#include <iostream>
#include <string>
#include <stdlib.h>
#include <vector>
//#include <algorithm>
using namespace std;
//vector容器的简单应用
void demo1() {
vector<int> v1;
v1.push_back(4);
v1.push_back(2);
v1.push_back(4);
v1.push_back(3);
v1.push_back(4);
cout << "v1中的元素个数:" << v1.size() << endl;
cout << "v1中保存的元素:" << endl;
//第一种方式,数组访问
//for (int i = 0; i < v1.size(); i++) {
// cout << v1[i] << endl;
//}
//第二种方式,迭代器访问
vector<int>::iterator is = v1.begin();
for (; is != v1.end(); is++) {
cout << *is << endl;
}
//统计容器中某个元素的个数
int rcount = count(v1.begin(), v1.end(), 4);
cout << "v1中数值为4的元素一共有" << rcount << "个" << endl;
}
class Student {
public:
Student(int age, const char* name) {
this->age = age;
strncpy_s(this->name, name, 64);
cout << "调用了构造函数" << endl;
}
Student(const Student& s) {
this->age = s.age;
strncpy_s(this->name, s.name, 64);
cout << "调用了拷贝构造函数" << endl;
}
~Student() {
cout << "调用了析构函数" << endl;
}
int age;
char name[64];
};
void demo2() {
vector<Student *> s; //使用指针提高效率,此处不可用引用!
Student s1(12,"小王");
Student s2(23,"大王");
s.push_back(&s1);
s.push_back(&s2);
//第一种方式,数组访问
/*for (int i = 0; i < s.size(); i++) {
cout << (*s[i]).age<<":"<<(*s[i]).name << endl;
}*/
//第二种方式,迭代器访问
vector<Student *>::iterator it = s.begin();
for (; it != s.end(); it++) {
cout << (**it).name<<":"<<(**it).age << endl;
}
}
int main() {
demo1();
cout << endl;
demo2();
system("pause");
return 0;
}
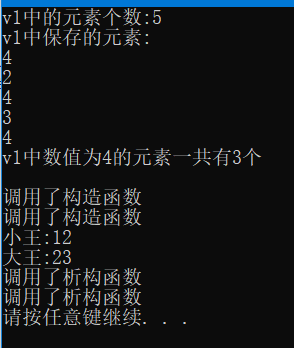