cURL php 爬虫 模拟 登陆 解析JSON
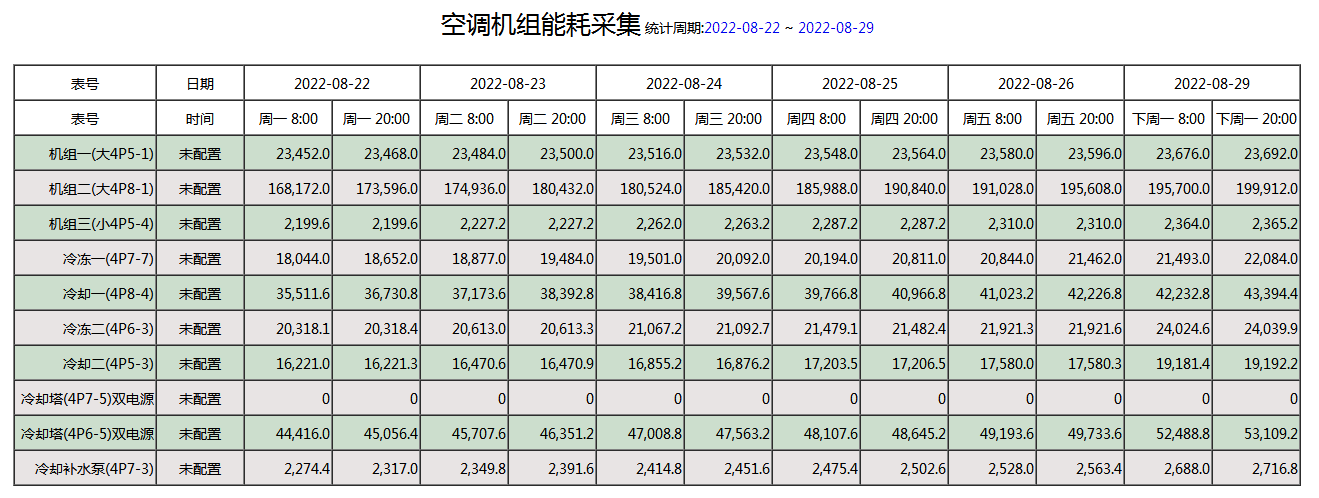

1 <?php 2 @header("content-type:text/html;charset=UTF-8"); 3 //登陆函数 4 if(isset($_REQUEST['tongjiTime'])){ 5 $chaxunTime = $_REQUEST ['tongjiTime']; 6 }else{ 7 $chaxunTime = date('Y-m-d', (time() - ((date('w') == 0 ? 7 : date('w')) - 1) * 24 * 3600)); 8 } 9 $urlQian = 'http://www.******.com:9999'; //账户信息地址 10 $user = '************'; //账户信息 11 $password = '***********'; //账户信息 12 $postUrl = $urlQian.'/home/system_login'; 13 function http_json_data($postUrl, $curlPost, $cookiesState) { 14 if (empty($postUrl) || empty($curlPost)) { 15 return false; 16 } 17 $cookie_file = dirname(__FILE__).'/cookie.txt'; 18 $ch = curl_init(); 19 $header = array(); 20 $header[] = 'content-type: application/x-www-form-urlencoded'; 21 $header[] = 'charset=UTF-8'; 22 curl_setopt($ch, CURLOPT_URL,$postUrl); 23 curl_setopt($ch, CURLOPT_HEADER, 0); 24 curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); 25 curl_setopt($ch, CURLOPT_POST, 1); 26 curl_setopt($ch, CURLOPT_POSTFIELDS, $curlPost); 27 if ($cookiesState==1){ 28 curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie_file); 29 }else{ 30 curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie_file); 31 } 32 curl_setopt($ch, CURLOPT_HTTPHEADER, $header); 33 curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); 34 curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false); 35 $data = curl_exec($ch); 36 curl_close($ch); 37 return $data; 38 } 39 function lidData($urlQian,$sid,$lid,$chaxunTime,$cookiesState=0,$k,$i) { 40 $postUrl=$urlQian.'/home/stoOriginal/list'; 41 $curlPost = 'sid='.$sid.'&lid='.$lid.'&time='.$chaxunTime; 42 $lidData = http_json_data($postUrl, $curlPost , $cookiesState=0); 43 $lidData= json_to_array($lidData); 44 //$fanhuiArray= array(); 45 //$fanhuiArray[][][$lid]['lid']=$lid; 46 $fanhuiArray['dclName'] = $lidData['rows']['8']['dclName']; 47 $fanhuiArray['chaxunTime'] = $chaxunTime; 48 $fanhuiArray['8']= $lidData['rows']['8']['stoOrignalValueZY']; 49 $fanhuiArray['20'] = $lidData['rows']['20']['stoOrignalValueZY']; 50 return $fanhuiArray; 51 } 52 function json_to_array($data) { 53 $data = json_decode($data,JSON_UNESCAPED_UNICODE); 54 return $data; 55 } 56 function array_to_json($data) { 57 $data = json_encode($data,JSON_UNESCAPED_UNICODE); 58 return $data; 59 } 60 $chaxuntimeArray = array( 61 $chaxunTime, 62 date("Y-m-d",strtotime("+1 day",strtotime($chaxunTime))), 63 date("Y-m-d",strtotime("+2 day",strtotime($chaxunTime))), 64 date("Y-m-d",strtotime("+3 day",strtotime($chaxunTime))), 65 date("Y-m-d",strtotime("+4 day",strtotime($chaxunTime))), 66 date("Y-m-d",strtotime("+7 day",strtotime($chaxunTime))) 67 ); 68 $weeks=array( 69 '周一', 70 '周二', 71 '周三', 72 '周四', 73 '周五', 74 '下周一' 75 ); 76 $bianhao=array( 77 '机组一(大4P5-1)'=>'4005', 78 '机组二(大4P8-1)'=>'4013', 79 '机组三(小4P5-4)'=>'4007', 80 '冷冻一(4P7-7)'=>'4012', 81 '冷却一(4P8-4)'=>'4014', 82 '冷冻二(4P6-3)'=>'4008', 83 '冷却二(4P5-3)'=>'4006', 84 '冷却塔(4P7-5)双电源'=>'4011', 85 '冷却塔(4P6-5)双电源'=>'4009', 86 '冷却补水泵(4P7-3)'=>'4010' 87 /* 4001,4002,4004,4010,4011,4012,4013,4014, 88 4003,4005,4006,4007,4008,4009,4032,4033,4034, 89 1002,1003 */ 90 ); 91 $curlPost='KEYDATA='.$user.',qt,'.md5($password).',qt,1234'; 92 $login = http_json_data($postUrl, $curlPost,$cookiesState=1); //模拟登陆 93 $login = json_to_array($login); 94 echo '<p align="center" style="font-size: 22px;">空调机组能耗采集<span style="font-size: 5px;"> 统计周期:<a style="text-decoration: none;" title="上一周" href="?tongjiTime='.date("Y-m-d",strtotime("-7 day",strtotime($chaxunTime))).'">'.