Abp.VNext 用.NET Core Console 项目介绍Abp (一)
首先需要 Nuget 上下载 Volo.Abp.Core Volo.Abp.Autofac
项目结构
AbpConsole 项目 Program 代码 引用了 Volo.Abp.Autofac
1 using AbpConsole; 2 using Microsoft.Extensions.DependencyInjection; 3 using Microsoft.Extensions.Hosting; 4 using Volo.Abp.Modularity.PlugIns; 5 6 static IHostBuilder CreateHostBuilder(string[] args) => 7 Host.CreateDefaultBuilder(args) 8 .ConfigureAppConfiguration((context, config) => 9 { 10 //setup your additional configuration sources 11 }) 12 .UseAutofac() 13 .ConfigureServices((hostContext, services) => 14 { 15 services.AddApplication<ConsoleModule>(options => 16 { 17 // 1、加载插件 18 options.PlugInSources.AddFolder(@"D:\学习\Plugln"); 19 }); 20 }); 21 CreateHostBuilder(args).RunConsoleAsync().Wait();
ConsoleHostedService 代码
1 using AbpConsoleAppCommon; 2 using AbpConsoleInteface; 3 using Microsoft.Extensions.Hosting; 4 using System; 5 using System.Collections.Generic; 6 using System.Linq; 7 using System.Text; 8 using System.Threading.Tasks; 9 10 namespace AbpConsole 11 { 12 public class ConsoleHostedService : IHostedService 13 { 14 15 //没有 传统 构造函数注入 16 // private readonly HelloWorldService _helloWorldService; 17 //public ConsoleHostedService(HelloWorldService helloWorldService) 18 //{ 19 // this._helloWorldService = helloWorldService; 20 //} 21 22 23 /// <summary> 24 /// 利用 abp 的autofac 进行属性依赖注入 25 /// </summary> 26 public HelloWorldService _helloWorldService { get; set; } 27 28 public CommonService CommonService { get; set; } 29 //这是插件 30 public IPluginService Plugln { get; set; } 31 public Task StartAsync(CancellationToken cancellationToken) 32 { 33 this._helloWorldService.Helloworld(); 34 this.CommonService.Show(); 35 Plugln.Plugin(); 36 return Task.CompletedTask; 37 } 38 39 public Task StopAsync(CancellationToken cancellationToken) 40 { 41 throw new NotImplementedException(); 42 } 43 } 44 }
ConsoleModule 代码
