引入依赖
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.0</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>${httpclient.version}</version>
<exclusions>
<exclusion>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</exclusion>
</exclusions>
</dependency>
准备工具类
package com.bm.test.utils;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.springframework.util.ObjectUtils;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.Map;
public class HttpClientUtil {
public static String doGet(String url, Map<String, String> params) {
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 响应结果字符串
String resultStr = "";
// 响应对象CloseableHttpResponse
CloseableHttpResponse response = null;
try {
// 创建URI
URIBuilder uriBuilder = new URIBuilder(url);
if (params != null) {
for (String key : params.keySet()) {
uriBuilder.addParameter(key, params.get(key));
}
}
URI uri = uriBuilder.build();
// 创建HttpGet请求
HttpGet httpGet = new HttpGet(uri);
// 执行请求
response = httpClient.execute(httpGet);
// 判断返回状态
if (response.getStatusLine().getStatusCode() == 200) {
resultStr = EntityUtils.toString(response.getEntity(), "UTF-8");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (response != null) {
response.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
return resultStr;
}
public static String doGet(String url) {
return doGet(url, null);
}
public static String doPost(String url, Map<String, String> params) {
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 响应结果字符串
String resultStr = "";
// 响应对象CloseableHttpResponse
CloseableHttpResponse response = null;
try {
// 创建HttpPost对象
HttpPost httpPost = new HttpPost(url);
ArrayList<NameValuePair> paramList = new ArrayList<>();
if (params != null) {
for (String key : params.keySet()) {
paramList.add(new BasicNameValuePair(key, params.get(key)));
}
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList);
httpPost.setEntity(entity);
}
// 执行请求
response = httpClient.execute(httpPost);
resultStr = EntityUtils.toString(response.getEntity(), "UTF-8");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (!ObjectUtils.isEmpty(response)) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return resultStr;
}
public static String doPost(String url) {
return doGet(url, null);
}
public static String doPostJson(String url, String json) {
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 响应结果字符串
String resultStr = "";
// 响应对象CloseableHttpResponse
CloseableHttpResponse response = null;
try {
// 创建HttpPost请求
HttpPost httpPost = new HttpPost(url);
// 创建请求内容
StringEntity entity = new StringEntity(json, ContentType.APPLICATION_JSON);
httpPost.setEntity(entity);
// 执行请求
response = httpClient.execute(httpPost);
resultStr = EntityUtils.toString(response.getEntity(), "UTF-8");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return resultStr;
}
}
调用方法
String state = "fail";
Integer isOpened = 1;
Map<String, String> params = new HashMap<>();
params.put("eid", "04F06D14-F1C9-4A46-BDFF-F25CA6D3A176");
String result = HttpClientUtil.doPost("url", params);
if (!StringUtils.isEmpty(result)) {
JSONObject resultJSON = JSONObject.parseObject(result);
state = (String) resultJSON.get("status");
isOpened = (Integer) resultJSON.get("is_opened");
} else {
state = "ok";
isOpened = 0;
}
if ("ok".equals(state) && 0 == isOpened) {
return R.error();
}
关注我的公众号SpaceObj 领取idea系列激活码
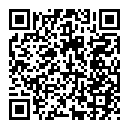