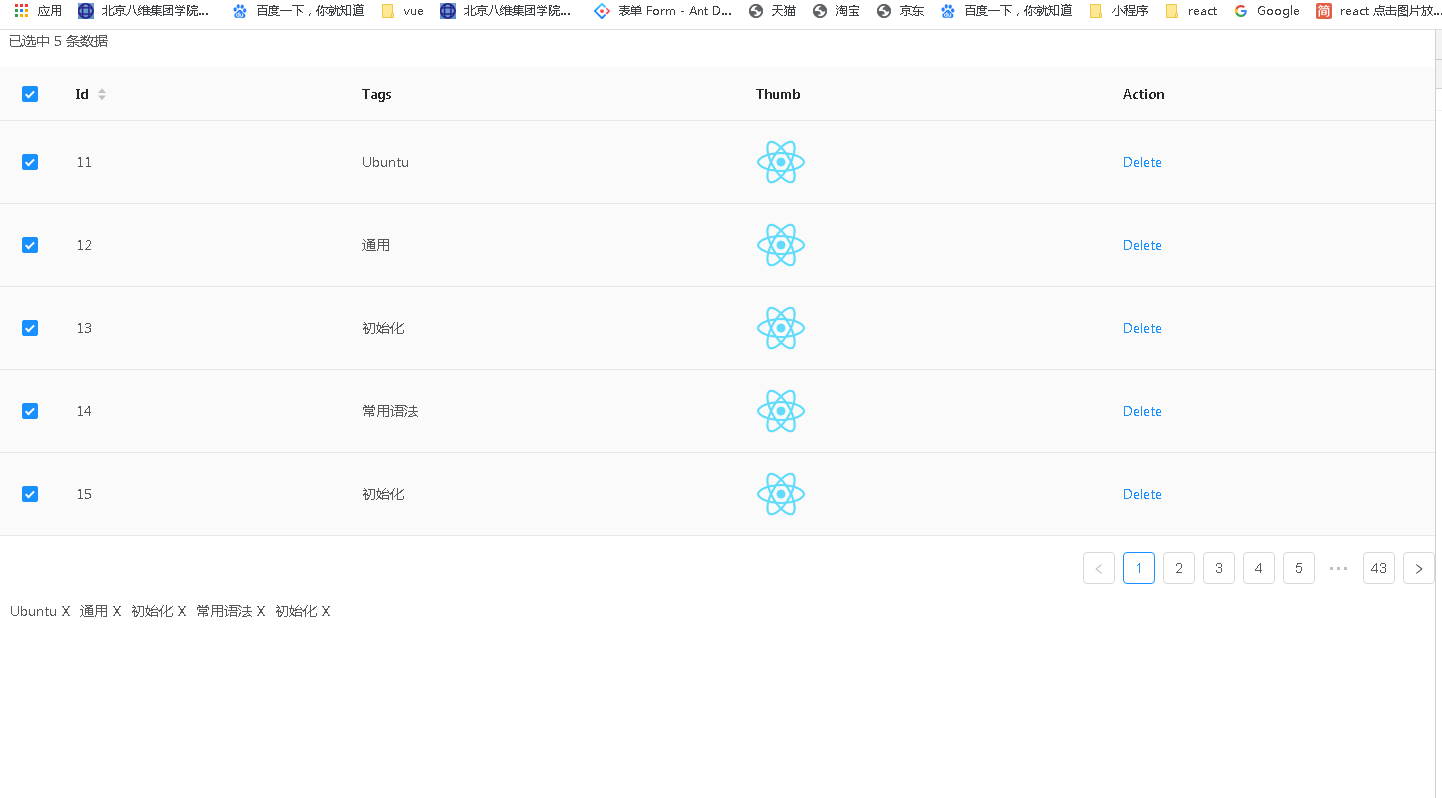
import React, { Component } from 'react'
import Axios from 'axios';
import qs from 'qs'
import api from '@/services/api'
import { Table } from 'antd';
export default class TableDemo extends Component {
state = {
selectedRowKeys: [],
selectedRows:[],
data: [],
total: ''
};
componentDidMount () {
this.initData(1)
}
initData(current){
Axios.post(api.limitData,qs.stringify({page: current, limit: 5})).then(res=>{ //这里是请求的自己的数据
this.setState({
data: res.data.result.list,
total: res.data.result.count
})
})
}
// 多选 //[id值] [{整行的数据},{整行的数据}]
onSelectChange = (selectedRowKeys, selectedRows) => {
this.setState({
selectedRowKeys,
selectedRows,
})
}
selectedRowsDelete = option => {
const { selectedRowKeys, selectedRows } = this.state
const checkedRowKeys = selectedRowKeys.filter( item => item !== option.id )
const checkedRows = selectedRows.filter( item => item.id !== option.id)
this.setState({
selectedRowKeys: checkedRowKeys,
selectedRows: checkedRows
})
}
// 删除
onDelete = option => {
// console.log(option)
}
// 获取分页器页码
limitData = current => {
this.initData(current)
}
render() {
const { selectedRows } = this.state
const columns = [
{
title: 'Id',
dataIndex: 'id',
sorter: (a, b) => a.id - b.id, //排序
},
{
title: 'Tags',
dataIndex: 'tags',
},
{
title: 'Thumb',
dataIndex: 'thumb',
render: record => (
<img src='/logo192.png' alt="" style={{width:'50px',height:'50px',borderRadius:'50%'}}/>
),
},
{
title: 'Action',
dataIndex: 'action',
render: (text, record) => (
<span>
<a onClick={()=>this.onDelete(text)}>Delete</a>
</span>
),
},
];
// 多选
const { selectedRowKeys } = this.state;
const rowSelection = {
selectedRowKeys,
onChange: this.onSelectChange,
getCheckboxProps: record => {
// console.log(record)
}
};
const hasSelected = selectedRowKeys.length > 0;
// 多选
// 分页器
const pagination = {
pageSize: 5,
onChange: this.limitData,
total: +this.state.total
}
return (
<>
<div style={{ marginBottom: 16 }}>
<span style={{ marginLeft: 8 }}>
{hasSelected ? `已选中 ${selectedRowKeys.length} 条数据` : ''}
</span>
</div>
<Table
rowKey="id"
columns={columns}
dataSource={this.state.data}
rowSelection={rowSelection} //checkbox
pagination={pagination} //分页器
/>
<div>
{
selectedRows.map( item =>{
return (
<span key={item.id} style={{ marginLeft: 10 }}>
{item.tags}
<i style={{ marginLeft: 5 }} onClick={()=>this.selectedRowsDelete(item)}>X</i>
</span>
)
})
}
</div>
</>
)
}
}