1.选定开发工具
选定eclipse为开发工具,用JAVA进行编程,实现此次测试。
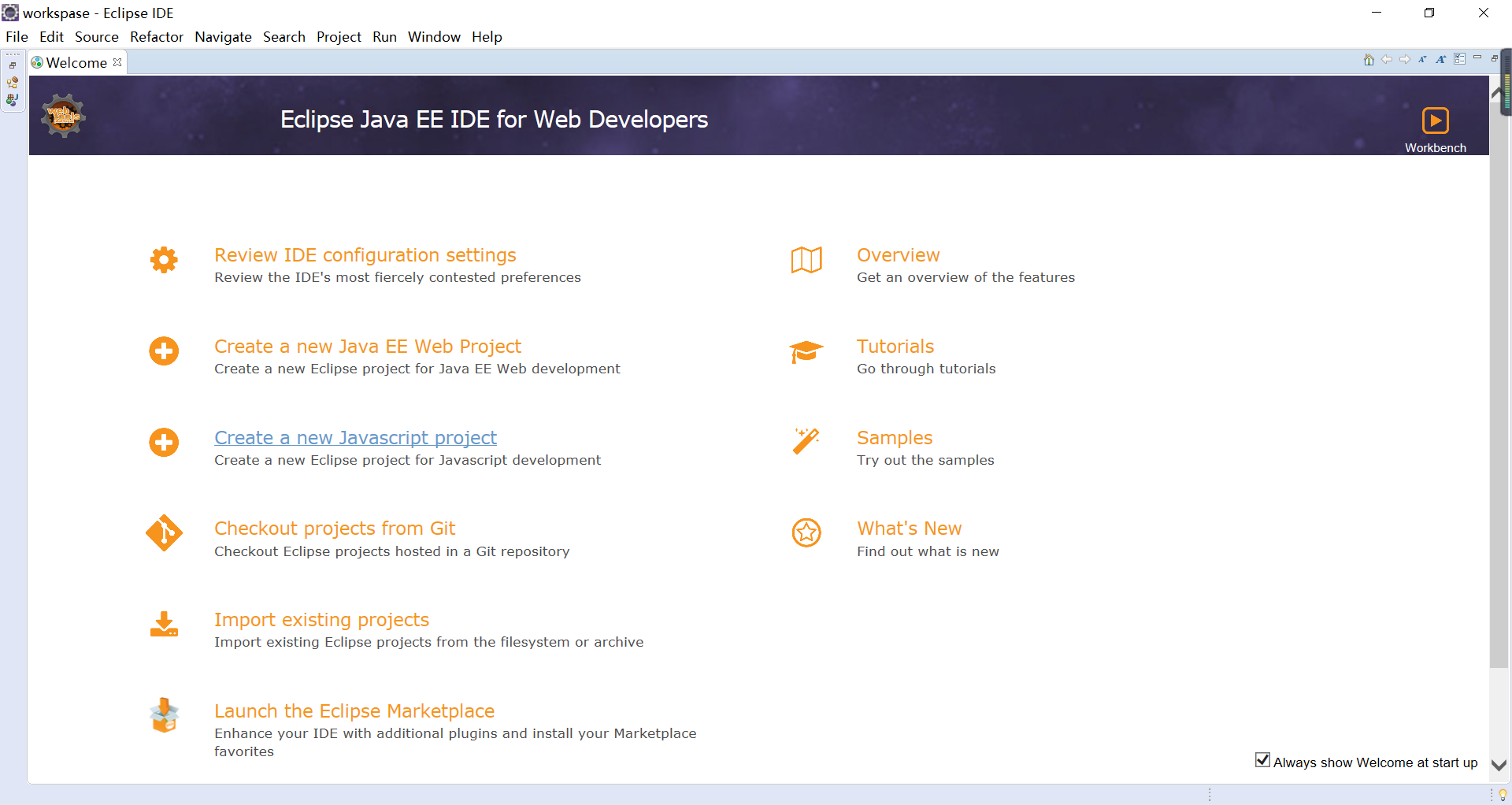2.编写需要被测试的java类
此次我们以顺序查找与二分查找法为例。
package com.mycode.tuils;
public class Search {
public int sqlSearch(int a,int[] arr,int b) //顺序查找
{
int i;
for(i=0;i<arr.length;i++)
{
if(a==arr[i])
{
b=i;
break;
}
}
return b;
}
public int binarySearch(int a,int[] arr,int b) //二分查找法("arr"为排序[升序]过后的数组)
{
int low=0;
int high=arr.length-1;
int mid;
while(low<=high)
{
mid = (low + high)/2;
if (arr[mid] == a)
{
b = mid;
break;
}
else if (arr[mid] < a)
low = mid + 1;
else if (arr[mid] > a)
high = mid - 1;
}
return b;
}
}
3.创建测试单元
(1)右键点击新建的project,选定Build Path->Add Library->JUnit->JUnit5
(2)创建新的Sound folder,命名为test(src->Sound floder)
(3)自动生成测试类,选定编写的被测试类Search()->NEW->orther
