学生管理系统(springMVC)
《Java Web编程》课程设计
学生管理系统
完成日期: 2018年12月26日
1 项目引言
1.1 项目简介
学生入校后,我们需要管理这些学生,那么就需要我们对这些学生进行很多的操作,此时我们学校对学生有条理的管理,包括一些基本信息的记录,就方便了学生的信息管理。
1.2 使用技术
这是一个学生管理系统,应用的是SSH框架Spring+SpringMVC+Hibernate的项目,
运用的知识:
spring,springmvc,hibernate,Oracle
-
- 基本数据库知识Oracle
-
- Spring+SpringMVC+Hibernate
-
- (重点)框架的MVC设计模式的应用
-
- 部分前端代码(CSS和JavaScript的应用)
1.3 开发环境
操作系统:Win10系统
开发语言:JavaEE,JavaWeb。
开发工具:MyEclipse Professional 2014, navicat
2 需求分析
1)功能介绍:
-
- 增删改查学生。(test测试类中实现以及在jsp页面实现修改和删除)
-
- 增删改查页面显示当前学生信息(session里获取)
-
- 页面时间自动更新功能
-
- 部分前端代码
(3)项目构建
项目分包:MVC架构
controller:控制层,写SpringMvc的action
dao:数据层,Hibernate对数据的操作
entity:实体类和相应的*.hbm.xml(hibernate的类配置文件)
servicesDao:业务Dao,对单笔Dao进行业务封装
utils:工具类
3 概要设计
3.1 功能模块图
增删改查学生
增删改查页面显示当前用户信息(session里获取)
时间更新
- - 部分前端代码
如图3-1所示:
实现页面显示:
注册页面
登录页面:
系统主页面:
1 系统实现
思路:
实现源码:
1 package entity; 2 3 import java.io.Serializable; 4 //实现序列化接口 5 //实体类 6 public class Student implements Serializable{ 7 //要与表中字段一一对应 8 private Integer id; 9 private String name; 10 private String password; 11 private String truename; 12 private String sex; 13 public Integer getId() { 14 return id; 15 } 16 public void setId(Integer id) { 17 this.id = id; 18 } 19 public String getName() { 20 return name; 21 } 22 public void setName(String name) { 23 this.name = name; 24 } 25 public String getPassword() { 26 return password; 27 } 28 public void setPassword(String password) { 29 this.password = password; 30 } 31 public String getTruename() { 32 return truename; 33 } 34 public void setTruename(String truename) { 35 this.truename = truename; 36 } 37 public String getSex() { 38 return sex; 39 } 40 public void setSex(String sex) { 41 this.sex = sex; 42 } 43 @Override 44 public String toString() { 45 return "Student [id=" + id + ", name=" + name + ", password=" 46 + password + ", truename=" + truename + ", sex=" + sex + "]"; 47 } 48 public Student(Integer id, String name, String password, String truename, 49 String sex) { 50 super(); 51 this.id = id; 52 this.name = name; 53 this.password = password; 54 this.truename = truename; 55 this.sex = sex; 56 } 57 public Student() { 58 super(); 59 } 60 61 62 }
1 package dao; 2 3 import java.sql.Date; 4 import java.util.List; 5 6 import entity.Student; 7 // DAO层 8 public interface StudentDAO { 9 //添加一条数据到数据库中 10 public void insert (Student stu); 11 //查询所有数据 12 public List<Student> queryall(); 13 //查询单条数据 14 public Student query(String name); 15 //删除单条数据 16 public void delete(Integer id); 17 //修改一条数据 18 public void update(Student stu); 19 //查询单条数据 20 public Student queryone(Integer id); 21 //展示日期 22 public Date date(); 23 }
Mapper:
1 <?xml version="1.0" encoding="UTF-8"?> 2 <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > 3 <mapper namespace="dao.StudentDAO"> 4 <insert id="insert" parameterType="student"> 5 <selectKey order="BEFORE" resultType="int" keyProperty="id"> 6 select student_sql.nextval from dual 7 </selectKey> 8 insert into student values(#{id},#{name},#{password},#{truename},#{sex}) 9 </insert> 10 <select id="queryall" resultType="student"> 11 select * from student 12 </select> 13 <!-- 查询单挑数据 --> 14 <select id="query" resultType="student"> 15 select * from student where name=#{name} 16 </select> 17 <delete id="delete" parameterType="java.lang.Integer"> 18 delete from student where id=#{id} 19 </delete> 20 <!-- 修改一条数据 --> 21 <update id="update" parameterType="student"> 22 update student set name=#{name},password=#{password},truename=#{truename},sex=#{sex} where id=#{id} 23 </update> 24 <!-- 查询单个对象 --> 25 <select id="queryone" resultType="student" parameterType="java.lang.Integer"> 26 select * from student where id=#{id} 27 </select> 28 <select id="date" resultType="java.sql.Date"> 29 select sysdate from dual 30 </select> 31 </mapper>
