Father.h:
#pragma once
#include <iostream>
#include <string>
using namespace std;
class Father
{
public:
Father();
Father(const string &name,int age);
string getName();
int getAge();
string description();
private:
string name;
int age;
};
Father.cpp:
#include "Father.h"
#include <string>
#include <sstream>
using namespace std;
Father::Father() {
name = "小熊猫";
age = 10;
}
Father::Father(const string& name, int age) {
cout << __FUNCTION__ << endl;
this->name = name;
this->age = age;
}
string Father::getName() {
return name;
}
int Father::getAge() {
return age;
}
string Father::description() {
stringstream ret;
ret << "姓名:" << name << " 年龄:" << age ;
return ret.str();
}
Son.h:
#pragma once
#include <string>
#include "Father.h"
using namespace std;
class Son:public Father
{
public:
Son(const string& name,int age,const string& game);
string getGame();
string description();
private:
string game;
};
Son.cpp:
#include "Son.h"
#include <string>
#include <sstream>
using namespace std;
string Son::getGame() {
return game;
}
Son::Son(const string &name, int age, const string &game):Father(name,age) {
//若没有显式的调用父类的构造函数,那么将会自动调用父类的默认构造函数
cout << __FUNCTION__ << endl;
this->game = game;
}
string Son::description() {
stringstream ret;
ret << "姓名:" << getName() << " 年龄:" << getAge() << " 游戏:" << game;
return ret.str();
}
main.cpp:
#include <iostream>
#include <string>
#include "Father.h"
#include "Son.h"
int main() {
Father wjl("老王", 68);
Son wsc("小王",25,"电竞");
cout << wjl.description() << endl;
cout << wsc.description() << endl;
system("pause");
return 0;
}
运行结果:
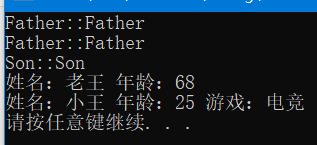