vuejs3.0 从入门到精通——组件传值方法——兄弟组件之间的传值
兄弟组件之间的传值
一、第一种方案
A组件-->父组件-->B组件
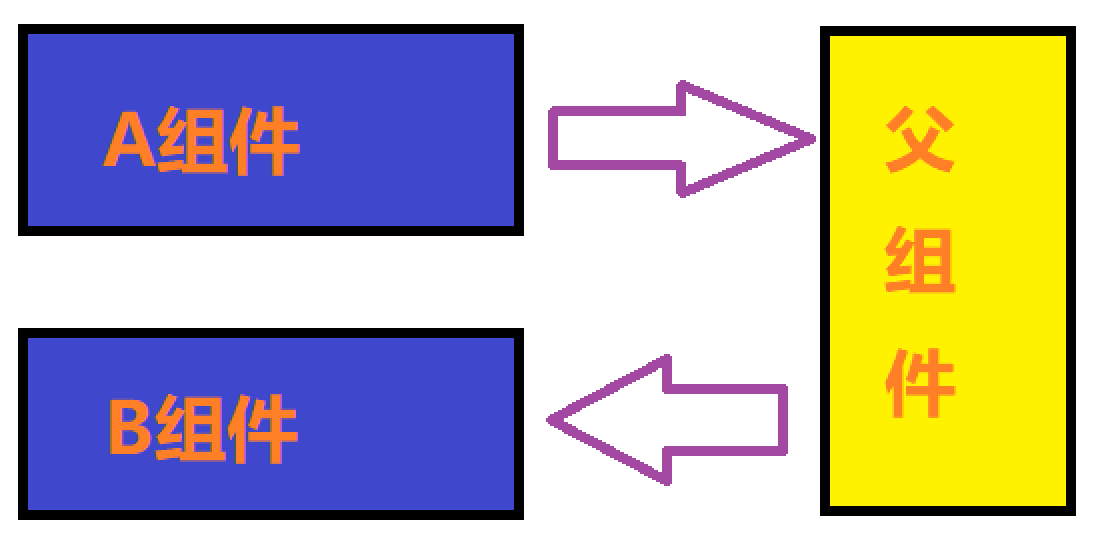
1.1、A组件
<template> <div> <h1>A组件</h1> <button @click="changeA">按钮</button> </div> </template> <script setup lang='ts'> import { ref } from 'vue' let str = ref('我是A组件的数据'); const emit = defineEmits(['fn']) const changeA = () => { emit('fn', str) } </script>
1.2、父组件
<template> <div> <A @fn='changeFn'></A> <B :str='str'></B> </div> </template> <script setup lang='ts'> import A from './A.vue' import B from './B.vue' import { ref } from 'vue' let str = ref('') const changeFn = (strVal: string) => { console.log(strVal) str.value = strVal } </script>
1.3、B组件
<template> <div> <h1>B组件</h1> {{ str }} </div> </template> <script setup lang='ts'> const props = defineProps({ str: { type: String, default: 'hello, 这是B组件数据' } }); console.log(props.str) </script>
二、第二种方案
2.1、组件安装
- npm install mitt -S
- mkdir -pv VITE-PROJECT/src/plugin/Bus.js
- echo "
import mitt from 'mitt';const emitter = mitt();export default emitter;
2.2、A组件
<!--子组件: A 组件-->
<template>
<div>
<h1>A组件</h1>
<button @click="changeA">按钮</button>
<hr>
</div>
</template>
<script setup lang='ts'>
import { ref } from 'vue'
import emitter from '@/plugin/Bus.ts'
let str = ref('我是A组件的数据');
const changeA = () => {
emitter.emit('Fn', str)
}
</script>
2.3、B组件
<!--子组件: B 组件--> <template> <div> <h1>B组件</h1> {{ s }} </div> </template> <script setup lang='ts'> import emitter from '@/plugin/Bus.ts' import { onBeforeMount, ref } from 'vue' let s = ref<unknown>(null); onBeforeMount(() => { emitter.on('Fn', (e: unknown) => { s.value = e; }) }) </script>