Service:
1 package service; 2 3 import java.util.List; 4 5 import entity.Student; 6 7 public interface StudentService { 8 //注册账户 9 public void regester(Student stu); 10 //展示所有数据 11 public List<Student> showall(); 12 //登录用户 13 public Student login(String name); 14 //删除一个用户 15 public void delete(Integer id); 16 //修改数据 17 public void update(Student stu); 18 //查询单条数据 19 public Student query(Integer id); 20 //查询日期 21 public String found(); 22 }
Serviceimpl:
1 package service; 2 3 import java.text.SimpleDateFormat; 4 import java.util.List; 5 6 import org.springframework.beans.factory.annotation.Autowired; 7 import org.springframework.stereotype.Service; 8 import org.springframework.transaction.annotation.Transactional; 9 10 import dao.StudentDAO; 11 import entity.Student; 12 13 @Service 14 //为原始类对象添加事务(额外功能) 15 @Transactional 16 public class StudentServiceimpl implements StudentService{ 17 /* 18 * 原始类对象:原始方法,调用dao和编写逻辑代码 19 * */ 20 //将DAO接口定义成这个类中的成员变量 21 @Autowired 22 private StudentDAO dao; 23 24 public StudentDAO getDao() { 25 return dao; 26 } 27 //注册一条数据 28 public void setDao(StudentDAO dao) { 29 this.dao = dao; 30 } 31 32 public void regester(Student stu){ 33 System.out.println(stu); 34 dao.insert(stu); 35 } 36 37 //展示所有数据 38 public List<Student> showall(){ 39 List<Student> list = dao.queryall(); 40 return list; 41 } 42 43 //注册方法 44 public Student login(String name){ 45 Student s = dao.query(name); 46 return s; 47 } 48 49 //删除一条数据 50 public void delete(Integer id){ 51 dao.delete(id); 52 } 53 54 //修改一条数据 55 public void update(Student stu){ 56 dao.update(stu); 57 } 58 59 //查询一条数据 60 public Student query(Integer id){ 61 Student s = dao.queryone(id); 62 return s; 63 } 64 65 //展示日期 66 public String found(){ 67 java.sql.Date date = dao.date(); 68 //转换成为java date 69 java.util.Date da = new java.util.Date(date.getTime()); 70 SimpleDateFormat Format = new SimpleDateFormat("yyyy-MM-dd"); 71 String s = Format.format(da); 72 return s; 73 } 74 }
Controller层
Student:
1 package action; 2 3 import java.util.List; 4 5 import javax.servlet.http.HttpServletRequest; 6 7 import org.springframework.beans.factory.annotation.Autowired; 8 import org.springframework.stereotype.Controller; 9 import org.springframework.web.bind.annotation.RequestMapping; 10 11 import entity.Student; 12 import service.StudentService; 13 14 //添加@Controller注解指定这个类对象是我们的控制器 15 @Controller 16 //为控制器添加url访问路径名称 17 @RequestMapping("/student") 18 public class StudentController { 19 /* 20 * 1.收集数据 2.调用service层方法 3.流程跳转 21 */ 22 @Autowired 23 private StudentService service; 24 25 public StudentService getService() { 26 return service; 27 } 28 29 public void setService(StudentService service) { 30 this.service = service; 31 } 32 33 @RequestMapping("/add") 34 public String add(Student stu){ 35 System.out.println("---------------------------"); 36 System.out.println(stu); 37 System.out.println(service); 38 service.regester(stu); 39 System.out.println("---------------------------"); 40 return "login"; 41 } 42 43 //展示所有数据到jsp页面上 44 @RequestMapping("/show") 45 public String show(HttpServletRequest request){ 46 