5. Django Templates 模板语言
5.1 Templstes 变量传值:
项目整体结构
url.py 中的代码:

1 from django.contrib import admin 2 from django.urls import path 3 from app01 import views as app01_views 4 5 urlpatterns = [ 6 path('admin/', admin.site.urls), 7 path('', app01_views.index), 8 path('index02/', app01_views.index02), 9 path('index03/', app01_views.index03), 10 path('index04/', app01_views.index04), 11 path('index05/', app01_views.index05), 12 ]
view.py 代码:

1 from django.shortcuts import render 2 from django.http import HttpResponse 3 4 # Create your views here. 5 6 def index(request): 7 # 获取登录的用户名 8 username = request.GET.get('username') 9 if username: 10 # 如果想把值传到Templates中,必须传字典类型 11 # {'user': username} --user: 在模板中通过这个名称访问,username: 具体传过去的值 12 context = {'user': username} 13 return render(request,'index.html',context=context) 14 else: 15 return HttpResponse("没有登录的用户!!!") 16 17 def index02(request): 18 # 传递多个值: 学号,姓名,性别,出生日期 19 sno = "950001" 20 name = "张三" 21 gender = "男" 22 birthday = "1990-07-02" 23 # 把多个值拼接成字典类型 24 context = {'sno': sno, 'name': name, 'gender': gender, 'birthday': birthday} 25 26 # 加载HTML时附带数据 27 return render(request, 'index02.html', context=context) 28 29 def index03(request): 30 # 传递list 集合 31 alice = ['95001', 'alice', '女', '1998-10-10'] 32 return render(request,'index03.html',context={'student': alice}) 33 34 def index04(request): 35 # 传递字典类型 36 alice = {'sno':'95001', 'name':'alice', 'gender':'女', 'birthday':'1998-10-10'} 37 return render(request, 'index04.html', context={'student': alice}) 38 39 # 定义一个类 40 class Student(object): 41 def __init__(self, sno, name, gender, birthday): 42 self.sno = sno 43 self.name = name 44 self.gender = gender 45 self.birthday = birthday 46 def index05(request): 47 # 传递一个对象 48 alice = Student('95008', '张三', '男', '1998-09-09') 49 return render(request, 'index05.html', context={'student': alice})
访问页面:
单个变量:

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style> 7 body{ 8 margin: 0px; 9 padding: 0px; 10 } 11 #title{ 12 width: 100%; 13 height: 100px; 14 background-color: yellowgreen; 15 font-size: 30px; 16 line-height: 100px; 17 } 18 #div01{ 19 width: 1200px; 20 height: 100px; 21 background-color: yellow; 22 margin: auto; 23 font-size: 25px; 24 line-height: 100px; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">模板语音变量传值</div> 30 <div id="div01">当前用户:{{ user }}</div> 31 </body> 32 </html>
访问页面:
传递多个值:HTML中引用

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style> 7 body{ 8 margin: 0px; 9 padding: 0px; 10 } 11 #title{ 12 width: 100%; 13 height: 100px; 14 background-color: yellowgreen; 15 font-size: 30px; 16 line-height: 100px; 17 } 18 #div01{ 19 width: 1200px; 20 height: 100px; 21 background-color: yellow; 22 margin: auto; 23 font-size: 25px; 24 line-height: 100px; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">模板语音变量传值</div> 30 <div id="div01">传递多个值: 31 学号:{{ sno }} 32 姓名:{{ name }} 33 性别:{{ gender }} 34 出生日期:{{ birthday }} 35 </div> 36 </body> 37 </html>
访问页面:
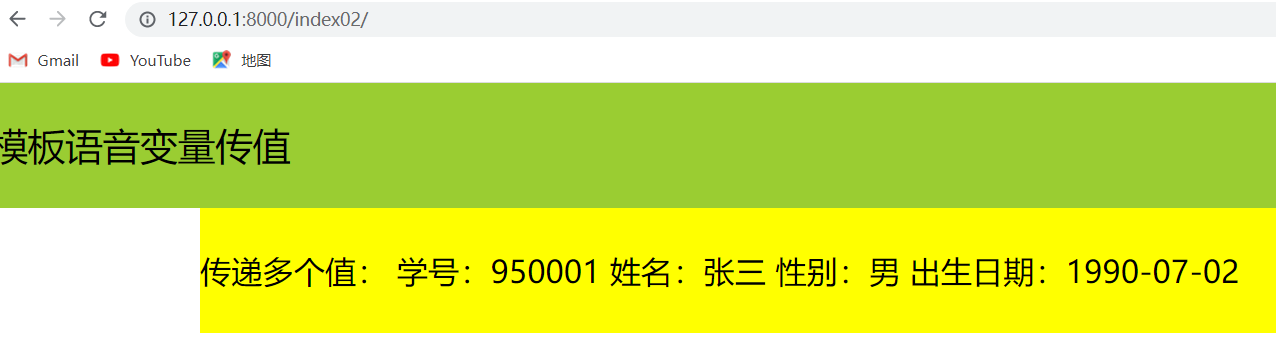
传递List 集合:HTML 中引用

