实验任务4
Vector.hpp源代码
#pragma once
#include <iostream>
#include <stdexcept>
using namespace std;
template<typename T>
class Vector {
public:
Vector(int n);
Vector(int n, T value);
Vector(const Vector<T>& vi);
~Vector();
int get_size() const;
T& at(int index) const;
T& at(int index);
T& operator[](int index) const;
T& operator[](int index);
template<typename U>
friend void output(const Vector<U>& vi);
private:
int size;
T* ptr;
};
template<typename T>
void output(const Vector<T>& vi) {
for(int i=0;i<vi.size;i++) {
cout<<vi.at(i)<<", ";
}
cout<<endl;
}
template<typename T>
Vector<T>::Vector(int n):size(n) {
if(n<0)
throw length_error("Vector constructor: negative size");
ptr=new T[size];
}
template<typename T>
Vector<T>::Vector(int n, T value) : size(n) {
if(n<0)
throw length_error("Vector constructor: negative size");
ptr=new T[size];
for(int i=0;i<size;i++) {
ptr[i]=value;
}
}
template<typename T>
Vector<T>::Vector(const Vector<T>& vi):size(vi.size),ptr(new T[size]) {
for(int i=0;i<size;i++) {
ptr[i]=vi.ptr[i];
}
}
template<typename T>
Vector<T>::~Vector() {
delete[] ptr;
}
template<typename T>
int Vector<T>::get_size() const {
return size;
}
template<typename T>
T& Vector<T>::at(int index) const {
if(index<0||index>=size) {
throw out_of_range("Vector::at(): index out of range");
}
return ptr[index];
}
template<typename T>
T& Vector<T>::at(int index) {