1 using AbpConsoleAppCommon; 2 using Microsoft.Extensions.DependencyInjection; 3 using System; 4 using System.Collections.Generic; 5 using System.Linq; 6 using System.Text; 7 using System.Threading.Tasks; 8 using Volo.Abp.Autofac; 9 using Volo.Abp.DependencyInjection; 10 using Volo.Abp.Modularity; 11 12 namespace AbpConsole 13 { 14 [DependsOn(typeof(AbpAutofacModule),typeof(CommonModule))] 15 public class ConsoleModule : AbpModule 16 { 17 public override void ConfigureServices(ServiceConfigurationContext context) 18 { 19 // 1、IOC注册自定义类 20 System.Console.WriteLine("加载ConsoleModule模块"); 21 //context.Services.AddSingleton<HelloWorldService>(); 22 context.Services.AddHostedService<ConsoleHostedService>(); 23 } 24 } 25 }
HelloWorldService 代码
1 using Microsoft.Extensions.DependencyInjection; 2 using System; 3 using System.Collections.Generic; 4 using System.Linq; 5 using System.Text; 6 using System.Threading.Tasks; 7 using Volo.Abp.DependencyInjection; 8 9 namespace AbpConsole 10 { 11 [Dependency(ServiceLifetime.Transient)] 12 public class HelloWorldService//: ITransientDependency 13 { 14 public void Helloworld() 15 { 16 Console.WriteLine("你好世界"); 17 } 18 } 19 }
这里主要 是特性
[DependsOn(typeof(AbpAutofacModule),typeof(CommonModule))] 对Module的标注 这里面是 是typeof 属性加载所以 Autofac需要在第一位置 要不然依赖
注入会有问题
[Dependency(ServiceLifetime.Transient)] 对 HelloWorldService 标注 有对生命周期 的标注
下面代码主要是 Abp 引用其他项目
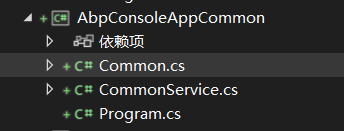
1 using Microsoft.Extensions.DependencyInjection; 2 using System; 3 using System.Collections.Generic; 4 using System.Linq; 5 using System.Text; 6 using System.Threading.Tasks; 7 using Volo.Abp.Modularity; 8 9 namespace AbpConsoleAppCommon 10 { 11 public class CommonModule:AbpModule 12 { 13 public override void ConfigureServices(ServiceConfigurationContext context) 14 { 15 // base.ConfigureServices(context); 16 // context.Services.AddHostedService<CommonService>(); 17 System.Console.WriteLine("加载CommonModule模块"); 18 } 19 } 20 }
1 using Microsoft.Extensions.DependencyInjection; 2 using System; 3 using System.Collections.Generic; 4 using System.Linq; 5 using System.Text; 6 using System.Threading.Tasks; 7 using Volo.Abp.DependencyInjection; 8 9 namespace AbpConsoleAppCommon 10 { 11 12 [Dependency(ServiceLifetime.Scoped)] 13 public class CommonService 14 { 15 public void Show() 16 { 17 Console.WriteLine("我是common"); 18 } 19 } 20 }