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style> 7 body{ 8 margin: 0px; 9 padding: 0px; 10 } 11 #title{ 12 width: 100%; 13 height: 100px; 14 background-color: yellowgreen; 15 font-size: 30px; 16 line-height: 100px; 17 } 18 #div01{ 19 width: 1200px; 20 height: 100px; 21 background-color: yellow; 22 margin: auto; 23 font-size: 25px; 24 line-height: 100px; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">模板语音变量传值</div> 30 <div id="div01">传递多个值----List集合: 31 学号:{{ student.0 }} 32 姓名:{{ student.1 }} 33 性别:{{ student.2 }} 34 出生日期:{{ student.3 }} 35 </div> 36 </body> 37 </html>
访问页面:
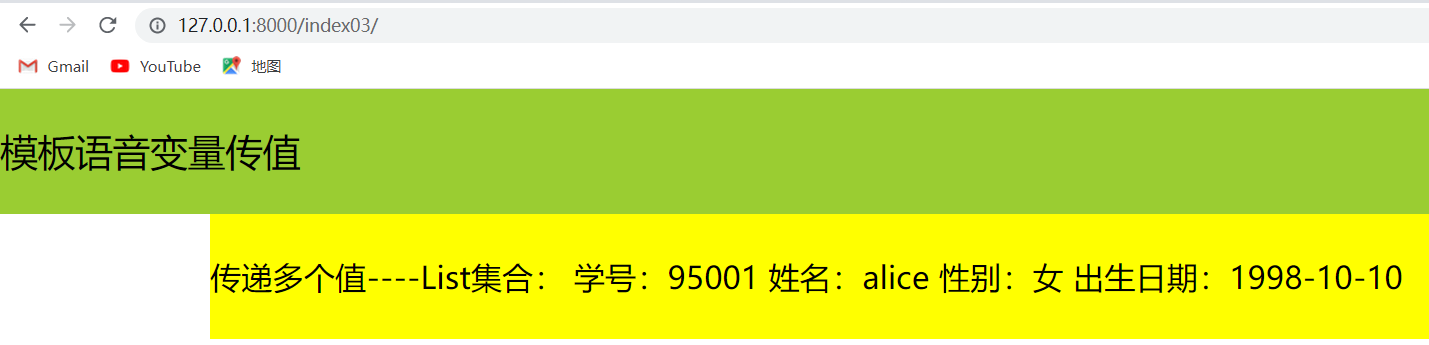
传递字典: HTML 中引用

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style> 7 body{ 8 margin: 0px; 9 padding: 0px; 10 } 11 #title{ 12 width: 100%; 13 height: 100px; 14 background-color: yellowgreen; 15 font-size: 30px; 16 line-height: 100px; 17 } 18 #div01{ 19 width: 1200px; 20 height: 100px; 21 background-color: yellow; 22 margin: auto; 23 font-size: 25px; 24 line-height: 100px; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">模板语音变量传值</div> 30 <div id="div01">传递多个值----Dict集合: 31 学号:{{ student.sno }} 32 姓名:{{ student.name }} 33 性别:{{ student.gender }} 34 出生日期:{{ student.birthday }} 35 </div> 36 </body> 37 </html>
访问页面:
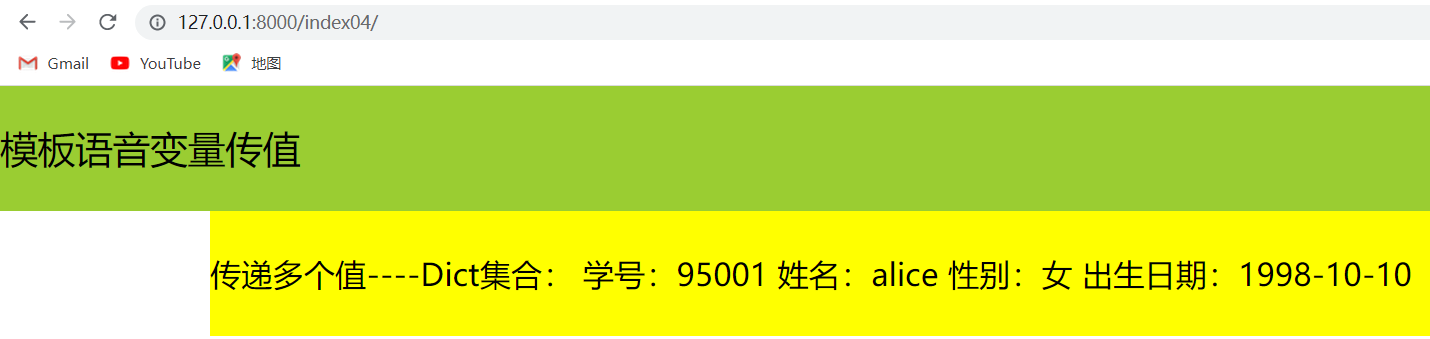
传递对象:HTML中引用

