1 #include <iostream>
2 #include "itkVectorGradientAnisotropicDiffusionImageFilter.h"
3 #include "itkVectorGradientMagnitudeImageFilter.h"
4 #include "itkWatershedImageFilter.h"
5
6 #include "itkImageFileReader.h"
7 #include "itkImageFileWriter.h"
8 #include "itkVectorCastImageFilter.h"
9 #include "itkScalarToRGBPixelFunctor.h"
10
11 int main( int argc, char *argv[] )
12 {
13 /*if (argc < 8 )
14 {
15 std::cerr << "Missing Parameters " << std::endl;
16 std::cerr << "Usage: " << argv[0];
17 std::cerr << " inputImage outputImage conductanceTerm diffusionIterations lowerThreshold outputScaleLevel gradientMode " << std::endl;
18 return EXIT_FAILURE;
19 }*/
20 /*现在我们声明图像和像素类型用来势力化滤波器。所有的滤波器都需要实数类型的像素
21 类型来正常工作。预处理阶段直接使用向量类型的数据而分割时使用浮点型标量数据。使用
22 itk::VectorCastImageFilter 来将图像从 RGB 像素类型转换为数字向量类型*/
23 typedef itk::RGBPixel< unsigned char > RGBPixelType;
24 typedef itk::Image< RGBPixelType, 2 > RGBImageType;
25 typedef itk::Vector< float, 3 > VectorPixelType;
26 typedef itk::Image< VectorPixelType, 2 > VectorImageType;
27 typedef itk::Image< itk::IdentifierType, 2 > LabeledImageType;
28 typedef itk::Image< float, 2 > ScalarImageType;
29 //使用上面创建的类型来声明各种图像域处理滤波器,并最终将它们用在处理过程中
30 typedef itk::ImageFileReader< RGBImageType > FileReaderType;
31 typedef itk::VectorCastImageFilter< RGBImageType, VectorImageType >
32 CastFilterType;
33 typedef itk::VectorGradientAnisotropicDiffusionImageFilter<
34 VectorImageType, VectorImageType >
35 DiffusionFilterType;
36 typedef itk::VectorGradientMagnitudeImageFilter< VectorImageType >
37 GradientMagnitudeFilterType;
38 typedef itk::WatershedImageFilter< ScalarImageType >
39 WatershedFilterType;
40
41 typedef itk::ImageFileWriter<RGBImageType> FileWriterType;
42
43 FileReaderType::Pointer reader = FileReaderType::New();
44 reader->SetFileName("VisibleWomanEyeSlice.png");
45
46 CastFilterType::Pointer caster = CastFilterType::New();
47 /*接下来我们实例化这些滤波器并设置它们的参数。第一步在图像预处理管道中使用一个
48 各向异性扩散滤波器来扩散输入彩色图像。对于这种滤波器类, CFL 条件要求对二维图像的
49 time step 不能超过 0.25 ,对三维不能超过 0.125 。迭代器的数量和 conductance term 将从命令行
50 得到。参见 6.7.3 小节将得到更多关于 ITK 各向异性扩散滤波器的信息*/
51 DiffusionFilterType::Pointer diffusion = DiffusionFilterType::New();
52 //设置迭代次数
53 diffusion->SetNumberOfIterations( atoi("10") );
54 //设置参数 conductance
55 diffusion->SetConductanceParameter( atof("2.0") );
56 diffusion->SetTimeStep(0.125);
57 //对向量类型图像 ITK 梯度大小滤波器可以随意地选择几个参数,这里我们仅允许那些主
58 //要成分分析的可用和不可用
59 GradientMagnitudeFilterType::Pointer
60 gradient = GradientMagnitudeFilterType::New();
61 //设置主要组成部分
62 gradient->SetUsePrincipleComponents(atoi("on"));
63 /*最后我们设置分水岭滤波器。它有两个参数。水平 Level 控制分水岭深度,而门限
64 Threshold 控制输入的最低门限值。这两个参数都作为输入图像中最大深度的一个百分比
65 (0.0 - 1.0) 来设置的*/
66 WatershedFilterType::Pointer watershed = WatershedFilterType::New();
67 //设置level
68 watershed->SetLevel( atof("0.2") );
69 /*分水岭滤波器的输出是一个无符号长整型 lable 图像,其中 lable 表示在一个特殊分割区域
70 中一个像素的成员关系。这种形式对视觉是不现实的,所以为了这个例子的目的,我们将把
71 它转换为 RGB 像素。 RGB 图像具有可以被保存为 png 文件和使用标准视图软件观看的优点。
72 itk::Functor::ScalarToRGBPixelFunctor 类是可以将一个标量值转换成一个 itk::RGBPixel 的一
73 个特殊功能对象。将这个算符写进 itk::UnaryFunctorImageFilter 来创建一个图像滤波器,用来
74 把标量图像转换成 RGB 图像*/
75 //设置阈值
76 watershed->SetThreshold( atof("0.01") );
77
78 typedef itk::Functor::ScalarToRGBPixelFunctor<unsigned long>
79 ColorMapFunctorType;
80 typedef itk::UnaryFunctorImageFilter<LabeledImageType,
81 RGBImageType, ColorMapFunctorType> ColorMapFilterType;
82 ColorMapFilterType::Pointer colormapper = ColorMapFilterType::New();
83
84 FileWriterType::Pointer writer = FileWriterType::New();
85 //保存的文件名
86 writer->SetFileName("VisibleWomanEyeSlice_FSL1.png");
87 //这种滤波器连接到一个单一的管道,在每个结尾都有 readers 和 writers
88 caster->SetInput(reader->GetOutput());
89 diffusion->SetInput(caster->GetOutput());
90 gradient->SetInput(diffusion->GetOutput());
91 watershed->SetInput(gradient->GetOutput());
92 colormapper->SetInput(watershed->GetOutput());
93 writer->SetInput(colormapper->GetOutput());
94
95 try
96 {
97 writer->Update();
98 }
99 catch (itk::ExceptionObject &e)
100 {
101 std::cerr << e << std::endl;
102 return EXIT_FAILURE;
103 }
104
105 return EXIT_SUCCESS;
106 }
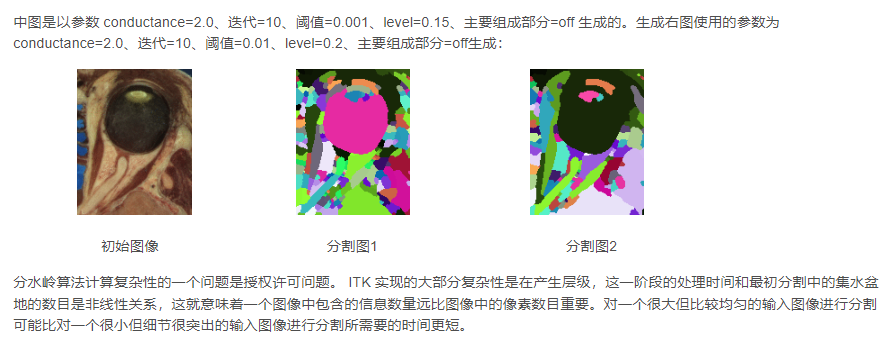