(4)点开orther,在Wizard中搜索JUnit->JUnit Test Case,如下图所示,修改图中红色标记处。
(5)点击下一步,选定所有被测试函数->Finish。
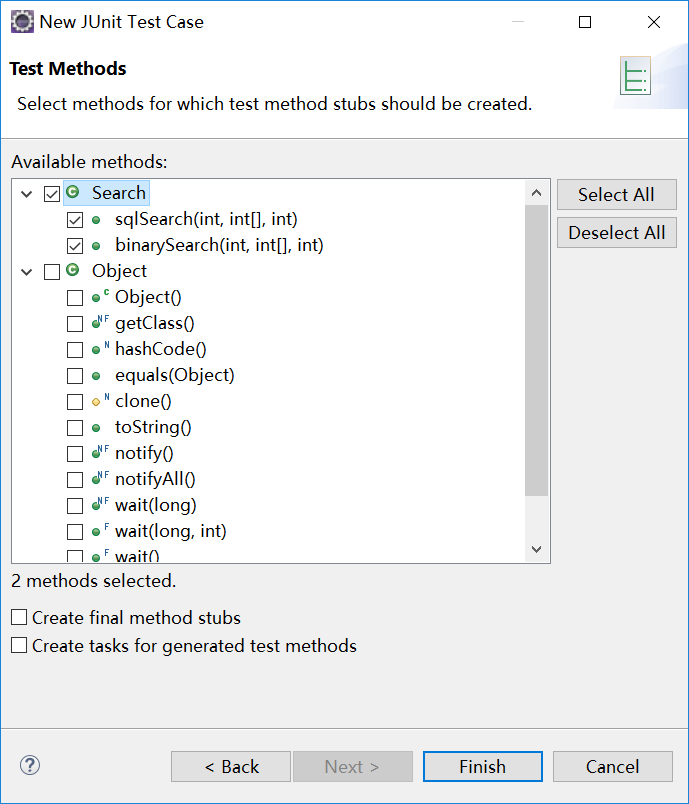4.编写测试类
package com.mycode.tuils;
import static org.junit.Assert.assertEquals;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class SearchAutoTest {
@Test
public void sqlSearch()
{
int[] arr= {1,5,8,6,11,25,36,42,15,85};
int a=8;
int b=0;
int c=2;
assertEquals(c,new Search().sqlSearch(a,arr,b));
}
@Test
public void binarySearch()
{
int[] arr= {1,5,6,8,11,15,25,36,42,85};
assertEquals(3,new Search().binarySearch(8,arr,0));
}
}
5.运行测试类
选定测试类->Run As->JUnit Test
##6.测试结果当Errors=0,与Failures=0,以及所有函数运行成功时说明此次测试成功。如下图所示。
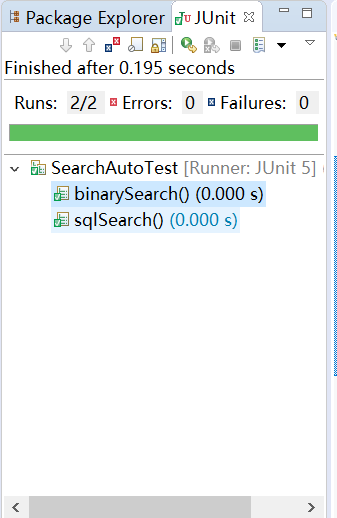###注意:
(1)每个测试函数前加@Test,以保证测试可以正常运行。
(2)测试类与被测试类所在的包的名字必须相同。
(3)编写被测试代码时先写主函数,保证程序的正常运行,在测试前再删除主函数。