139. Word Break(动态规划)
Given a non-empty string s and a dictionary wordDict containing a list of non-empty words, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
Note:
- The same word in the dictionary may be reused multiple times in the segmentation.
- You may assume the dictionary does not contain duplicate words.
Example 1:
Input: s = "leetcode", wordDict = ["leet", "code"] Output: true Explanation: Return true because"leetcode"
can be segmented as"leet code"
.
Example 2:
Input: s = "applepenapple", wordDict = ["apple", "pen"] Output: true Explanation: Return true because"
applepenapple"
can be segmented as"
apple pen apple"
. Note that you are allowed to reuse a dictionary word.
Example 3:
Input: s = "catsandog", wordDict = ["cats", "dog", "sand", "and", "cat"] Output: false
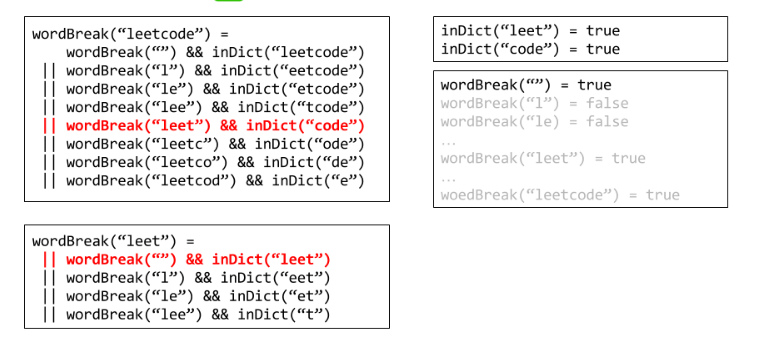
dp[i] 0-i这个字符串是否满足wordbreak
dp[0] = 0
dp[1] =dp[0] && s[0:1] in dict
dp[2] =dp[0] && s[0:1] in dict || dp[1] && s[1:2] in dict
dp[3] =dp[0] && s[0:3] in dict || dp[1] && s[1:3] in dict || dp[2] && s[2:3] in dict
dp[i] =dp[0] && s[0:i] in dict || ,,,,,,,||dp[i-1]&& s[i-1:i] in dict
Time complexity O(n^2)
Space complexity O(n)
语法注意:
s = "abcde"; 要得到”ce“
string word = s.substr(2,2);
1 class Solution { 2 public: 3 bool wordBreak(string s, vector<string>& wordDict) { 4 vector<bool> dp(s.size()+1,false); 5 unordered_set<string>dict(wordDict.begin(),wordDict.end()); 6 dp[0] = true; 7 for(int i = 1 ; i<=s.size();i++){ 8 for(int j = 0;j<i;j++){ 9 string word = s.substr(j,i-j); 10 if(dp[j]&&dict.find(word)!=dict.end()){ 11 cout<<word<<endl; 12 dp[i] = true; 13 break; 14 } 15 } 16 } 17 return dp[s.size()]; 18 } 19 };
参考:
http://zxi.mytechroad.com/blog/leetcode/leetcode-139-word-break/