$chaxunTime.'</a> ~ <a style="text-decoration: none;" title="下一周" href="?tongjiTime='.date("Y-m-d",strtotime("+7 day",strtotime($chaxunTime))).'">'.date("Y-m-d",strtotime("+7 day",strtotime($chaxunTime))).'</a></span></p>'; 95 if ($login['result']=='success'){ 96 echo '<table border="1" style="margin:auto;table-layout:fixed;font-size:12px;width: 1120px;height: 300px;" cellspacing="0">'; 97 echo '<tr style="text-align:center;height: 30px;"><td style="width: 120px;vertical-align:middle;">表号</td><td style="vertical-align:middle;">日期</td>'; 98 foreach($chaxuntimeArray as $key => $val ){ 99 echo '<td colspan="2" style="vertical-align:middle;">'.$val.'</td>'; 100 } 101 echo '</tr>'; 102 echo '<tr style="text-align:center;height:30px;"><td style="vertical-align:middle;">表号</td><td style="vertical-align:middle;">时间</td>'; 103 foreach($weeks as $key => $val ){ 104 echo '<td style="vertical-align:middle;">'.$val.' 8:00</td><td style="vertical-align:middle;">'.$val.' 20:00</td>'; 105 } 106 echo '</tr>'; 107 ///////////////解析表格开始/////////////////// 108 $ki=0; 109 foreach($bianhao as $k => $lid ){ 110 $ki++; 111 if ($ki%2==0){ 112 echo '<tr style="text-align:center;height:30px;background:#e8e4e4;">'; 113 }else{ 114 echo '<tr style="text-align:center;height:30px;background:#ccdecd;">'; 115 } 116 echo '<td style="vertical-align:middle;text-align:right;">'; 117 echo $k; 118 echo '</td>'; 119 echo '<td style="vertical-align:middle;">未配置</td>'; 120 foreach($chaxuntimeArray as $i => $xingqi ){ 121 $sid = '403'; 122 $data = lidData($urlQian,$sid,$lid,$xingqi,$cookiesState=0,$k,$i); 123 if ($data['8']==0){ 124 $fan[$k][$i]['8']=0; 125 }else{ 126 $fan[$k][$i]['8']=number_format($data['8'],1); 127 } 128 if ($data['20']==0){ 129 $fan[$k][$i]['20']=0; 130 }else{ 131 $fan[$k][$i]['20']=number_format($data['20'],1); 132 } 133 echo '<td style="text-align: right;">'.$fan[$k][$i]['8'].'</td><td style="text-align: right;">'.$fan[$k][$i]['20'].'</td>'; 134 } 135 echo '</tr>'; 136 } 137 ///////////////解析表格结束/////////////////// 138 echo '</tr></table>'; 139 echo '<p align="center">备注:该方案为全自动统计空调机组能耗,现在人工统计时间为8:30和20:30,<span style="color:red;">调整到8:00和20:00各一次</span>,经过测试准确无误后取消人工统计</p>'; 140 }else{ 141 echo "账户密码错误,请联系管理员!"; 142 } 143 ?>
知识点:
1.封装cURL函数, 传递cookie达到登陆或获取数据
if ($cookiesState==1){ curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie_file); }else{ curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie_file); }
2.多个foreach循环用法,
$ki=0; foreach($bianhao as $k => $lid ){ $ki++; if ($ki%2==0){ echo '<tr style="text-align:center;height:30px;background:#e8e4e4;">'; }else{ echo '<tr style="text-align:center;height:30px;background:#ccdecd;">'; } echo '<td style="vertical-align:middle;text-align:right;">'; echo $k; echo '</td>'; echo '<td style="vertical-align:middle;">未配置</td>'; foreach($chaxuntimeArray as $i => $xingqi ){ $sid = '403'; $data = lidData($urlQian,$sid,$lid,$xingqi,$cookiesState=0,$k,$i); if ($data['8']==0){ $fan[$k][$i]['8']=0; }else{ $fan[$k][$i]['8']=number_format($data['8'],1); } if ($data['20']==0){ $fan[$k][$i]['20']=0; }else{ $fan[$k][$i]['20']=number_format($data['20'],1); } echo '<td style="text-align: right;">'.$fan[$k][$i]['8'].'</td><td style="text-align: right;">'.$fan[$k][$i]['20'].'</td>'; } echo '</tr>'; }