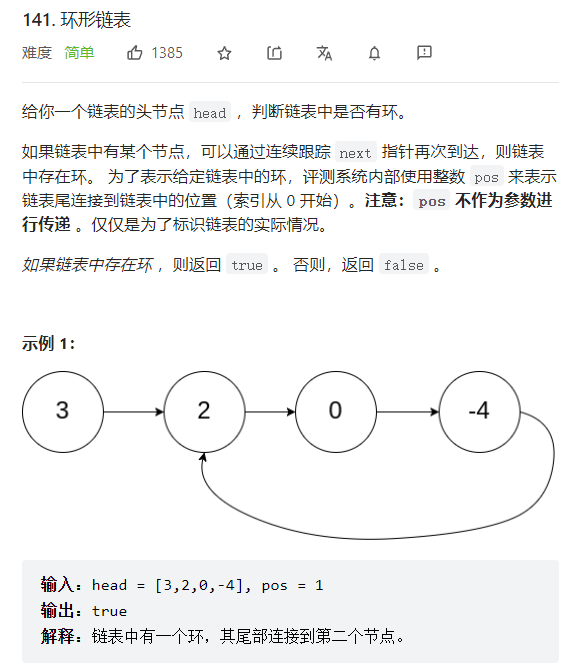
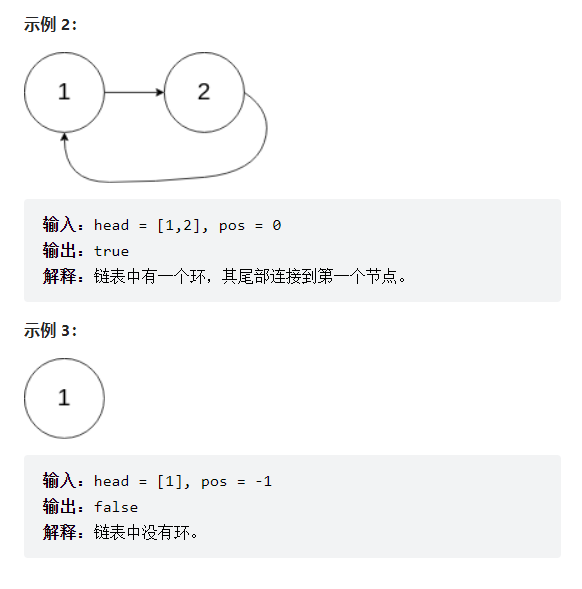
想法:
1:遍历链表,每次判断节点是否被访问过。(哈希表)
2:快慢指针(看题解之后)
两个指针pq都在head头指针开始(初始化);
快指针每次走两步,慢指针每次走一步,如果存在环,那么两指针一定会相遇,如果不存在环,快指针一定一直在慢指针前面;
1 官方代码: 2 public class Solution { 3 public boolean hasCycle(ListNode head) { 4 if (head == null || head.next == null) { 5 return false; 6 } 7 ListNode slow = head; 8 ListNode fast = head.next; 9 while (slow != fast) { 10 if (fast == null || fast.next == null) { 11 return false; 12 } 13 slow = slow.next; 14 fast = fast.next.next; 15 } 16 return true; 17 } 18 } 19 20 21 作者:LeetCode-Solution 22 链接:https://leetcode-cn.com/problems/linked-list-cycle/solution/huan-xing-lian-biao-by-leetcode-solution/ 23 来源:力扣(LeetCode) 24 著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
自己写的:
1 /** 2 * Definition for singly-linked list. 3 * class ListNode { 4 * int val; 5 * ListNode next; 6 * ListNode(int x) { 7 * val = x; 8 * next = null; 9 * } 10 * } 11 */ 12 public class Solution { 13 public boolean hasCycle(ListNode head) { 14 if((head != null)&&(head.next != null)){//空链表或单个节点直接返回false,不可能有环 15 ListNode p = new ListNode(); 16 ListNode q = new ListNode(); 17 p = head;q = head;//两个指针pq都在head头指针开始(初始化) 18 while((p != null)&&(q != null)){ 19 //当两个都不为空时继续,若其中一个为空还没遇到那也是不存在环 20 //快指针每次走两步,慢指针每次走一步 21 p = p.next; 22 if(q.next == null){return false;}//防止下一步报错 23 q = q.next.next; 24 if(p == q){//如果存在环,那么两指针一定会相遇,如果不存在环,快指针一定一直在慢指针前面 25 return true; 26 } 27 } 28 } 29 return false; 30 } 31 }