System.out.println("-----------------------------"); 47 List<Student> list = service.showall(); 48 request.setAttribute("list", list); 49 System.out.println("-----sfhfgthftg--------------------------"); 50 return "emplist"; 51 } 52 53 //登录控制器 54 @RequestMapping("/login") 55 public String login(Student stu,HttpServletRequest request,String code) { 56 System.out.println("--------------------------"); 57 //通过页面传来的name 去数据库中查询一个对象返回 58 Student student = service.login(stu.getName()); 59 //存入一个标记到作用域中,作为强制登录的判断条件 60 request.getSession().setAttribute("key",student); 61 // 判断student对象是否为空 62 if (student != null) { 63 if(request.getSession().getAttribute("code").equals(code)){ 64 if (student.getPassword().equals(stu.getPassword())) { 65 System.out.println("_---------------登录成功"); 66 return "forward:/student/show.do"; 67 } else { 68 System.out.println("密码错误-----------------"); 69 return "login"; 70 } 71 }else{ 72 System.out.println("验证码错误===================="); 73 return "login"; 74 } 75 } else { 76 System.out.println("============================用户不存在"); 77 return "register"; 78 } 79 80 } 81 82 // 删除一条数据 83 @RequestMapping("/delete") 84 public String delete(int id,HttpServletRequest request){ 85 if(request.getSession().getAttribute("key")!=null){ 86 service.delete(id); 87 return "forward:/student/show.do";}else{ 88 return "login"; 89 } 90 } 91 92 //修改一条数据 93 @RequestMapping("/update") 94 public String update(Student stu){ 95 service.update(stu); 96 return "forward:/student/show.do"; 97 } 98 //查询单个对象,并存入作用域跳转到修改页面 99 @RequestMapping("/select") 100 public String select(HttpServletRequest request){ 101 String i = request.getParameter("id"); 102 //字符串转换int 103 int id = Integer.parseInt(i); 104 Student student = service.query(id); 105 request.setAttribute("student",student); 106 return "updateEmp"; 107 } 108 }
Code:
1 package action; 2 3 import java.awt.image.BufferedImage; 4 import java.io.IOException; 5 import java.io.PrintWriter; 6 7 import javax.imageio.ImageIO; 8 import javax.imageio.stream.ImageOutputStream; 9 import javax.servlet.ServletOutputStream; 10 import javax.servlet.http.HttpServletResponse; 11 import javax.servlet.http.HttpSession; 12 13 import org.springframework.stereotype.Controller; 14 import org.springframework.web.bind.annotation.RequestMapping; 15 16 import util.SecurityCode; 17 import util.SecurityImage; 18 19 //添加注解使这个类对象成为控制器 20 @Controller 21 @RequestMapping("/code") 22 public class CodeController { 23 //固定public String 24 @RequestMapping("/code") 25 public void img(HttpServletResponse response, HttpSession session) throws IOException { 26 // 获得验证码字符串 27 String cool = SecurityCode.getSecurityCode(); 28 // 将验证码字符串存入到session作用域中,目的是为了判断时有条件可依 29 session.setAttribute("code", cool); 30 // 将验证码字符串组装拼接成验证码图片 31 BufferedImage image = SecurityImage.createImage(cool); 32 // 响应到客户端 33 ServletOutputStream out = response.getOutputStream(); 34 // 打印输出验证码到页面中 35 ImageIO.write(image, "png", out); 36 } 37 }
Util
securityCode:
1 package util; 2 3 import java.util.Arrays; 4 5 /** 6 * 7 * @author others 8 * date:2014-8-26 下午2:17:19 9 * 描述:随机生成验证码值:验证码串 10 */ 11 public class SecurityCode { 12 /** 13 * 验证码难度级别 14 * Simple-数字 15 * Medium-数字和小写字母 16 * Hard-数字和大小写字母 17 */ 18 public enum SecurityCodeLevel { 19 Simple, Medium, Hard 20 }; 21 /** 22 * 产生默认验证码,4位中等难度 23 * 24 * @return 25 */ 26 public static String getSecurityCode() { 27 return getSecurityCode(3, SecurityCodeLevel.Medium, false); 28 } 29 /** 30 * 产生长度和难度任意的验证码 31 * 32 * @param length 33 * @param level 34 * @param isCanRepeat 35 * @return 36 */ 37 private static String getSecurityCode(int length, SecurityCodeLevel level, boolean isCanRepeat) { 38 // 随机抽取len个字符 39 int len = length; 40 // 字符集合(--除去易混淆的数字0,1,字母l,o,O) 41 char[] codes = { 42 '2', '3', '4', '5', '6', '7', '8', '9', 43 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'm', 'n', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 44 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'J', 'K', 'L', 'M', 'N', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z' 45 }; 46 // 根据不同难度截取字符串 47 if (level == SecurityCodeLevel.Simple) { 48 codes = Arrays.copyOfRange(codes, 0, 10); 49 } else if (level == SecurityCodeLevel.Medium) { 50 codes = Arrays.copyOfRange(codes, 0, 36); 51 } 52 // 字符集和长度 53 int n = codes.length; 54 // 抛出运行时异常 55 if (len > n && isCanRepeat == false) { 56 throw new RuntimeException(String.format("调用SecurityCode.getSecurityCode(%1$s,%2$s,%3$s)出现异常," + "当isCanRepeat为%3$s时,传入参数%1$s不能大于%4$s", len, level, isCanRepeat, n)); 57 } 58 // 存放抽取出来的字符 59 char[] result = new char[len]; 60 // 判断能否出现重复字符 61 if (isCanRepeat) { 62 for (int i = 0; i < result.length; i++) { 63 // 索引0 and n-1 64 int r = (int) (Math.random() * n); 65 // 将result中的第i个元素设置为code[r]存放的数值 66 result[i] = codes[r]; 67 } 68 } else { 69 for (int i = 0; i < result.length; i++) { 70 // 索引0 and n-1 71 int r = (int) (Math.random() * n); 72 // 将result中的第i个元素设置为code[r]存放的数值 73 result[i] = codes[r]; 74 // 必须确保不会再次抽取到那个字符,这里用数组中最后一个字符改写code[r],并将n-1 75 codes[r] = codes[n - 1]; 76 n--; 77 } 78 } 79 return String.valueOf(result); 80 } 81 public static void main(String[] args) { 82 System.out.println(SecurityCode.getSecurityCode()); 83 } 84 }
securityImage:
1 package util; 2 3 import java.awt.Color; 4 import java.awt.Font; 5 import java.awt.Graphics2D; 6 import java.awt.image.BufferedImage; 7 import java.util.Random; 8 9 public class SecurityImage { 10 /** 11 * 生成验证码图片 12 * 13 * @param securityCode 14 * 15 * @return 16 * 17 */ 18 public static BufferedImage createImage(String securityCode) { 19 20 int codeLength = securityCode.length();// 验证码长度 21 22 int fontSize = 30;// 字体大小 23 24 int fontWidth = fontSize + 1; 25 26 // 图片宽高 27 28 int width = codeLength * fontWidth + 4; 29 30 int height = fontSize * 1 + 1; 31 32 // 图片 33 34 BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB); 35 36 Graphics2D g = image.createGraphics(); 37 38 g.setColor(Color.WHITE);// 设置背景色 39 40 g.fillRect(0, 0, width, height);// 填充背景 41 42 g.setColor(Color.LIGHT_GRAY);// 设置边框颜色 43 44 g.setFont(new Font("Arial", Font.BOLD, height - 2));// 边框字体样式 45 46 g.drawRect(0, 0, width - 1, height - 1);// 绘制边框 47 48 // 绘制噪点 49 50 Random rand = new Random(); 51 52 g.setColor(Color.LIGHT_GRAY); 53 54 for (int i = 0; i < codeLength * 6; i++) { 55 56 int x = rand.nextInt(width); 57 58 int y = rand.nextInt(height); 59 60 g.drawRect(x, y, 1, 1);// 绘制1*1大小的矩形 61 62 } 63 64 // 绘制验证码 65 66 int codeY = height - 10; 67 68 g.setColor(new Color(19, 148, 246)); 69 70 g.setFont(new Font("Georgia", Font.BOLD, fontSize)); 71 for (int i = 0; i < codeLength; i++) { 72 double deg = new Random().nextDouble() * 20; 73 g.rotate(Math.toRadians(deg), i * 16 + 13, codeY - 7.5); 74 g.drawString(String.valueOf(securityCode.charAt(i)), i * 16 + 5, codeY); 75 g.rotate(Math.toRadians(-deg), i * 16 + 13, codeY - 7.5); 76 } 77 78 g.dispose();// 关闭资源 79 80 return image; 81 82 } 83 84 }
