Description
Given the data of correct output file and the data of user's result file, your task is to determine which result the Judge System will return.
Input
Each test case has two parts, the data of correct output file and the data of the user's result file. Both of them are starts with a single line contains a string "START" and end with a single line contains a string "END", these two strings are not the data. In other words, the data is between the two strings. The data will at most 5000 characters.
Output
Sample Input
Sample Output
分析:
最好的方法就是将输入的数组去除空行 \n,\t然后再两两进行比较
代码:

#include<stdio.h> #include<string.h> const int maxn=5001; char s1[maxn],s2[maxn]; void input(char *s) { char temp[maxn]; gets(temp); while(strcmp(temp,"START")!=0) gets(temp); while(gets(temp)) { if(strcmp(temp,"END")==0) break; if(strlen(temp)!=0) strcat(s,temp); strcat(s,"\n"); } } void del(char *s,int l) { char temp[maxn]; int i,j; j=0; for(i=0;i<l;i++) { if(s[i]!=' '&&s[i]!='\n'&&s[i]!='\t') temp[j++]=s[i]; } temp[j]='\0'; strcpy(s,temp); } int sum() { int l1,l2; l1=strlen(s1); l2=strlen(s2); if(l1==l2&&strcmp(s1,s2)==0) return 1; del(s1,l1); del(s2,l2); if(strcmp(s1,s2)==0) return 0; else return -1; } int main() { int T,count; scanf("%d",&T); while(T--) { input(s1); input(s2); count=sum(); if(count==1) printf("Accepted\n"); else if(count==0) printf("Presentation Error\n"); else printf("Wrong Answer\n"); } return 0; }

#include<stdio.h> #include<string.h> const int maxn=5001; char s1[maxn],s2[maxn],t1[maxn],t2[maxn]; char temp[maxn]; void input(char *s,char *t) { gets(temp); while(strcmp(temp,"START")!=0) gets(temp); while(gets(temp)) { if(strcmp(temp,"END")==0) break; if(strlen(temp)!=0) strcat(s,temp); strcat(s,"\n"); } int k=0; int l=strlen(s); int i; for(i=0;i<l;i++) { if(s[i]!=' '&&s[i]!='\n'&&s[i]!='\t') t[k++]=s[i]; } t[k]='\0'; } int main() { int T; scanf("%d",&T); while(T--) { s1[0]='\0'; s2[0]='\0'; input(s1,t1); input(s2,t2); if(strcmp(s1,s2)==0) printf("Accepted\n"); else if(strcmp(t1,t2)==0) printf("Presentation Error\n"); else printf("Wrong Answer\n"); } return 0; }

#include <algorithm> #include <iostream> #include <cstring> #include <cstdlib> #include <vector> #include <cmath> #include <cstdio> using namespace std; #ifdef __int64 typedef __int64 LL; #else typedef long long LL; #endif const int inf=0x3f3f3f3f; const int maxn=5050; char t[maxn],as[maxn],bs[maxn],ap[maxn],bp[maxn]; int main() { int n,ca,cb; scanf("%d",&n); getchar(); while(n--) { gets(t); memset(t,0,sizeof(t)); memset(as,0,sizeof(as)); memset(bs,0,sizeof(bs)); memset(ap,0,sizeof(ap)); memset(bp,0,sizeof(bp)); ca=cb=0; while(gets(t)) { if(strcmp(t,"END")==0) break; strcat(as,t); ca++; } gets(t); while(gets(t)) { if(strcmp(t,"END")==0) break; strcat(bs,t); cb++; } if(ca==cb&&strcmp(as,bs)==0) printf("Accepted\n"); else { int la=strlen(as),lb=strlen(bs); int lla=0,llb=0; for(int i=0;i<la;i++) { if(as[i]!=' '&&as[i]!='\n'&&as[i]!='\t') ap[lla++]=as[i]; } for(int i=0;i<lb;i++) { if(bs[i]!=' '&&bs[i]!='\n'&&bs[i]!='\t') bp[llb++]=bs[i]; } if(strcmp(ap,bp)==0) printf("Presentation Error\n"); else printf("Wrong Answer\n"); } } return 0; }
Description
Note: The point P1 in the picture is the vertex of the parabola.
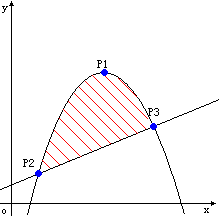
Input
Each test case contains three intersectant points which shows in the picture, they are given in the order of P1, P2, P3. Each point is described by two floating-point numbers X and Y(0.0<=X,Y<=1000.0).
Output
Sample Input
Sample Output
Hint
For float may be not accurate enough, please use double instead of float.
分析:
一道高数题 就是求出曲线和直线的方程在积分就好了!(曲线的方程可用顶点式 y = a(x-h)^2+l, (h,L) 为顶点坐标)
代码:
1.

