X8023Z-DataGrid绑定DropdownList源码
该示例实现功能:
1:根据DropDownList动态更新DataGrid
2:利用DropDownList和TextBox作为模板列
3:编辑动态更新数据库
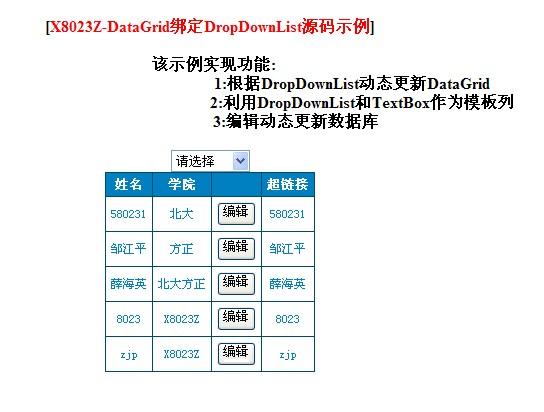
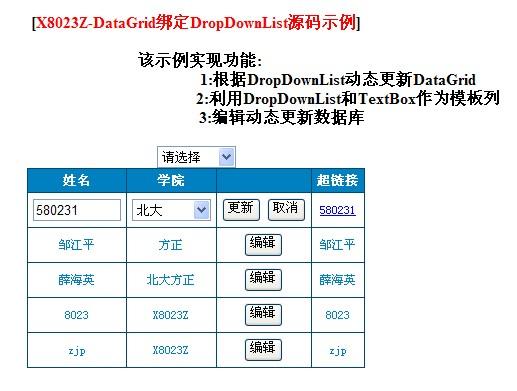
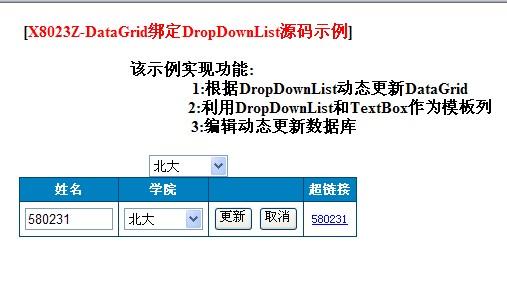
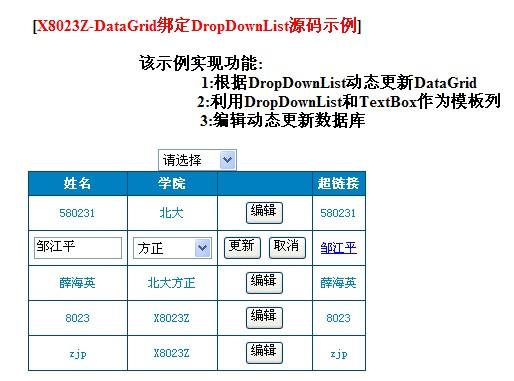
部分源码:
X8023Z_DataGrid
1
using System;
2
using System.Collections;
3
using System.ComponentModel;
4
using System.Configuration;
5
using System.Data;
6
using System.Drawing;
7
using System.Web;
8
using System.Web.SessionState;
9
using System.Web.UI;
10
using System.Web.UI.WebControls;
11
using System.Web.UI.HtmlControls;
12
using System.Data.SqlClient;
13
14
namespace X8023Z
15