Test:
1 package test; 2 3 import java.util.List; 4 5 import org.junit.Test; 6 import org.springframework.context.ApplicationContext; 7 import org.springframework.context.support.ClassPathXmlApplicationContext; 8 9 import service.StudentService; 10 import dao.StudentDAO; 11 import entity.Student; 12 13 public class StudentTest { 14 @Test 15 public void test(){ 16 ApplicationContext ctx = new ClassPathXmlApplicationContext("/applicationContext.xml"); 17 //通过接口名首字母小写作为beanid拿到的就是接口的实现类对象 18 StudentDAO dao = (StudentDAO)ctx.getBean("studentDAO"); 19 //dao.insert(new Student(10,"张明明","zmm","张明明","男")); 20 /*Student s = new Student(); 21 s.setId(11); 22 s.setName("樊小明"); 23 s.setPassword("fxm"); 24 dao.insert(s);*/ 25 /*List<Student> list = dao.queryall(); 26 for(Student student : list){ 27 System.out.println(student); 28 }*/ 29 //Student s = dao.query("张玉贤"); 30 //System.out.println(s); 31 //dao.delete(17); 32 dao.update(new Student(1,"贾克斯","jks","jia","男")); 33 //Student s = dao.queryone(8); 34 //System.out.println(s); 35 36 } 37 @Test 38 public void test2(){ 39 ApplicationContext ctx = new ClassPathXmlApplicationContext("/applicationContext.xml"); 40 StudentService s = (StudentService)ctx.getBean("studentServiceimpl"); 41 //s.regester(new Student(15,"金克斯","jks")); 42 /*List<Student> list = s.showall(); 43 for(Student student : list){ 44 System.out.println(student); 45 }*/ 46 //s.update(new Student(19,"老司机","lsj")); 47 //String ss = s.found(); 48 //System.out.println(ss); 49 50 51 } 52 }
jsp
Register:
1 <%@page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%> 2 <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> 3 <c:set var="baseurl" value="${pageContext.request.contextPath}"></c:set> 4 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 5 <html> 6 <head> 7 <title>regist</title> 8 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 9 <link rel="stylesheet" type="text/css" href="${baseurl}/css/style.css" /> 10 </head> 11 <body> 12 <div id="wrap"> 13 <div id="top_content"> 14 <div id="header"> 15 <div id="rightheader"> 16 <p> 17 <script type="text/javascript"> 18 var date=new Date(); 19 //toLocaleString() 获取本地时间格式的方法 20 document.write(date.toLocaleString()); 21 document.write("<hr size='3' color='blue'>"); 22 </script><br /> 23 </p> 24 </div> 25 <div id="topheader"> 26 <h1 id="title"> 27 <a href="#">main</a> 28 </h1> 29 </div> 30 <div id="navigation"></div> 31 </div> 32 <div id="content"> 33 <p id="whereami"></p> 34 <h1>注册</h1> 35 <form action="${pageContext.request.contextPath}/student/add.do" 36 method="post"> 37 <table cellpadding="0" cellspacing="0" border="0" 38 class="form_table"> 39 <tr> 40 <td valign="middle" align="right">用户名:</td> 41 <td valign="middle" align="left"><input type="text" 42 class="inputgri" name="name" /></td> 43 </tr> 44 <tr> 45 <td valign="middle" align="right">真实姓名:</td> 46 <td valign="middle" align="left"><input type="text" 47 class="inputgri" name="truename" /></td> 48 </tr> 49 <tr> 50 <td valign="middle" align="right">密码:</td> 51 <td valign="middle" align="left"><input type="password" 52 class="inputgri" name="password" /></td> 53 </tr> 54 <tr> 55 <td valign="middle" align="right">性别:</td> 56 <td valign="middle" align="left">男 <input type="radio" 57 class="inputgri" name="sex" value="男" checked="checked" /> 女 <input 58 type="radio" class="inputgri" name="sex" value="女" /> 59 </td> 60 </tr> 61 62 </table> 63 <p> 64 <input type="submit" class="button" value="Submit »" /> 65 </p> 66 </form> 67 </div> 68 </div> 69 <div id="footer"> 70 <div id="footer_bg">ABC@126.com</div> 71 </div> 72 </div> 73 </body> 74 </html>
