鸿蒙NEXT开发案例:亲戚关系计算器
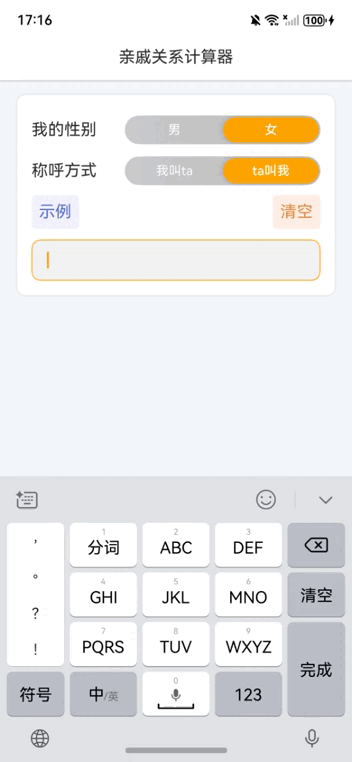
【引言】
在快节奏的现代生活中,人们往往因为忙碌而忽略了与亲戚间的互动,特别是在春节期间,面对众多的长辈和晚辈时,很多人会感到困惑,不知道该如何正确地称呼每一位亲戚。针对这一问题,我们开发了一款基于鸿蒙NEXT平台的“亲戚关系计算器”应用,旨在帮助用户快速、准确地识别和称呼他们的亲戚。
【环境准备】
• 操作系统:Windows 10
• 开发工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
• 目标设备:华为Mate60 Pro
• 开发语言:ArkTS
• 框架:ArkUI
• API版本:API 12
• 三方库:@nutpi/relationship(核心算法)
【应用背景】
中国社会有着深厚的家庭观念,亲属关系复杂多样。从血缘到姻亲,从直系到旁系,每一种关系都有其独特的称呼方式。然而,随着社会的发展,家庭成员之间的联系逐渐变得疏远,尤其是对于年轻人来说,准确地称呼每一位亲戚成了一项挑战。为了应对这一挑战,“亲戚关系计算器”应运而生。
【核心功能】
1. 关系输入:用户可以通过界面输入或选择具体的亲戚关系描述,例如“爸爸的哥哥的儿子”。
2. 性别及称呼选择:考虑到不同地区的习俗差异,应用允许用户选择自己的性别和希望使用的称呼方式,比如“哥哥”、“姐夫”等。
3. 关系计算:利用@nutpi/relationship库,根据用户提供的信息,精确计算出正确的亲戚称呼。
4. 示例与清空:提供示例按钮供用户测试应用功能,同时也设有清空按钮方便用户重新开始。
5. 个性化设置:支持多种方言和地方习惯的称呼方式,让应用更加贴近用户的实际需求。
【用户界面】
应用的用户界面简洁明了,主要由以下几个部分组成:
• 选择性别:通过分段按钮让用户选择自己的性别。
• 选择称呼方式:另一个分段按钮让用户选择希望的称呼方式。
• 输入关系描述:提供一个文本输入框,用户可以在此处输入具体的关系描述。
• 结果显示区:在用户提交信息后,这里会显示出正确的亲戚称呼。
• 操作按钮:包括示例按钮、清空按钮等,方便用户操作。
【完整代码】
导包
ohpm install @nutpi/relationship
代码
// 导入关系计算模块 import relationship from "@nutpi/relationship" // 导入分段按钮组件及配置类型 import { SegmentButton, SegmentButtonItemTuple, SegmentButtonOptions } from '@kit.ArkUI'; // 使用 @Entry 和 @Component 装饰器标记这是一个应用入口组件 @Entry @Component // 定义一个名为 RelationshipCalculator 的结构体,作为组件主体 struct RelationshipCalculator { // 用户输入的关系描述,默认值为“爸爸的堂弟” @State private userInputRelation: string = "爸爸的堂弟"; // 应用的主题颜色,设置为橙色 @State private themeColor: string | Color = Color.Orange; // 文字颜色 @State private textColor: string = "#2e2e2e"; // 边框颜色 @State private lineColor: string = "#d5d5d5"; // 基础内边距大小 @State private paddingBase: number = 30; // 性别选项数组 @State private genderOptions: object[] = [Object({ text: '男' }), Object({ text: '女' })]; // 称呼方式选项数组 @State private callMethodOptions: object[] = [Object({ text: '我叫ta' }), Object({ text: 'ta叫我' })]; // 性别选择按钮的配置 @State private genderButtonOptions: SegmentButtonOptions | undefined = undefined; // 称呼方式选择按钮的配置 @State private callMethodButtonOptions: SegmentButtonOptions | undefined = undefined; // 当前选中的性别索引 @State @Watch('updateSelections') selectedGenderIndex: number[] = [0]; // 当前选中的称呼方式索引 @State @Watch('updateSelections') selectedCallMethodIndex: number[] = [0]; // 用户输入的关系描述 @State @Watch('updateSelections') userInput: string = ""; // 计算结果显示 @State calculationResult: string = ""; // 输入框是否获得焦点 @State isInputFocused: boolean = false; // 当选择发生改变时,更新关系计算 updateSelections() { // 根据索引获取选中的性别(0为男,1为女) const gender = this.selectedGenderIndex[0] === 0 ? 1 : 0; // 判断是否需要反转称呼方向 const reverse = this.selectedCallMethodIndex[0] === 0 ? false : true; // 调用关系计算模块进行计算 const result: string[] = relationship({ text: this.userInput, reverse: reverse, sex: gender }) as string[]; // 如果有计算结果,则更新显示;否则显示默认提示 if (result && result.length > 0) { this.calculationResult = `${reverse ? '对方称呼我' : '我称呼对方'}:${result[0]}`; } else { this.calculationResult = this.userInput ? '当前信息未查到关系' : ''; } } // 组件即将显示时,初始化性别和称呼方式选择按钮的配置 aboutToAppear(): void { this.genderButtonOptions = SegmentButtonOptions.capsule({ buttons: this.genderOptions as SegmentButtonItemTuple, multiply: false, fontColor: Color.White, selectedFontColor: Color.White, selectedBackgroundColor: this.themeColor, backgroundColor: this.lineColor, backgroundBlurStyle: BlurStyle.BACKGROUND_THICK }); this.callMethodButtonOptions = SegmentButtonOptions.capsule({ buttons: this.callMethodOptions as SegmentButtonItemTuple, multiply: false, fontColor: Color.White, selectedFontColor: Color.White, selectedBackgroundColor: this.themeColor, backgroundColor: this.lineColor, backgroundBlurStyle: BlurStyle.BACKGROUND_THICK }); } // 构建组件界面 build() { // 创建主列布局 Column() { // 标题栏 Text('亲戚关系计算器') .fontColor(this.textColor) .fontSize(18) .width('100%') .height(50) .textAlign(TextAlign.Center) .backgroundColor(Color.White) .shadow({ radius: 2, color: this.lineColor, offsetX: 0, offsetY: 5 }); // 内部列布局 Column() { // 性别选择行 Row() { Text('我的性别').fontColor(this.textColor).fontSize(18); // 性别选择按钮 SegmentButton({ options: this.genderButtonOptions, selectedIndexes: this.selectedGenderIndex }).width('400lpx'); }.height(45).justifyContent(FlexAlign.SpaceBetween).width('100%'); // 称呼方式选择行 Row() { Text('称呼方式').fontColor(this.textColor).fontSize(18); // 称呼方式选择按钮 SegmentButton({ options: this.callMethodButtonOptions, selectedIndexes: this.selectedCallMethodIndex }).width('400lpx'); }.height(45).justifyContent(FlexAlign.SpaceBetween).width('100%'); // 示例与清空按钮行 Row() { // 示例按钮 Text('示例') .fontColor("#5871ce") .fontSize(18) .padding(`${this.paddingBase / 2}lpx`) .backgroundColor("#f2f1fd") .borderRadius(5) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .onClick(() => { this.userInput = this.userInputRelation; }); // 空白间隔 Blank(); // 清空按钮 Text('清空') .fontColor("#e48742") .fontSize(18) .padding(`${this.paddingBase / 2}lpx`) .clickEffect({ level: ClickEffectLevel.LIGHT, scale: 0.8 }) .backgroundColor("#ffefe6") .borderRadius(5) .onClick(() => { this.userInput = ""; }); }.height(45) .justifyContent(FlexAlign.SpaceBetween) .width('100%'); // 用户输入框 TextInput({ text: $$this.userInput, placeholder: !this.isInputFocused ? `请输入称呼。如:${this.userInputRelation}` : '' }) .placeholderColor(this.isInputFocused ? this.themeColor : Color.Gray) .fontColor(this.isInputFocused ? this.themeColor : this.textColor) .borderColor(this.isInputFocused ? this.themeColor : Color.Gray) .caretColor(this.themeColor) .borderWidth(1) .borderRadius(10) .onBlur(() => this.isInputFocused = false) .onFocus(() => this.isInputFocused = true) .height(45) .width('100%') .margin({ top: `${this.paddingBase / 2}lpx` }); } .alignItems(HorizontalAlign.Start) .width('650lpx') .padding(`${this.paddingBase}lpx`) .margin({ top: `${this.paddingBase}lpx` }) .borderRadius(10) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor, offsetX: 0, offsetY: 0 }); // 结果显示区 Column() { Row() { // 显示计算结果 Text(this.calculationResult).fontColor(this.textColor).fontSize(18); }.justifyContent(FlexAlign.SpaceBetween).width('100%'); } .visibility(this.calculationResult ? Visibility.Visible : Visibility.None) .alignItems(HorizontalAlign.Start) .width('650lpx') .padding(`${this.paddingBase}lpx`) .margin({ top: `${this.paddingBase}lpx` }) .borderRadius(10) .backgroundColor(Color.White) .shadow({ radius: 10, color: this.lineColor, offsetX: 0, offsetY: 0 }); } .height('100%') .width('100%') .backgroundColor("#f4f8fb"); } }