#include<stdio.h> int main() { int n; double x1,x2,x3,y1,y2,y3; double s,a,b,c,k,h; while(~scanf("%d",&n)) { while(n--) { scanf("%lf %lf %lf %lf %lf %lf",&x1,&y1,&x2,&y2,&x3,&y3); a=(y2-y1)/((x2-x1)*(x2-x1)); b=-2*a*x1; c=y1-a*x1*x1-b*x1; k=(y2-y3)/(x2-x3); h=y2-k*x2; s=(a*(x3*x3*x3-x2*x2*x2)/3)+((b-k)*(x3*x3-x2*x2)/2)+((c-h)*(x3-x2)); printf("%.2lf\n",s); } } return 0; }
2.
这里我们设抛物线顶点式方程y=a*(x-h)^2+k;顶点坐标为(h,k)。h=x1;k=y1;a=(y-k)/(x-h)^2;
由积分可得(x2,x3)之间的面积,再减去梯形的面积即可

#include <algorithm> #include <iostream> #include <cstring> #include <cstdlib> #include <vector> #include <cstdio> #include <cmath> using namespace std; #ifdef __int64 typedef __int64 LL; #else typedef long long LL; #endif const int inf = 0x3f3f3f3f; const int maxn = 100000; int main() { int n; double x1,y1,x2,y2,x3,y3; scanf("%d",&n); while(n--) { scanf("%lf%lf%lf%lf%lf%lf",&x1,&y1,&x2,&y2,&x3,&y3); double b=x1; double c=y1; double a=(y2-c)/(x2-b)/(x2-b); double k=(y3-y2)/(x3-x2); double b1=y2-k*x2; double s1=a*x3*x3*x3/3-(2*a*b+k)*x3*x3/2+(a*b*b+c-b1)*x3; double s2=a*x2*x2*x2/3-(2*a*b+k)*x2*x2/2+(a*b*b+c-b1)*x2; printf("%.2f\n",s1-s2); } return 0; }
Description
Input
Output
Sample Input
Sample Output
分析:
解线性同余方程组
法一:
comes from lyminghao
a[i]<=10 and m <=10 . 所以我们可以枚举到{a[0],a[1],...a[n-1]}的lcm
代码:

#include <stdio.h> #include <string.h> #include <stdlib.h> #include <math.h> #include <string> #include <iostream> #include <algorithm> using namespace std; int gcd(int a,int b) { if(a<b) swap(a,b); if(b==0) return a; else return gcd(b,a%b); } int lcm(int x,int y) { return x*y/gcd(x,y); } int a[11],b[11]; int main() { int n,m; int cse; cin>>cse; while(cse--) { scanf("%d %d",&n,&m); for(int i=0;i<m;i++) scanf("%d",&a[i]); for(int i=0;i<m;i++) scanf("%d",&b[i]); int ans=a[0]; for(int i=1;i<m;i++) ans=lcm(ans,a[i]); int cnt=0; for(int i=0;i<=n&&i<=ans;i++) { for(int j=0;j<m;j++) { if(i%a[j]!=b[j]) break; if(j==m-1) cnt=(n-i)/ans+1; } } cout<<cnt<<endl; } return 0; }
注:gcd(x,y)为大整数的最大公约数
欧几里德算法(Euclid)阐述了一种gcd算法。gcd(greatest common divisor),简言之,我们想求gcd(x,y),假设(x>y),如果存在下式:x = q*y + r,那么则有gcd(x,y) = gcd(y,r) ,其实上式也称为gcd递归定理,即gcd(a,b) = gcd (b,a mod b)。
这个递归式看似很简单。实则它还是很值得推敲的,首先,它怎么证明?其次,该算法的运行时间为如何?
在密码学中,欧几里德算法有着相当广泛的应用,譬如求乘法逆元,大整数分解等等。。
在<<编程之美>>一书中,给出了不少gcd算法的简单实现。因为gcd算法的实现是递归,所以要特别注意栈溢出。先做个标记,以后会把栈详细分析一下。
最简单的gcd算法:

2

3

4

5

6

ACM中常用的gcd算法:

经过优化的gcd算法(分成奇偶两种情况):
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

lcm:最小公倍数
返回整数参数的最小公倍数。最小公倍数是所有整数参数 number1、number2 等等的最小正整数倍数。
法二:
中国剩余定理.
http://zh.wikipedia.org/wiki/%E4%B8%AD%E5%9B%BD%E5%89%A9%E4%BD%99%E5%AE%9A%E7%90%86
代码:

#include <algorithm> #include <iostream> #include <cstring> #include <cstdlib> #include <vector> #include <cstdio> #include <cmath> using namespace std; #ifdef __int64 typedef __int64 LL; #else typedef long long LL; #endif const int inf = 0x3f3f3f3f; const int maxn = 15; LL a[maxn],b[maxn]; LL exgcd(LL a,LL b,LL &x,LL &y) { if(b==0) { x=1; y=0; return a; } LL d=exgcd(b,a%b,x,y); LL tmp=x; x=y; y=tmp-a/b*y; return d; } int main() { int t,n,m; LL x,y,g,c; scanf("%d",&t); while(t--) { scanf("%d%d",&n,&m); for(int i=0; i<m; i++) { scanf("%I64d",&a[i]); } for(int i=0; i<m; i++) { scanf("%I64d",&b[i]); } for(int i=0; i<m; i++) { if(a[i]<=b[i]) { puts("0"); continue; } } bool flag=1; for(int i=1; i<m; i++) { g=exgcd(a[i-1],a[i],x,y); c=b[i]-b[i-1]; if(c%g) { flag=0; break; } x*=c/g; y*=c/g; a[i]=a[i-1]/g*a[i]; b[i]=(a[i-1]*x+b[i-1])%a[i]; b[i]=(b[i]+a[i])%a[i]; } if(flag==0||n<b[m-1]) { puts("0"); continue; } LL ans=(n-b[m-1])/a[m-1]+1; if(b[m-1]==0){ ans--; } printf("%I64d\n",ans); } return 0; }