#include <iostream>
#include <string.h>
using namespace std;
typedef int SElemType;
typedef struct StackNode {
SElemType data;
struct StackNode* next;
}StackNode,*LinkStack;
//初始化链栈
void InitStack(LinkStack &S) {
S = NULL;
}
//入栈
bool EnterStack(LinkStack &S,int e) {
StackNode* p = new StackNode;
if (!p)return false;
p->data = e;
p->next = S;
S = p;
return true;
}
//出栈
bool PopStack(LinkStack& S, int &e) {
if (!S)return false;
e = S->data;
StackNode* q = S;
S = S->next;
delete q;
return true;
}
//取栈顶的元素
int GetTop(LinkStack& S) {
if (S) {
return S->data;
}
else {
return -1;
}
}
//打印输出栈元素
void PrintStack(LinkStack& S) {
LinkStack i = S;
cout << "链栈的元素为:";
while (i!=NULL) {
cout << i->data <<" ";
i = i->next;
}
cout << endl;
}
int main(void) {
LinkStack S;
int count = 0;//入栈元素个数
int count1 = 0;//出栈元素个数
int e = 0;
//初始化
InitStack(S);
//入栈
cout << "请输入入栈元素个数:";
cin >> count;
while (count > 0) {
cout << "请输入要入栈的元素:";
cin >> e;
if (EnterStack(S, e)) {
cout << "元素 " << e << " 入栈成功!" << endl;
//取栈顶元素
cout << "此时的栈顶元素为:" << GetTop(S) << endl;
}
else {
cout << "元素 " << e << " 入栈失败!" << endl;
}
count--;
}
//出栈
cout << "请输入要出栈的元素个数:";
cin >> count1;
while (count1>0) {
if (PopStack(S, e)) {
cout << "元素 " << e << " 出栈成功!" << endl;
count1--;
}
else {
cout << "元素 " << e << " 出栈失败!" << endl;
}
}
//打印输出剩余的栈元素
PrintStack(S);
system("pause");
return 0;
}
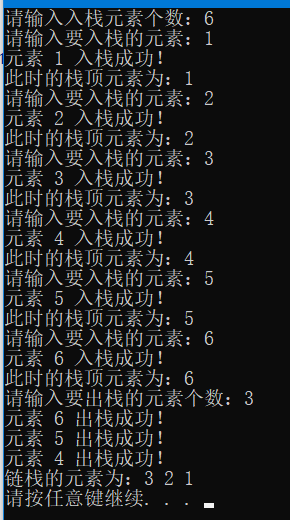