#ifndef MYLABEL_H
#define MYLABEL_H
#include <QWidget>
#include <QDebug>
#include <QMouseEvent>
#include <QLabel>
namespace Ui {
class MyLabel;
}
class MyLabel : public QLabel
{
Q_OBJECT
public:
explicit MyLabel(QWidget *parent = nullptr);
~MyLabel();
void mousePressEvent(QMouseEvent *event) override
{
qDebug() << "mousePressEvent";
}
void mouseReleaseEvent(QMouseEvent *event) override
{
qDebug() << "mouseReleaseEvent";
}
void mouseMoveEvent(QMouseEvent *event) override
{
qDebug() << "x" << event->x();
qDebug() << "y" << event->y();
qDebug() << "globalX" << event->globalX();
qDebug() << "globalY" << event->globalY();
}
private:
Ui::MyLabel *ui;
};
#endif // MYLABEL_H
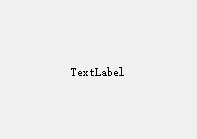
/* 鼠标按下、移动、释放 */
21:58:16: Starting ...
mousePressEvent
x 18
y 29
globalX 928
globalY 549
x 20
y 29
globalX 930
globalY 549
x 22
y 29
globalX 932
globalY 549
x 23
y 29
globalX 933
globalY 549
x 25
y 30
globalX 935
globalY 550
mouseReleaseEvent