1 <?php 2 #二叉树的非递归遍历 3 class Node { 4 public $data; 5 public $left; 6 public $right; 7 } 8 9 #前序遍历,和深度遍历一样 10 function preorder($root) { 11 $stack = array(); 12 array_push($stack, $root); 13 while (!empty($stack)) { 14 $cnode = array_pop($stack); 15 echo $cnode->data . " "; 16 if ($cnode->right != null) array_push($stack, $cnode->right); 17 if ($cnode->left != null) array_push($stack, $cnode->left); 18 } 19 } 20 21 #中序遍历 22 function inorder($root) { 23 $stack = array(); 24 $cnode = $root; 25 while (!empty($stack) || $cnode != null) { 26 while ($cnode != null) { 27 array_push($stack, $cnode); 28 $cnode = $cnode->left; 29 } 30 31 $cnode = array_pop($stack); 32 echo $cnode->data . " "; 33 34 $cnode = $cnode->right; 35 } 36 } 37 38 #后序遍历 39 #使用双栈实现 40 function postorder($root) { 41 $pushstack = array(); 42 $visitstack = array(); 43 array_push($pushstack, $root); 44 45 while (!empty($pushstack)) { 46 $cnode = array_pop($pushstack); 47 array_push($visitstack, $cnode); 48 if ($cnode->left != null) array_push($pushstack, $cnode->left); 49 if ($cnode->right != null) array_push($pushstack, $cnode->right); 50 } 51 52 while (!empty($visitstack)) { 53 $cnode = array_pop($visitstack); 54 echo $cnode->data . " "; 55 } 56 } 57 58 $root = new Node(); 59 $n1 = new Node(); 60 $n11 = new Node(); 61 $n12 = new Node(); 62 $n2 = new Node(); 63 $n21 = new Node(); 64 $n22 = new Node(); 65 $root->data = 0; 66 $n1->data = 1; 67 $n11->data = 11; 68 $n12->data = 12; 69 $n2->data = 2; 70 $n21->data = 21; 71 $n22->data = 22; 72 $root->left = $n1; 73 $root->right = $n2; 74 $n1->left = $n11; 75 $n1->right = $n12; 76 $n2->left = $n21; 77 $n2->right = $n22; 78 79 preorder($root); 80 echo "<br>"; 81 inorder($root); 82 echo "<br>"; 83 postorder($root); 84 ?>
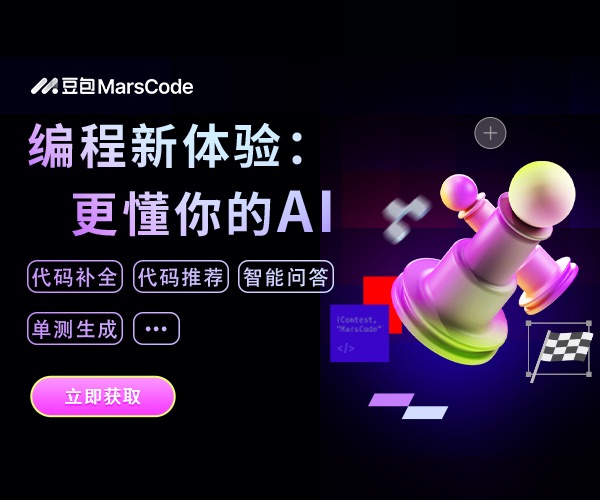