return const_cast<T&>(static_cast<const Vector<T>*>(this)->at(index));
}
template<typename T>
T& Vector<T>::operator[](int index) const {
if (index < 0 || index >= size) {
throw out_of_range("Vector::operator[](): index out of range");
}
return ptr[index];
}
template<typename T>
T& Vector<T>::operator[](int index) {
return const_cast<T&>(static_cast<const Vector<T>*>(this)->operator[](index));
}
task4.cpp源代码
#include <iostream>
#include "Vector.hpp"
void test1() {
using namespace std;
int n;
cout << "Enter n: ";
cin >> n;
Vector<double> x1(n);
for(auto i = 0; i < n; ++i)
x1.at(i) = i * 0.7;
cout << "x1: "; output(x1);
Vector<int> x2(n, 42);
const Vector<int> x3(x2);
cout << "x2: "; output(x2);
cout << "x3: "; output(x3);
x2.at(0) = 77;
x2.at(1) = 777;
cout << "x2: "; output(x2);
cout << "x3: "; output(x3);
}
void test2() {
using namespace std;
int n, index;
while(cout << "Enter n and index: ", cin >> n >> index) {
try {
Vector<int> v(n, n);
v.at(index) = -999;
cout << "v: "; output(v);
}
catch (const exception &e) {
cout << e.what() << endl;
}
}
}
int main() {
cout << "测试1: 模板类接口测试\n";
test1();
cout << "\n测试2: 模板类异常处理测试\n";
test2();
}
运行测试截图
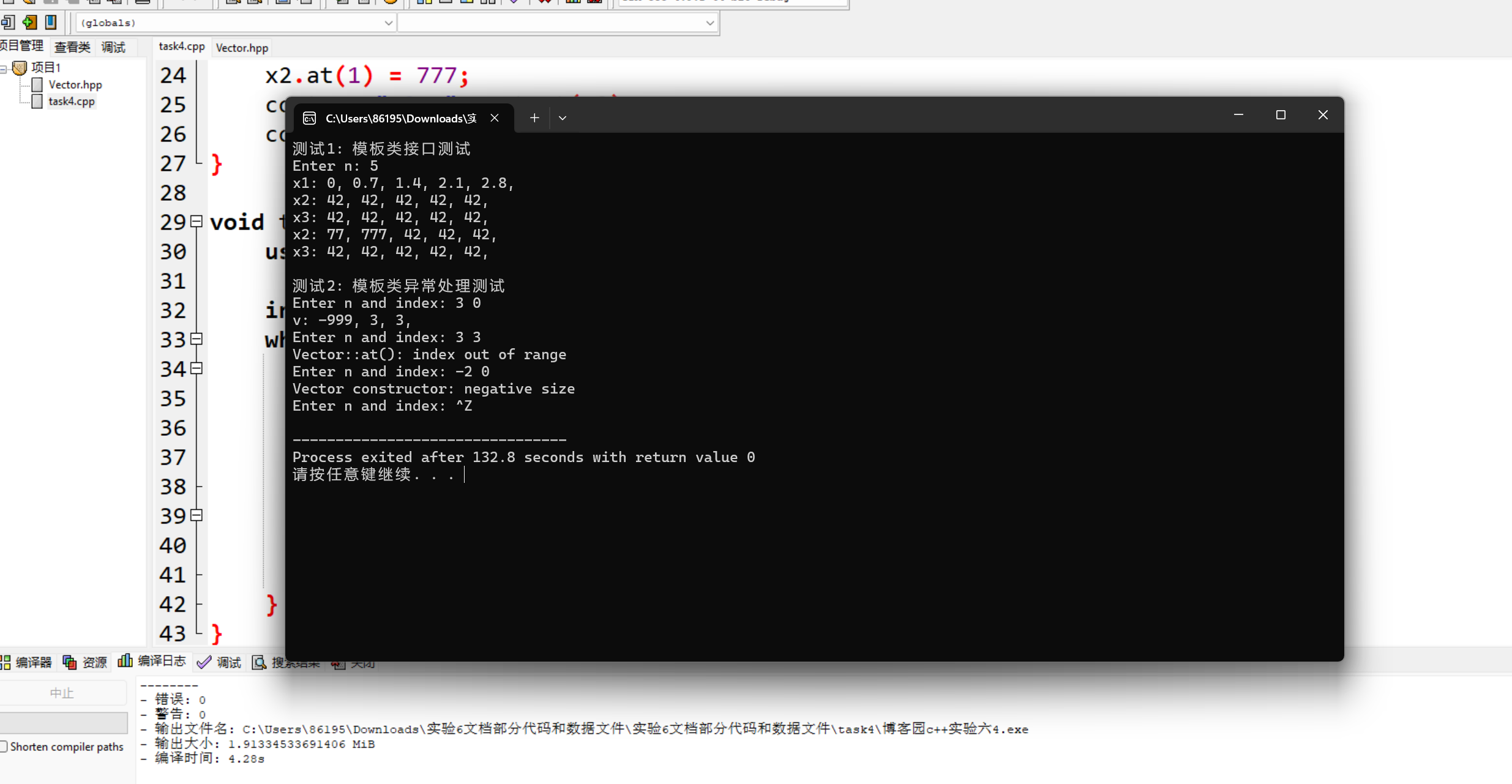
实验任务5
task5.cpp源码
#include<iostream>
#include<fstream>
#include<string>
#include<vector>
#include<iomanip>
#include<algorithm>
using namespace std;
class student{
