第一步添加必要dll (点击下方地址下载)
第二步添加一个WordHelper类,代码如下

using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.IO; using System.Reflection; using System.Text; using System.Data; using Word = Microsoft.Office.Interop.Word; //using iTextSharp.text.pdf; using System.Text.RegularExpressions; using System.Runtime.InteropServices; using System.Diagnostics; /// <summary> /// WordHelper 的摘要说明 /// </summary> public static class WordHelper { /* *64位系统配置组件服务权限,dcomcnfg.exe若找不到word组件先使用用comexp.msc -32打开 *标识选项卡中, 应选择"交互式用户 " */ private static object _missing = Missing.Value; #region Word操作 /// <summary> /// 打开Word.Document /// </summary> /// <param name="oWord"></param> /// <param name="ofileName"></param> /// <returns></returns> public static Word.Document OpenDocument(Word.Application oWord, object ofileName) { try { Word.Document oDoc = oWord.Documents.Open(ref ofileName, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing); //oWord.Activate(); //oWord.Visible = true; return oDoc; } catch (Exception ex) { Common.WriteExceptionLog(ex); } return null; } /// <summary> /// 书签替换 /// </summary> /// <param name="oWord"></param> /// <param name="name"></param> /// <param name="value"></param> public static void ReplaceBookMark(this Word.Document doc, string bookmark, string value) { if (doc.Bookmarks.Exists(bookmark)) { object bookmarkName = bookmark; Word.Bookmark bm = doc.Bookmarks.get_Item(ref bookmarkName); bm.Range.Text = value; } } /// <summary> /// 跳转到书签 /// </summary> public static void GoToBookMark(this Word.Document doc, string bookmark) { if (doc.Bookmarks.Exists(bookmark)) { object Nothing = _missing; object what = Word.WdGoToItem.wdGoToBookmark; object bookmarkName = bookmark; doc.ActiveWindow.Selection.GoTo(ref what, ref Nothing, ref Nothing, ref bookmarkName); } } /// <summary> /// 填充表格,注意传入的参数与表格的列数和顺序一直 /// 模板表中要有为空待填写的一行 /// </summary> /// <param name="doc"></param> /// <param name="tabIndex">表格索引</param> /// <param name="dt">填充数据</param> public static void FullTable(this Word.Document doc, int tabIndex, DataTable dt) { for (int i = 1; i <= dt.Rows.Count; i++) { if (i != dt.Rows.Count) { doc.Tables[tabIndex + 1].Rows[i + 1].Select(); doc.Application.Selection.InsertRows(1); } for (int j = 1; j <= dt.Columns.Count; j++) { doc.Tables[tabIndex + 1].Cell(i + 1, j).Range.Text = dt.Rows[i - 1][j - 1].ToString(); } } } /// <summary> /// 设置表格底色透明 /// 边框好看点 /// </summary> public static void SetTableColor(Word.Application oWord) { int tbc = oWord.ActiveWindow.Selection.Tables.Count; if (tbc > 0) { Word.Borders border = oWord.ActiveWindow.Selection.Tables[1].Borders; border.Enable = 1; border[Word.WdBorderType.wdBorderLeft].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderLeft].LineWidth = Word.WdLineWidth.wdLineWidth150pt; border[Word.WdBorderType.wdBorderLeft].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderRight].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderRight].LineWidth = Word.WdLineWidth.wdLineWidth150pt; border[Word.WdBorderType.wdBorderRight].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderTop].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderTop].LineWidth = Word.WdLineWidth.wdLineWidth150pt; border[Word.WdBorderType.wdBorderTop].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderBottom].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderBottom].LineWidth = Word.WdLineWidth.wdLineWidth150pt; border[Word.WdBorderType.wdBorderBottom].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderHorizontal].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderHorizontal].LineWidth = Word.WdLineWidth.wdLineWidth050pt; border[Word.WdBorderType.wdBorderHorizontal].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderVertical].LineStyle = Word.WdLineStyle.wdLineStyleSingle; border[Word.WdBorderType.wdBorderVertical].LineWidth = Word.WdLineWidth.wdLineWidth050pt; border[Word.WdBorderType.wdBorderVertical].Color = Word.WdColor.wdColorAutomatic; border[Word.WdBorderType.wdBorderDiagonalDown].LineStyle = Word.WdLineStyle.wdLineStyleNone; border[Word.WdBorderType.wdBorderDiagonalUp].LineStyle = Word.WdLineStyle.wdLineStyleNone; border.Shadow = false; oWord.Options.DefaultBorderColor = Word.WdColor.wdColorAutomatic; oWord.Options.DefaultBorderLineStyle = Word.WdLineStyle.wdLineStyleSingle; oWord.Options.DefaultBorderLineWidth = Word.WdLineWidth.wdLineWidth150pt; oWord.ActiveWindow.Selection.Tables[1].Shading.Texture = Word.WdTextureIndex.wdTextureNone; oWord.ActiveWindow.Selection.Tables[1].Shading.ForegroundPatternColor = Word.WdColor.wdColorAutomatic; oWord.ActiveWindow.Selection.Tables[1].Shading.BackgroundPatternColor = Word.WdColor.wdColorAutomatic; } } /// <summary> /// 添加分页符 /// </summary> /// <param name="oWord"></param> public static void AddBreak(this Word.Application oWord) { object myunit = Word.WdUnits.wdStory; oWord.Selection.EndKey(ref myunit, ref _missing); object pBreak = (int)Word.WdBreakType.wdPageBreak; oWord.Selection.InsertBreak(ref pBreak); } /// <summary> /// Word合并 /// </summary> /// <param name="_wordApp"></param> /// <param name="mainDoc"></param> /// <param name="tempDoc"></param> public static void Combine(Word.Application _wordApp, Word.Document mainDoc, Word.Document tempDoc) { Word.Range tempRange = tempDoc.Range(ref _missing, ref _missing); Word.Range mainRange = mainDoc.Range(ref _missing, ref _missing); tempRange.Select(); tempRange.Copy(); object end = Word.WdCollapseDirection.wdCollapseEnd; mainRange.Collapse(ref end); mainRange.Select(); mainRange.Paste(); CloseDocument(tempDoc); } /// <summary> /// 删除最后一页 /// </summary> /// <param name="oWord"></param> public static void DeleteLastPage(this Word.Application oWord) { object objWhat = Word.WdGoToItem.wdGoToPage; object objWhich = Word.WdGoToDirection.wdGoToLast; oWord.Selection.GoTo(ref objWhat, ref objWhich, ref _missing, ref _missing); Word.Range range1 = oWord.Selection.Range; Word.Range range2 = oWord.ActiveDocument.Range(ref _missing, ref _missing); object start = range1.Start; object end = range2.End; oWord.ActiveDocument.Range(ref start, ref end).Delete(ref _missing, ref _missing); } /// <summary> /// 删除最后一段 /// </summary> /// <param name="oWord"></param> /// <param name="oDoc"></param> public static void DeleteLastParagraph(this Word.Application oWord, Word.Document oDoc) { Word.Paragraph paragraph; Word.Paragraphs paragraphs = oDoc.Paragraphs; for (int i = paragraphs.Count; i > 0; i--) { paragraph = paragraphs[i]; if (paragraph.Range.Text.Trim() == string.Empty) { paragraph.Range.Select(); Word.Range range1 = paragraph.Range; object start = range1.Start; object end = range1.End; oWord.ActiveDocument.Range(ref start, ref end).Delete(ref _missing, ref _missing); } else { break; } } } /// <summary> /// 另存为 Word /// </summary> /// <param name="filename"></param> /// <returns></returns> public static void SaveAsDoc(this Word.Document oDoc, object filename) { object filefarmat = Word.WdSaveFormat.wdFormatDocument97; oDoc.SaveAs(ref filename, ref filefarmat, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing); } /// <summary> /// 另存为PDF /// </summary> /// <param name="filename"></param> /// <returns></returns> public static string SaveAsPdf(this Word.Document oDoc, object filename) { object savefilename = filename.ToString().Replace("doc", "pdf"); object filefarmat = Word.WdSaveFormat.wdFormatPDF; oDoc.SaveAs(ref savefilename, ref filefarmat, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing, ref _missing); return savefilename.ToString(); } /// <summary> /// 关闭 Word.Application /// </summary> public static void CloseApplication(Word.Application oWord, Word.Document oDoc) { if (oWord != null) { CloseDocument(oDoc); oWord.Quit(ref _missing, ref _missing, ref _missing); System.Runtime.InteropServices.Marshal.ReleaseComObject(oWord); oWord = null; } } /// <summary> /// 关闭Word文件 /// </summary> /// <param name="oDoc"></param> public static void CloseDocument(this Word.Document oDoc) { if (oDoc != null) { oDoc.Save(); oDoc.Close(ref _missing, ref _missing, ref _missing); oDoc = null; } } /// <summary> /// Word下载 /// </summary> /// <param name="oWord"></param> /// <param name="oDoc"></param> /// <param name="oFileName"></param> public static void DownLoadWord(Word.Application oWord, Word.Document oDoc, object oFileName) { string wordName = oFileName.ToString(); CloseApplication(oWord, oDoc); HttpResponse response = HttpContext.Current.Response; response.ContentType = "application/msword"; response.AddHeader("Content-Disposition", "attachment;filename=" + HttpUtility.UrlEncode(Path.GetFileName(wordName), Encoding.UTF8)); ; response.TransmitFile(wordName); } /// <summary> /// 杀word进程 /// </summary> public static void killAllProcess() { System.Diagnostics.Process[] myPs; myPs = System.Diagnostics.Process.GetProcessesByName("WINWORD.EXE"); foreach (System.Diagnostics.Process p in myPs) { if (p.Id != 0) { string myS = "WINWORD.EXE" + p.ProcessName + " ID:" + p.Id.ToString(); try { if (p.Modules != null) if (p.Modules.Count > 0) { System.Diagnostics.ProcessModule pm = p.Modules[0]; myS += "\n Modules[0].FileName:" + pm.FileName; myS += "\n Modules[0].ModuleName:" + pm.ModuleName; myS += "\n Modules[0].FileVersionInfo:\n" + pm.FileVersionInfo.ToString(); if (pm.ModuleName.ToLower() == "winword.exe") { p.Kill(); } } } catch { throw; } } } } /// <summary> /// 资源清理 /// </summary> /// <param name="oWord"></param> /// <param name="oDoc"></param> public static void Clear(Word.Application oWord, Word.Document oDoc) { CloseApplication(oWord, oDoc); Marshal.ReleaseComObject(oWord); killAllProcess(); } #endregion #region pdf操作 /// <summary> /// Pdf下载 /// </summary> /// <param name="filename"></param> public static void DownLoadPDF(string fileName) { HttpResponse response = HttpContext.Current.Response; response.ContentType = "application/PDF"; response.AddHeader("Content-Disposition", "attachment;filename=" + HttpUtility.UrlEncode(Path.GetFileName(fileName), Encoding.UTF8)); ; response.TransmitFile(fileName); } /// <summary> /// pdf只读加密 /// </summary> /// <param name="pdfSrc"></param> /// <param name="pdfDest"></param> //public static void PdfNoCopy(string pdfSrc, string pdfDest) //{ // pdfDest = HttpContext.Current.Server.MapPath(pdfDest); // PdfReader reader = new PdfReader(pdfSrc); // Stream os = (Stream)(new FileStream(pdfDest, FileMode.Create)); // PdfEncryptor.Encrypt(reader, os, null, null, PdfWriter.AllowScreenReaders | PdfWriter.AllowPrinting, false); //} #endregion }
第三步,也就是直接使用步骤了
using Microsoft.Office.Interop.Word; //引用命名空间
string modePath = AppDomain.CurrentDomain.BaseDirectory + "ContractManagement\\Files\\Model.doc"; string filePath = AppDomain.CurrentDomain.BaseDirectory + "ContractManagement\\Files\\" + DateTime.Now.ToString("yyyyMM"); if (!Directory.Exists(filePath)) Directory.CreateDirectory(filePath); Random rd = new Random(); string tempFileName = "\\" + DateTime.Now.ToString("yyyyMMddHHmmss") + rd.Next(1000).ToString("000") + ".doc"; string tempPath = filePath + tempFileName; File.Copy(modePath, tempPath, true);
--------------------上边代码都是废话重点看下边--------------------- Application app = new Application(); Document doc = WordHelper.OpenDocument(app, tempPath); //C#打开world doc.ReplaceBookMark("mark1", ""); //C#修改书签 mark1是模板world中的书签 System.Data.DataTable dt = new System.Data.DataTable(); //List<MyClass> list = new List<MyClass>(); //dt = ToDataTable<MyClass>(list);
//C#如果用到这步操作 获取ToDataTable<T>(IEnumerable<T> list)
//参考博主C#;DataTable添加列;DataTable转List泛型集合;List泛型集合转DataTable泛型集合; doc.FullTable(0, dt); //C#填充表格 doc.SaveAsPdf(tempPath.Replace(".doc", ".pdf")); //C#将world转换成PDF WordHelper.CloseApplication(app, doc); //C#关闭world
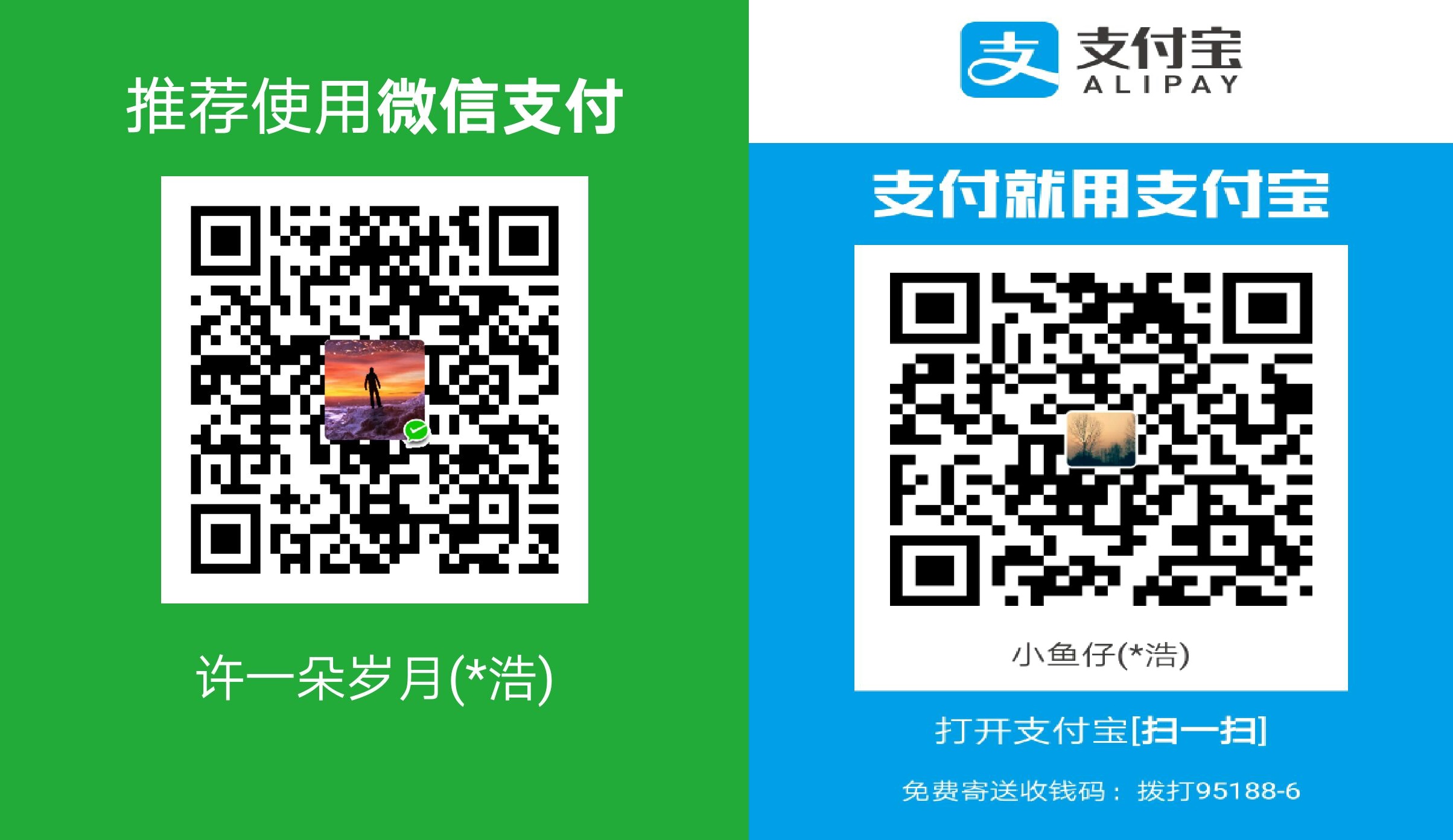
您的资助是我最大的动力!
金额随意,欢迎来赏!