{
16
/**//// <summary>
17
/// dgdr8023 的摘要说明。
18
/// </summary>
19
partial class dgdr8023 : System.Web.UI.Page
20
{
21
22
23
private void Page_Load(object sender, System.EventArgs e)
24
{
25
// 在此处放置用户代码以初始化页面
26
if (!IsPostBack)
27
{
28
SetBind();
29
SetBind2();
30
}
31
}
32
33
protected void SetBind()
34
{
35
36
SqlConnection conn = new SqlConnection(ConfigurationManager.AppSettings["conn"]);
37
SqlDataAdapter da = new SqlDataAdapter("select * from stu,dep where stu.studepid=dep.depid", conn);
38
DataSet ds = new DataSet();
39
da.Fill(ds, "table1");
40
this.DataGrid1.DataSource = ds.Tables["table1"];
41
this.DataGrid1.DataBind();
42
43
}
44
45
protected void SetBind2()
46
{
47
48
SqlConnection conn2 = new SqlConnection(ConfigurationManager.AppSettings["conn"]);
49
SqlDataAdapter da2 = new SqlDataAdapter("select * from dep", conn2);
50
DataSet ds2 = new DataSet();
51
da2.Fill(ds2, "table1");
52
this.DropDownList1.DataSource = ds2.Tables["table1"];
53
this.DropDownList1.DataTextField = "depname";
54
this.DropDownList1.DataValueField = "depid";
55
this.DropDownList1.DataBind();
56
this.DropDownList1.Items.Insert(0, new ListItem("请选择", ""));
57
58
}
59
60
protected void SetBind3()
61
{
62
string s = this.DropDownList1.SelectedValue;
63
SqlConnection conn = new SqlConnection(ConfigurationManager.AppSettings["conn"]);
64
SqlCommand comm = new SqlCommand();
65
comm.Connection = conn;
66
if (s != "")
67
{
68
comm.CommandText = "select * from stu,dep where stu.studepid=dep.depid and depid=@depid";
69
SqlParameter parm1 = new SqlParameter("@depid", SqlDbType.Int);
70
parm1.Value = s;
71
comm.Parameters.Add(parm1);
72
}
73
else
74
comm.CommandText = "select * from stu,dep where stu.studepid=dep.depid";
75
SqlDataAdapter da = new SqlDataAdapter();
76
da.SelectCommand = comm;
77
DataSet ds = new DataSet();
78
da.Fill(ds, "table1");
79
this.DataGrid1.DataSource = ds.Tables["table1"];
80
this.DataGrid1.DataBind();
81
82
}
83
Web 窗体设计器生成的代码#region Web 窗体设计器生成的代码
84
override protected void OnInit(EventArgs e)
85
{
86
//
87
// CODEGEN: 该调用是 ASP.NET Web 窗体设计器所必需的。
88
//
89
InitializeComponent();
90
base.OnInit(e);
91
}
92
93
/**//// <summary>
94
/// 设计器支持所需的方法 - 不要使用代码编辑器修改
95
/// 此方法的内容。
96
/// </summary>
97
private void InitializeComponent()
98
{
99
this.DataGrid1.ItemDataBound += new System.Web.UI.WebControls.DataGridItemEventHandler(this.DataGrid1_ItemDataBound);
100
this.DropDownList1.SelectedIndexChanged += new System.EventHandler(this.DropDownList1_SelectedIndexChanged);
101
this.Load += new System.EventHandler(this.Page_Load);
102
103
}
104
#endregion
105
106
private void DataGrid1_ItemDataBound(object sender, System.Web.UI.WebControls.DataGridItemEventArgs e)
107
{
108
109
SqlConnection conn = new SqlConnection(ConfigurationManager.AppSettings["conn"]);
110
SqlDataAdapter da = new SqlDataAdapter("select * from dep", conn);
111
DataSet ds = new DataSet();
112
da.Fill(ds, "table1");
113
if (e.Item.ItemType == ListItemType.EditItem)
114
