Book.h:
#pragma once
#include <string>
using namespace std;
class Book
{
public:
Book(const string& bookname, const string& isbn, double price);
double getPrice();
string getISBN();
string getBookname();
protected:
double price;
string ISBN;
string bookname;
};
Book.cpp:
#include "Book.h"
Book::Book(const string& bookname, const string& isbn, double price)
{
this->bookname = bookname;
this->ISBN = isbn;
this->price = price;
}
double Book::getPrice()
{
return price;
}
string Book::getISBN()
{
return ISBN;
}
string Book::getBookname()
{
return bookname;
}
Sellbook.h:
#pragma once
#include "Book.h"
#include <string>
using namespace std;
class Sellbook : public Book
{
public:
Sellbook(string bookname, string isbn, double price, double discount = 10.0);
void setDiscount(double discount);
double getDiscount();
double getPrice();
private:
double discount;
};
Sellbook.cpp:
#include "Sellbook.h"
Sellbook::Sellbook(string bookname, string isbn, double price, double discount ) :Book(bookname,isbn,price)
{
this->discount = discount;
}
void Sellbook::setDiscount(double discount)
{
this->discount = discount;
}
double Sellbook::getDiscount()
{
return discount;
}
double Sellbook::getPrice()
{
return price * discount * 0.1;
}
main.cpp:
#include <iostream>
#include <string>
#include "Book.h"
#include "Sellbook.h"
using namespace std;
int main() {
Book b1("C程序设计","02222",50);
Sellbook b2("C++程序设计","300012",24);
cout << b1.getBookname() << "的原价是:" << b1.getPrice() << ",书号是:" << b1.getISBN() << endl;
cout << b2.getBookname() << "的原价是:" << b2.getPrice() << ",书号是:" << b2.getISBN() << endl;
b2.setDiscount(5.0);
cout << b2.getBookname() << "的折扣是:" << b2.getDiscount() << endl;
cout << b2.getBookname() << "打折后的价格是:" << b2.getPrice() << ",书号是:" << b2.getISBN() << endl;
system("pause");
return 0;
}
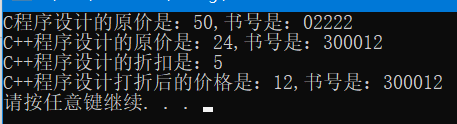