HDU 1016 Prime Ring Problem 题解
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
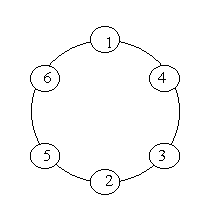
Note: the number of first circle should always be 1.
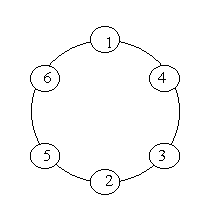
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
本题就是考递归搜索的能力。
数据不大。其它Prime, map等的优化都没多大作用的。
记得记录好数据,就不会有问题了。
只是HDU的推断系统的确垃圾,其它OJ都不会在意末尾多一个空格或者回车的问题的,HDU就一个空格一个回车都一定要依照她的格式。否则就presentation error.
#include <stdio.h> const int MAX_N = 20; int num; int cycle[MAX_N]; bool vis[MAX_N]; bool isPrime(int n) { for (int i = 2; i*i <= n; i++) if (n % i == 0) return false; return true; } bool isLegal(int val, int i) { int left = i-1; if (!isPrime(val+cycle[left])) return false; if (i+1 == num && !isPrime(val+cycle[0])) return false; return true; } void printNums(int i = 1) { if (i == num) { for (int j = 0; j+1 < num; j++) { printf("%d ", cycle[j]); } printf("%d\n", cycle[i-1]); return ; } for (int v = 2; v <= num; v++) { if (vis[v]) continue; if (isLegal(v, i)) { cycle[i] = v; vis[v] = true; printNums(i+1); vis[v] = false; } } } int main() { int t = 0; cycle[0] = 1; while (scanf("%d", &num) != EOF) { printf("Case %d:\n", ++t); for (int i = 2; i <= num; i++) vis[i] = false; printNums(); putchar('\n'); } return 0; }