1.可以用简单的数据类型作为参数:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> data;
data.push_back(1);
data.push_back(5);
for (int i = 0; i < data.size(); i++) {
cout << data[i] << " ";
}
system("pause");
return 0;
}

2.也可以用一个类作为参数:
Boy.h:
#pragma once
#include <string>
using namespace std;
class Girl;
class Boy
{
public:
Boy();
Boy(int age,string name,int salary);
int getAge()const;
string getName()const;
int getSalary()const;
bool isSatisfied(const Girl& girl)const;
string description()const;
~Boy();
private:
int age;
string name;
int salary;
};
Girl.h:
#pragma once
#include <iostream>
using namespace std;
class Boy;
class Girl
{
public:
Girl();
Girl(string name,int age,int faceScore);
int getAge()const;
string getName()const;
int getFaceScore()const;
bool isSatisfied(const Boy& boy)const;
string description()const;
~Girl();
private:
int age;
string name;
int faceScore; //颜值
};
Boy.cpp:
#include <sstream>
#include "Boy.h"
#include "Girl.h"
#define SALARY_FACTORY 0.01
Boy::Boy()
{
age = 0;
name = "";
salary = 0;
}
Boy::Boy(int age, string name, int salary) {
this->age = age;
this->name = name;
this->salary = salary;
}
int Boy::getAge()const {
return age;
}
string Boy::getName() const {
return name;
}
int Boy::getSalary()const {
return salary;
}
bool Boy::isSatisfied(const Girl& girl)const {
if (girl.getFaceScore() >= salary * SALARY_FACTORY) {
return true;
}
else
{
return false;
}
}
string Boy::description()const {
stringstream ret;
ret << name << "-男-薪资(" << salary << ")-年龄(" << age << ")";
return ret.str();
}
Boy::~Boy() {
}
Girl.cpp:
#include "Girl.h"
#include "Boy.h"
#include <sstream>
#define FACESCORE_FACTORY 1
Girl::Girl() {
name = "";
age = 0;
faceScore = 0;
}
Girl::Girl(string name, int age, int faceScore) {
this->name = name;
this->age = age;
this->faceScore = faceScore;
}
int Girl::getAge()const {
return age;
}
string Girl::getName()const {
return name;
}
int Girl::getFaceScore()const {
return faceScore;
}
bool Girl::isSatisfied(const Boy& boy)const {
if (boy.getSalary() >= faceScore * FACESCORE_FACTORY) {
return true;
}
else
{
return false;
}
}
string Girl::description()const {
stringstream ret;
ret << name << "-女-颜值(" << faceScore << ")-年龄(" << age << ")";
return ret.str();
}
Girl::~Girl() {
}
主函数main.cpp:
#include <iostream>
#include <vector>
#include "Girl.h"
#include "Boy.h"
using namespace std;
int main() {
Boy boy1(26,"小刘",25000);
Boy boy2(24,"小王",15000);
Girl girl1("小花",25,13000);
Girl girl2("小美", 23, 10000);
vector<Boy> boys;
vector<Girl> girls;
boys.push_back(boy1);
boys.push_back(boy2);
girls.push_back(girl1);
girls.push_back(girl2);
for (int i = 0; i < boys.size(); i++) {
cout << boys[i].description() << endl;
}
for (int j = 0; j < girls.size(); j++) {
cout << girls[j].description() << endl;
}
system("pause");
return 0;
}
最后的实现结果:
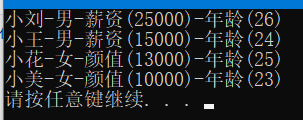