Load Caffe framework models
Introduction
In this tutorial you will learn how to use opencv_dnn module for image classification by using GoogLeNet trained network from Caffe model zoo.
We will demonstrate results of this example on the following picture.
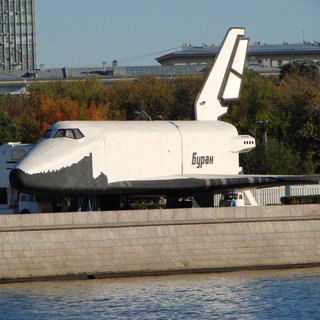
Source Code
We will be using snippets from the example application, that can be downloaded here.
Explanation
-
Firstly, download GoogLeNet model files: bvlc_googlenet.prototxt and bvlc_googlenet.caffemodel
Also you need file with names of ILSVRC2012 classes: synset_words.txt.
Put these files into working dir of this program example.
- Create the importer of Caffe models
Ptr<dnn::Importer> importer;try //Try to import Caffe GoogleNet model{importer = dnn::createCaffeImporter(modelTxt, modelBin);}catch (const cv::Exception &err) //Importer can throw errors, we will catch them{std::cerr << err.msg << std::endl;}
- Create the network and initialize its by using the created importer
dnn::Net net;importer->populateNet(net);importer.release(); //We don't need importer anymore
-
Read input image and convert to the blob, acceptable by GoogleNet
Mat img = imread(imageFile);if (img.empty()){std::cerr << "Can't read image from the file: " << imageFile << std::endl;exit(-1);}dnn::Blob inputBlob = dnn::Blob(img); //Convert Mat to dnn::Blob image batchFirstly, we resize the image and change its channel sequence order.
Now image is actually a 3-dimensional array with 224x224x3 shape.
Next, we convert the image to 4-dimensional blob (so-called batch) with 1x2x224x224 shape by using special cv::dnn::Blob constructor.
-
Pass the blob to the network
net.setBlob(".data", inputBlob); //set the network inputIn bvlc_googlenet.prototxt the network input blob named as "data", therefore this blob labeled as ".data" in opencv_dnn API.
Other blobs labeled as "name_of_layer.name_of_layer_output".
- Make forward pass
net.forward(); //compute output
- Determine the best class
dnn::Blob prob = net.getBlob("prob"); //gather output of "prob" layerint classId;double classProb;getMaxClass(prob, &classId, &classProb);//find the best class
prob
blob. And find the index of element with maximal value in this one. This index correspond to the class of the image. - Print results
std::vector<String> classNames = readClassNames();std::cout << "Best class: #" << classId << " '" << classNames.at(classId) << "'" << std::endl;std::cout << "Probability: " << classProb * 100 << "%" << std::endl;
Best class: #812 'space shuttle'
Probability: 99.6378%