public class Array2D<T>{
public int Width { get; }
public int Height { get; }
public T[] Data { get; }
public Array2D(int width, int height)
{
Width = width;
Height = height;
Data = new T[width * height];
}
public T this[int x, int y]
{
get => Data[y * Width + x];
set => Data[y * Width + x] = value;
}
public T this[(int x, int y) index]
{
get => Data[index.y * Width + index.x];
set => Data[index.y * Width + index.x] = value;
}
}
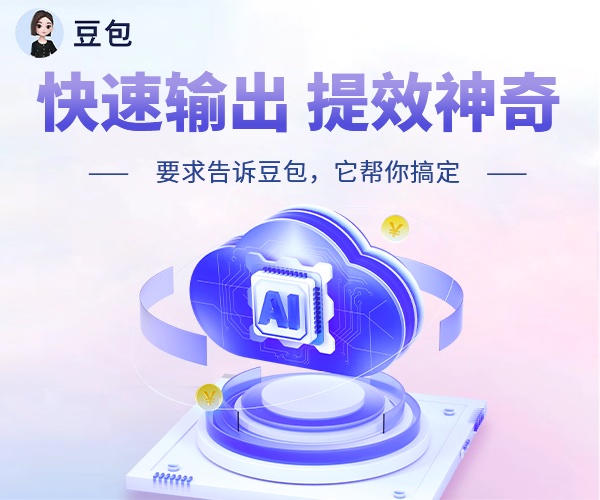