pymysql模块使用
一、写函数的原因
写这个函数的原因就是为了能够不每次在用Python用数据库的时候还要在写一遍 做个通用函数做保留,也给大家做个小小的分享,函数不是最好的,希望有更好的代码的朋友能提出 互相学习
二、函数代码
PS:代码是用Python3.6 写的
import pymysql class mysql (object): def __init__(self, dbconfig): """ 初始化连接信息 :param dbconfig: 连接信息的字典 """ self.host = dbconfig['host'] self.port = dbconfig['port'] self.user = dbconfig['user'] self.passwd = dbconfig['passwd'] self.db = dbconfig['db'] self.charset = dbconfig['charset'] self._conn = None self._connect() self._cursor = self._conn.cursor () def _connect(self): """ 连接数据库方法 :return: """ try: self._conn = pymysql.connect (host=self.host, port=self.port, user=self.user, passwd=self.passwd, db=self.db, charset=self.charset) except pymysql.Error as e: print(e) def query(self, sql): try: result = self._cursor.execute (sql) except pymysql.Error as e: print(e) result = False return result def select(self, table, column='*', condition=''): """ 查询数据库方法 :param table: 库里面的表 :param column: 列字段 :param condition: 条件语句 (where id=1) :return: """ condition = ' where ' + condition if condition else None if condition: sql = "select %s from %s %s" % (column, table, condition) # print(sql) else: sql = "select %s from %s" %(column, table) # print(sql) self.query (sql) return self._cursor.fetchall() def insert(self,table,tdict): """ 插入数据库方法,replace去重插入 insert不去重插入 :param table: 表名 :param tdict: 要插入的字典 :return: """ column = '' value = '' for key in tdict: column += ',' + key value += "','" + tdict[key] column = column[1:] value = value[2:] + "'" sql = "replace into %s(%s) values(%s)" %(table,column,value) # 去重 # sql = "insert into %s(%s) values(%s)" %(table,column,value) # 不去重 self._cursor.execute(sql) self._conn.commit() return self._cursor.lastrowid def update(self,table,tdict,condition=''): """ 更新数据库方法 :param table: 表名 :param tdict: 更新数据库数据字典 :param condition: 条件语句 (where id=1) :return: """ if not condition: print('must have id') exit() else: condition = 'where ' + condition value = '' for key in tdict: value += ",%s='%s'" %(key,tdict[key]) value = value[1:] sql = "update %s set %s %s" %(table,value,condition) # print(sql) self._cursor.execute(sql) self._conn.commit() return self.affected_num() def delete(self,table,condition=''): """ 删除方法 :param table: 表名 :param condition: 条件语句 (where id=1) :return: """ condition = ' where ' + condition if condition else None sql = "delete from %s %s" %(table,condition) # print(sql) self._cursor.execute(sql) self._conn.commit() return self.affected_num() def all(self,*args): """ 可以执行所有的SQL语句 相当于登录到数据库执行,就是返回结果没有做处理 后期会更新 :param args: SQL 语句(select * from ssh) :return: """ sql = input("请输入SQL语句>>:") sql = "%s" %sql self._cursor.execute (sql) self._conn.commit () return self._cursor.fetchall () def rollback(self): """ 数据库的事务 :return: """ self._conn.rollback() def affected_num(self): """ 受影响的条数 :return: """ return self._cursor.rowcount def int_to_ip_or_ip_to_int(self,method,num,ip=''): """ 主要是对数据库的IP和数值之间的转换 :param method: 转换方法两种(inet_ntoa,inet_aton) :param num: 数值 :param ip: IP地址 :return: """ if method == 'inet_ntoa': sql = "select %s(%s)" %(method,num) elif method == 'inet_aton': sql = "select %s(%s)" %(method,ip) self.query(sql) return self._cursor.fetchall() def __del__(self): """ 关闭连接 :return: """ try: self._cursor.close () self._conn.close () except: pass def close(self): """ 关闭连接 :return: """ self.__del__ () # 函数的使用 if __name__ == '__main__': dbconfig = { 'host': '192.168.163.129', # MySQL地址 'port': 3306, # 端口 'user': 'root', # 用户名 'passwd': '123456', # 密码 'db': 'com_hosts', # 数据库 'charset': 'utf8', # 字符集 } db = mysql (dbconfig) # 初始化函数 # 查询 print(db.select ('ssh')) # 更新 print(db.update('ssh', tdict, 'Id=2')) # 插入 print(db.insert('ssh',tdict)) # 删除 print(db.delete('ssh','Id=6')) # 执行SQL语句 while True: a = db.all() for i in a: print(i) db.close()
三、未实现功能(后续更新)
其实代码的结果最好都是元祖,没有对结果进行数据格式化显示,后期会更新,大家用的时候根据需求对数据进行一些处理就好了,其实在公司是想写个MySQL的管理平台的,以后写好了会更新,也希望大家一起学习,可以QQ我
作者:朱敬志
-------------------------------------------
个性签名:在逆境中要看到生活的美,在希望中别忘记不断奋斗
如果觉得这篇文章对你有小小的帮助的话,记得在右下角点个“推荐”哦,博主在此感谢!
万水千山总是情,打赏一分行不行,所以如果你心情还比较高兴,也是可以扫码打赏博主,哈哈哈(っ•̀ω•́)っ✎⁾⁾!
也可以关注我的微信公众号,不定时更新技术文章(kubernetes,Devops,Python)等
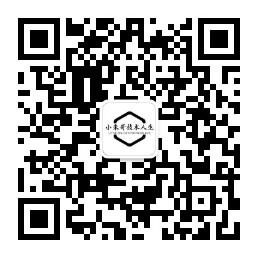