引用Common项目
设置依赖设置
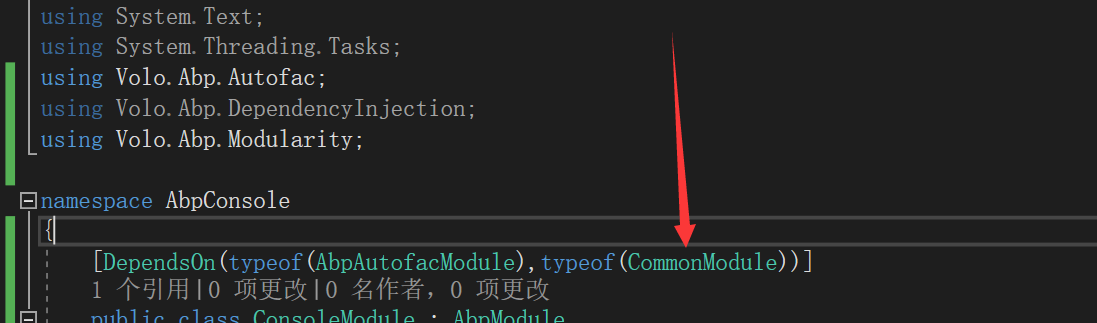
使用插件
上层不能直接依赖插件 ,如果直接依赖插件就违反开闭原则,需要依赖接口
重点说明 接口实现 类名称是有约定的 ,一般去掉 I 就是接口实现类 要不然加载插件会出现注入报错的情况
PluginService : IPluginService
1 using AbpConsoleInteface; 2 using Microsoft.Extensions.DependencyInjection; 3 using System; 4 using System.Collections.Generic; 5 using System.Linq; 6 using System.Text; 7 using System.Threading.Tasks; 8 using Volo.Abp.DependencyInjection; 9 namespace AbpConsole.Plugln 10 { 11 [Dependency(ServiceLifetime.Transient)] 12 public class PluginService : IPluginService 13 { 14 public void Plugin() 15 { 16 Console.WriteLine("我是插件"); 17 } 18 } 19 }
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Text; 5 using System.Threading.Tasks; 6 using Volo.Abp.Modularity; 7 8 namespace AbpConsole.Plugln 9 { 10 /// <summary> 11 /// 插件模块 12 /// </summary> 13 public class Plugin : AbpModule 14 { 15 public override void ConfigureServices(ServiceConfigurationContext context) 16 { 17 System.Console.WriteLine("加载插件模块..........."); 18 } 19 } 20 }
然后编译,将编译后的DLL文件放置到文件夹里,然后引用
.ConfigureServices((hostContext, services) =>
{
services.AddApplication<ConsoleModule>(options =>
{
// 1、加载插件
options.PlugInSources.AddFolder(@"D:\学习\Plugln");
});
});
1 // See https://aka.ms/new-console-template for more information 2 3 4 using AbpConsole; 5 using Microsoft.Extensions.DependencyInjection; 6 using Microsoft.Extensions.Hosting; 7 using Volo.Abp.Modularity.PlugIns; 8 9 static IHostBuilder CreateHostBuilder(string[] args) => 10 Host.CreateDefaultBuilder(args) 11 .ConfigureAppConfiguration((context, config) => 12 { 13 //setup your additional configuration sources 14 }) 15 .UseAutofac() 16 .ConfigureServices((hostContext, services) => 17 { 18 services.AddApplication<ConsoleModule>(options => 19 { 20 // 1、加载插件 21 options.PlugInSources.AddFolder(@"D:\学习\Plugln"); 22 }); 23 }); 24 CreateHostBuilder(args).RunConsoleAsync().Wait(); 25 26 Console.WriteLine("Hello, World!");
掉用 直接写接口依赖注入了
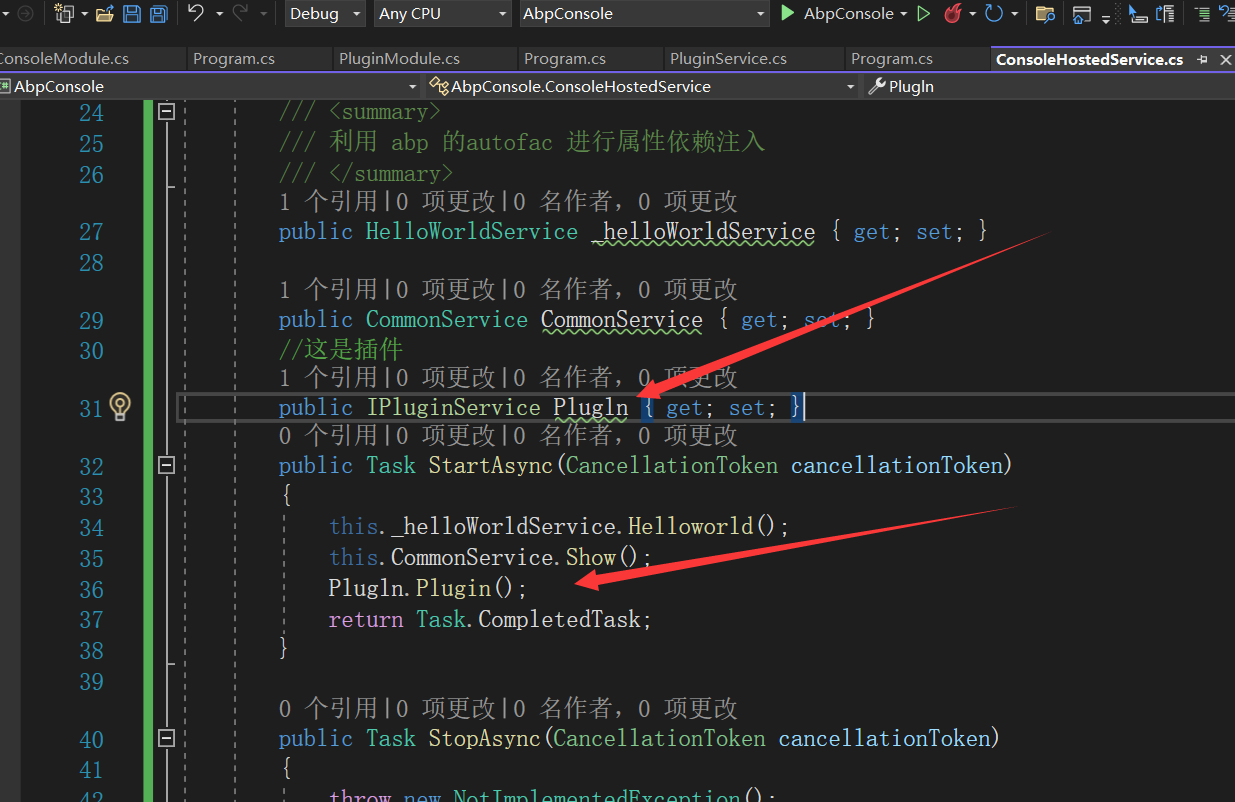