public:
student()=default;
~student()=default;
string get_major()const {
return major;
}
int get_score()const {
return score;
}
friend ostream& operator<<(ostream& out,const student& s) {
out<<setiosflags(ios_base::left);
out<<setw(10)<<s.number
<<setw(10)<<s.name
<<setw(10)<<s.major
<<setw(10)<<s.score;
return out;
}
friend istream& operator>>(istream& in,student& s) {
in>>s.number>>s.name>>s.major>>s.score;
return in;
}
private:
string number;
string name;
string major;
int score;
};
bool compare(const student& s1,const student& s2) {
if (s1.get_major()<s2.get_major())
return true;
if (s1.get_major()==s2.get_major())
return s1.get_score()>s2.get_score();
return false;
}
void output(ostream& out,const vector<student>& v) {
for (auto& i:v)
out<<i<<endl;
}
void save(const string& filename,vector<student>& v) {
ofstream out(filename);
if (!out.is_open()) {
cout << "fail to open file to write\n";
return;
}
output(out, v);
out.close();
}
void load(const string& filename, vector<student>& v) {
ifstream in(filename);
if (!in.is_open()) {
cout<<"fail to open to read\n";
return;
}
string title_line;
getline(in, title_line);
student t;
while(in>>t)
v.push_back(t);
in.close();
}
int main() {
vector<student>v;
load("data5.txt", v);
sort(v.begin(),v.end(),compare);
output(cout, v);
save("ans5.txt", v);
}
运行结果截图
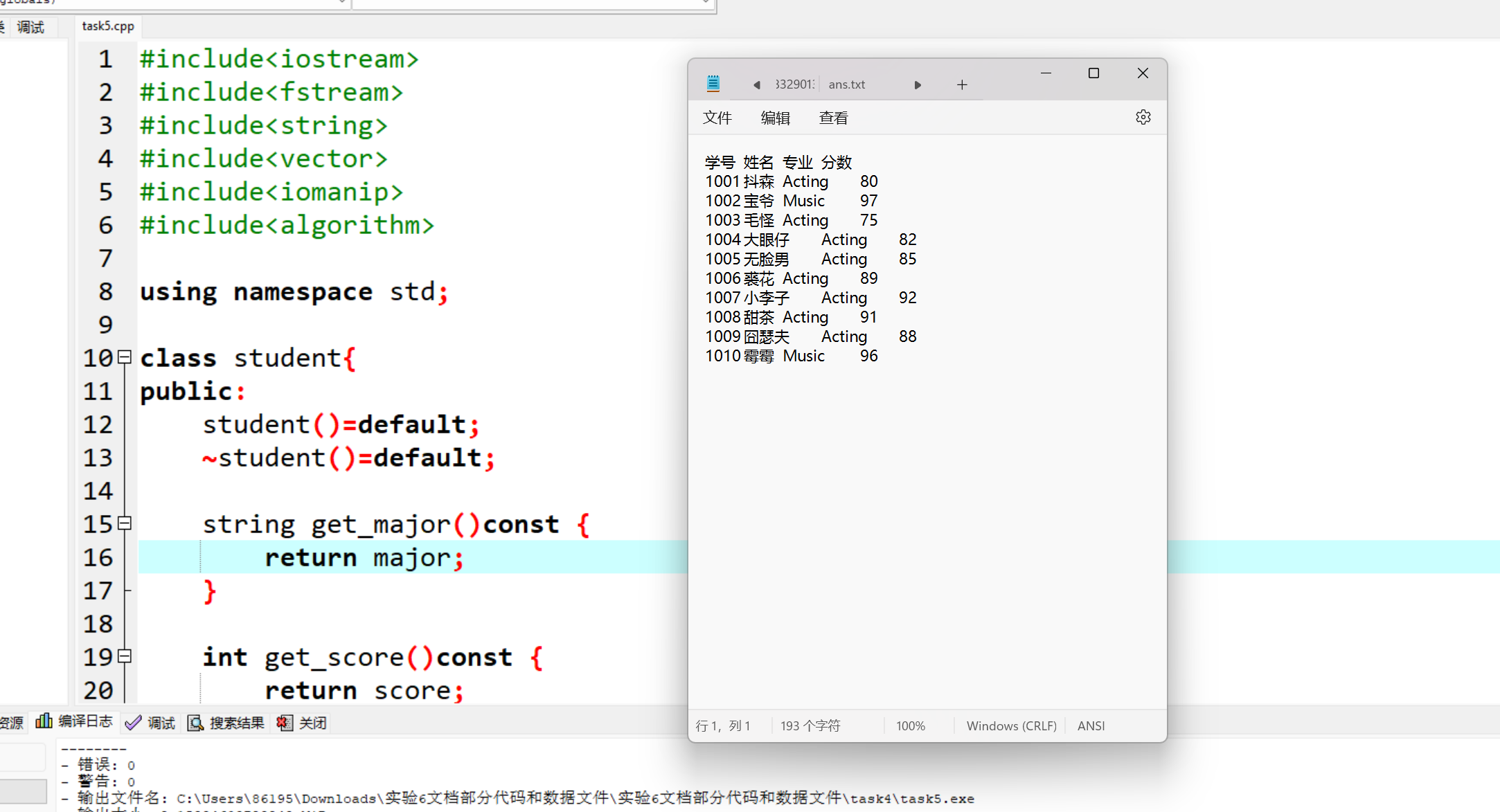