Login:
1 <%@page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%> 2 <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> 3 <c:set var="baseurl" value="${pageContext.request.contextPath}"></c:set> 4 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 5 <html> 6 <head> 7 <title>login</title> 8 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 9 <link rel="stylesheet" type="text/css" href="${baseurl}/css/style.css" /> 10 </head> 11 12 <body> 13 <div id="wrap"> 14 <div id="top_content"> 15 <div id="header"> 16 <div id="rightheader"> 17 <p> 18 <script type="text/javascript"> 19 var date=new Date(); 20 //toLocaleString() 获取本地时间格式的方法 21 document.write(date.toLocaleString()); 22 document.write("<hr size='3' color='blue'>"); 23 </script><br /> 24 </p> 25 </div> 26 <div id="topheader"> 27 <h1 id="title"> 28 <a href="#">main</a> 29 </h1> 30 </div> 31 <div id="navigation"></div> 32 </div> 33 <div id="content"> 34 <p id="whereami"></p> 35 <h1>login</h1> 36 <form action="${pageContext.request.contextPath}/student/login.do" 37 method="post"> 38 <table cellpadding="0" cellspacing="0" border="0" 39 class="form_table"> 40 <tr> 41 <td valign="middle" align="right">username:</td> 42 <td valign="middle" align="left"><input type="text" 43 class="inputgri" name="name" /></td> 44 </tr> 45 <tr> 46 <td valign="middle" align="right">password:</td> 47 <td valign="middle" align="left"><input type="password" 48 class="inputgri" name="password" /></td> 49 </tr> 50 <tr> 51 <td valign="middle" align="right">验证码:<img id="num" src="${pageContext.request.contextPath}/code/code.do" /></td> 52 <td> <input type="text" name="code" /></td> 53 </tr> 54 </table> 55 <p align="left"> 56 <input type="submit" class="button" value="登陆 »" 57 style="height: 26px; " /> 58 </p> 59 </form> 60 </div> 61 </div> 62 <div id="footer"> 63 <div id="footer_bg">ABC@126.com</div> 64 </div> 65 </div> 66 </body> 67 </html>
Update:
1 <%@page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%> 2 <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> 3 <c:set var="baseurl" value="${pageContext.request.contextPath}"></c:set> 4 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 5 <html> 6 <head> 7 <title>update Emp</title> 8 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 9 <link rel="stylesheet" type="text/css" href="${baseurl}/css/style.css" /> 10 </head> 11 12 <body> 13 <div id="wrap"> 14 <div id="top_content"> 15 <div id="header"> 16 <div id="rightheader"> 17 <p> 18 <script type="text/javascript"> 19 var date=new Date(); 20 //toLocaleString() 获取本地时间格式的方法 21 document.write(date.toLocaleString()); 22 document.write("<hr size='3' color='blue'>"); 23 </script><br /> 24 </p> 25 </div> 26 <div id="topheader"> 27 <h1 id="title"> 28 <a href="#">Main</a> 29 </h1> 30 </div> 31 <div id="navigation"></div> 32 </div> 33 <div id="content"> 34 <p id="whereami"></p> 35 <h1>updateEmp info:</h1> 36 <form action="${pageContext.request.contextPath}/student/update.do" 37 method="post"> 38 <table cellpadding="0" cellspacing="0" border="0" 39 class="form_table"> 40 <tr> 41 <td valign="middle" align="right">id:</td> 42 <td valign="middle" align="left"><input type="text" 43 