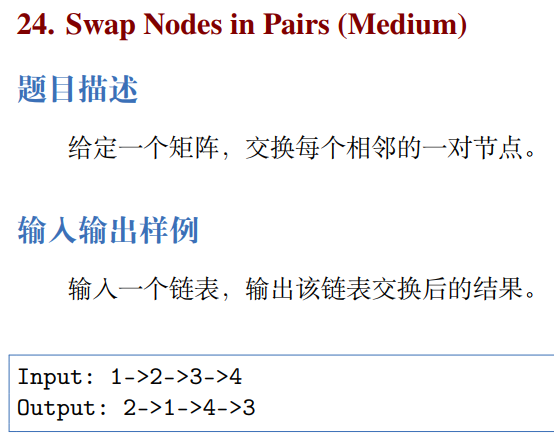
#include <iostream>
#include <stack>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) :val(x), next(nullptr) {}
};
ListNode* swapPairs1(ListNode* head) {
ListNode* dummyHead = new ListNode(0);
dummyHead->next = head;//使用虚拟头节点指向head
ListNode* cur = dummyHead;
while (cur->next && cur->next->next) {
ListNode* tmp = cur->next;
ListNode* tmp1 = cur->next->next->next;
cur->next = cur->next->next;
cur->next->next = tmp;
tmp->next = tmp1;
cur = cur->next->next;
}
return dummyHead->next;
}
int main() {
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(4);
head->next->next->next = new ListNode(8);
ListNode* swapList = swapPairs1(head);
std::cout << "Merged List: ";
printList(swapList);
// 释放内存
ListNode* current = swapList;
while (current) {
ListNode* next = current->next;
delete current;
current = next;
}
return 0;
}
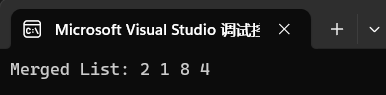