{
115
DropDownList ddl = (DropDownList)e.Item.FindControl("dep");
116
ddl.DataSource = ds.Tables["table1"];
117
ddl.DataTextField = "depname";
118
ddl.DataValueField = "depid";
119
ddl.DataBind();
120
ddl.Items.FindByValue(Convert.ToString(DataBinder.Eval(e.Item.DataItem, "depid"))).Selected = true;//选择数据库内的作为默认
121
}
122
}
123
124
protected void edit(object sender, DataGridCommandEventArgs e)
125
{
126
this.DataGrid1.EditItemIndex = e.Item.ItemIndex;
127
if (this.DropDownList1.SelectedValue == "")
128
SetBind();
129
else
130
SetBind3();
131
}
132
133
protected void cancel(object sender, DataGridCommandEventArgs e)
134
{
135
this.DataGrid1.EditItemIndex = -1;
136
if (this.DropDownList1.SelectedValue == "")
137
SetBind();
138
else
139
SetBind3();
140
}
141
142
protected void update(object sender, DataGridCommandEventArgs e)
143
{
144
if (e.Item.ItemType == ListItemType.EditItem)//只有在编辑按下以后才能提交
145
{
146
SqlConnection conn = new SqlConnection(ConfigurationManager.AppSettings["conn"]);
147
SqlCommand comm = new SqlCommand("update stu set stuname=@name,studepid=@depid where stuid=@id", conn);
148
SqlParameter parm1 = new SqlParameter("@name", SqlDbType.NVarChar, 50);
149
parm1.Value = ((TextBox)e.Item.FindControl("name")).Text;
150
SqlParameter parm2 = new SqlParameter("@depid", SqlDbType.Int);
151
parm2.Value = ((DropDownList)e.Item.FindControl("dep")).SelectedValue;
152
SqlParameter parm3 = new SqlParameter("@id", SqlDbType.Int);
153
parm3.Value = this.DataGrid1.DataKeys[e.Item.ItemIndex];
154
comm.Parameters.Add(parm1);
155
comm.Parameters.Add(parm2);
156
comm.Parameters.Add(parm3);
157
conn.Open();
158
comm.ExecuteNonQuery();
159
conn.Close();
160
this.DataGrid1.EditItemIndex = -1;
161
if (this.DropDownList1.SelectedValue == "")
162
SetBind();
163
else
164
SetBind3();//如果选择过滤则使用SetBind3()
165
}
166
}
167
168
private void DropDownList1_SelectedIndexChanged(object sender, System.EventArgs e)
169
{
170
SetBind3();
171
}
172
}
173
}
如有学习者请与QQ群:60960278联系
1:根据DropDownList动态更新DataGrid
2:利用DropDownList和TextBox作为模板列
3:编辑动态更新数据库
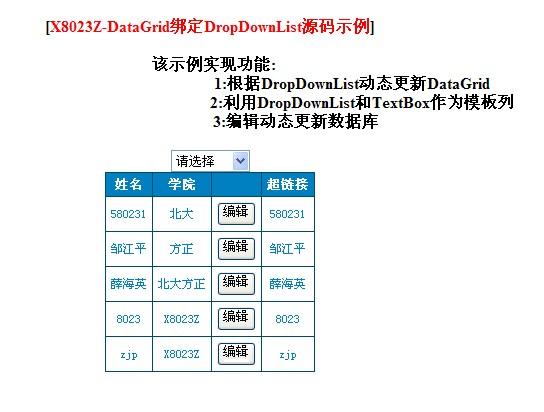
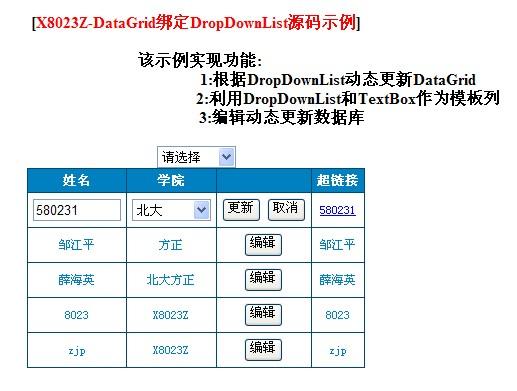
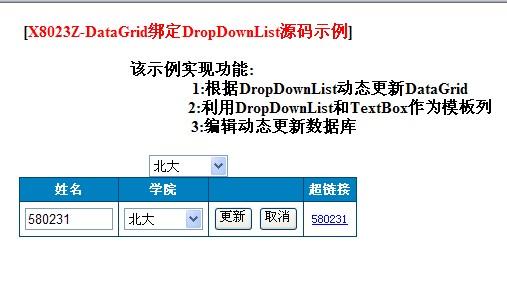
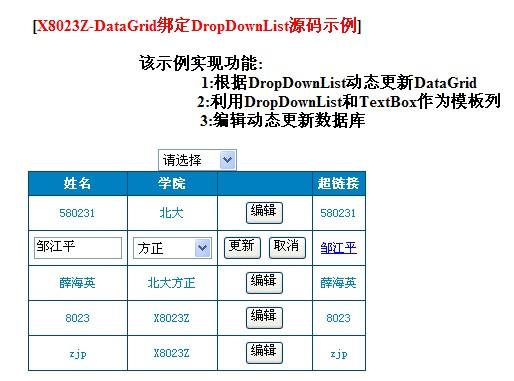
部分源码:


1

2

3

4

5

6

7

8

9

10

11

12

13

14

15



16


17

18

19

20



21

22

23

24



25

26

27



28

29

30

31

32

33

34



35

36

37

38

39

40

41

42

43

44

45

46



47

48

49

50

51

52

53

54

55

56

57

58

59

60

61



62

63

64

65

66

67



68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83


84

85



86

87

88

89

90

91

92

93


94

95

96

97

98



99

100

101

102

103

104

105

106

107



108

109

110

111

112

113

114



115

116

117

118

119

120

121

122

123

124

125



126

127

128

129

130

131

132

133

134



135

136

137

138

139

140

141

142

143



144

145



146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169



170

171

172

173

如有学习者请与QQ群:60960278联系