class="inputgri" name="id" value="${requestScope.student.id}" readonly="readonly"/></td> 44 </tr> 45 <tr> 46 <td valign="middle" align="right">name:</td> 47 <td valign="middle" align="left"><input type="text" 48 class="inputgri" name="name" value="${requestScope.student.name}" /></td> 49 </tr> 50 <tr> 51 <td valign="middle" align="right">truename:</td> 52 <td valign="middle" align="left"><input type="text" 53 class="inputgri" name="truename" 54 value="${requestScope.student.truename}" /></td> 55 </tr> 56 <tr> 57 <td valign="middle" align="right">password:</td> 58 <td valign="middle" align="left"><input type="text" 59 class="inputgri" name="password" 60 value="${requestScope.student.password}" /></td> 61 </tr> 62 <tr> 63 <td valign="middle" align="right">sex:</td> 64 <td valign="middle" align="left"><input type="text" 65 class="inputgri" name="sex" value="${requestScope.student.sex}" /></td> 66 </tr> 67 </table> 68 <p> 69 <input type="submit" class="button" value="修改" /> 70 </p> 71 </form> 72 </div> 73 </div> 74 <div id="footer"> 75 <div id="footer_bg">ABC@126.com</div> 76 </div> 77 </div> 78 </body> 79 </html>
Emplist:
1 <%@page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%> 2 <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> 3 <c:set var="baseurl" value="${pageContext.request.contextPath}"></c:set> 4 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 5 <html> 6 <head> 7 <title>emplist</title> 8 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 9 <link rel="stylesheet" type="text/css" href="${baseurl}/css/style.css" /> 10 </head> 11 <body> 12 <div id="wrap"> 13 <div id="top_content"> 14 <div id="header"> 15 <div id="rightheader"> 16 <p> 17 <script type="text/javascript"> 18 var date=new Date(); 19 //toLocaleString() 获取本地时间格式的方法 20 document.write(date.toLocaleString()); 21 document.write("<hr size='3' color='blue'>"); 22 </script><br /> 23 </p> 24 </div> 25 <div id="topheader"> 26 <h1 id="title"> 27 <a href="#">main</a> 28 </h1> 29 </div> 30 <div id="navigation"></div> 31 </div> 32 <div id="content"> 33 <p id="whereami"></p> 34 <h1>Welcome!</h1> 35 <h1>尊敬的用户:${sessionScope.key.name}</h1> 36 <table class="table"> 37 <tr class="table_header"> 38 <td>ID</td> 39 <td>Name</td> 40 <td>Password</td> 41 <td>Truename</td> 42 <td>Sex</td> 43 <td>Operation</td> 44 </tr> 45 46 <c:forEach var="student" items="${list}"> 47 <tr> 48 <td>${student.id}</td> 49 <td>${student.name}</td> 50 <td>${student.password}</td> 51 <td>${student.truename}</td> 52 <td>${student.sex}</td> 53 <td> 54 <a href="${pageContext.request.contextPath}/student/delete.do?id=${student.id}">删除</a> 55 <a href="${pageContext.request.contextPath}/student/select.do?id=${student.id}">修改</a> 56 </td> 57 </tr> 58 </c:forEach> 59 60 61 </table> 62 </div> 63 </div> 64 <div id="footer"> 65 <div id="footer_bg">ABC@126.com</div> 66 </div> 67 </div> 68 </body> 69 </html>
结束语:
刚开始接触这个课题时,感觉很多问题接踵而至,不知所措,但是,在自己的努力和别人的帮助下下,还是成功了,心里很开心,很充实。这个课题研究的意义是,对数据库进行增删改查操作,这个课题给我带来了很大的收获。我在当中也学到了很多精神,刻苦奋斗,锲而不舍。人生不会再有第二个大学,我很珍惜在大学里的一切,也很怀念这一切。活在当下,珍惜当下,充实当下。
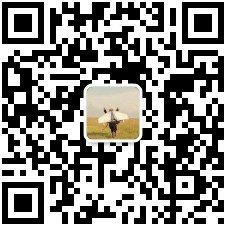
作者:泰斗贤若如
微信公众号:去有风的地方飞翔
Github:https://github.com/zyx110
有事微信:zyxt1637039050
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文链接,否则保留追究法律责任的权利。
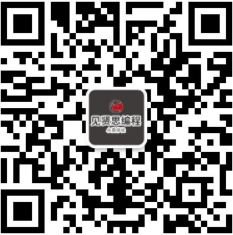
我不能保证我所说的都是对的,但我能保证每一篇都是用心去写的,我始终认同: “分享的越多,你的价值增值越大”,我们一同在分享中进步,在分享中成长,越努力越幸运。再分享一句话“十年前你是谁,一年前你是谁,甚至昨天你是谁,都不重要。重要的是,今天你是谁,以及明天你将成为谁。”
人生赢在转折处,改变从现在开始!
支持我的朋友们记得点波推荐哦,您的肯定就是我前进的动力。