1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style> 7 body{ 8 margin: 0px; 9 padding: 0px; 10 } 11 #title{ 12 width: 100%; 13 height: 100px; 14 background-color: yellowgreen; 15 font-size: 30px; 16 line-height: 100px; 17 } 18 #div01{ 19 width: 1200px; 20 height: 100px; 21 background-color: yellow; 22 margin: auto; 23 font-size: 25px; 24 line-height: 100px; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">模板语音变量传值</div> 30 <div id="div01">传递多个值----对象: 31 学号:{{ student.sno }} 32 姓名:{{ student.name }} 33 性别:{{ student.gender }} 34 出生日期:{{ student.birthday }} 35 </div> 36 </body> 37 </html>
访问页面:
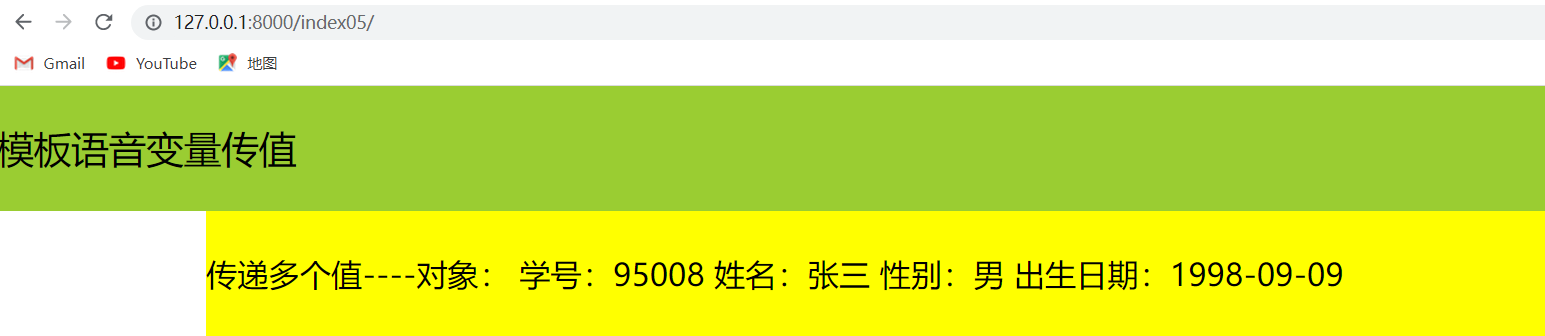
5.2 模板中的if 标签

1 {% load static %} 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <title>youku首页</title> 7 <link type="text/css" rel="stylesheet" href="{% static 'css/basic.css' %}"> 8 </head> 9 <body> 10 <div id="title"> 11 <div> 12 <div id="left">优酷首页</div> 13 <div id="right"> 14 {% if type == '1' %} 15 欢迎您!{{ user }}[普通会员] 16 {% elif type == '2' %} 17 欢迎您!{{ user }}[高级会员] 18 {% elif type == '3' %} 19 欢迎您!{{ user }}[管理员] 20 {% else %} 21 <a href = "{% url 'login' %}">登录</a> 22 {% endif %} 23 24 </div> 25 </div> 26 </div> 27 <div id="bigpic"><img src="{% static "img/index.png" %}"></div> 28 </body> 29 </html>
view.py

1 from django.shortcuts import render 2 3 # Create your views here. 4 5 6 def index(request): 7 # 获取查询字符串的数据 8 username = request.GET.get('username') 9 type = request.GET.get('type') 10 11 print(username, type) 12 # 准备出入的数据 13 content = {'user': username, 'type': type} 14 return render(request, 'index.html', context=content) 15 16 17 def login(request): 18 return render(request, 'login.html')
index.html

1 {% load static %} 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <title>youku首页</title> 7 <link type="text/css" rel="stylesheet" href="{% static 'css/basic.css' %}"> 8 </head> 9 <body> 10 <div id="title"> 11 <div> 12 <div id="left">优酷首页</div> 13 <div id="right"> 14 {% if type == '1' %} 15 欢迎您!{{ user }}[普通会员] 16 {% elif type == '2' %} 17 欢迎您!{{ user }}[高级会员] 18 {% elif type == '3' %} 19 欢迎您!{{ user }}[管理员] 20 {% else %} 21 <a href = "{% url 'login' %}">登录</a> 22 {% endif %} 23 24 </div> 25 </div> 26 </div> 27 <div id="bigpic"><img src="{% static "img/index.png" %}"></div> 28 </body> 29 </html>
login.html

1 {% load static %} 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <title>系统登录</title> 7 <style> 8 body{ 9 padding: 0px; 10 margin: 0px; 11 } 12 #title{ 13 width:100%; 14 height: 100px; 15 background-color: yellowgreen; 16 font-size: 32px; 17 font-weight: bold; 18 padding-left: 20px; 19 line-height: 100px; 20 } 21 #bigpic { 22 height: 470px; 23 width:450px; 24 margin: 30px auto; 25 } 26 </style> 27 </head> 28 <body> 29 <div id="title">优酷会员登录</div> 30 <div id="bigpic"><img src="{% static "img/login.png" %}"></div> 31 </body> 32 </html>
访问:
访问login:
5.3 模板中的for 标签,使用bootstrap 和 datatables 展示表格
代码结构:
url.py

1 from django.contrib import admin 2 from django.urls import path 3 from app01 import views as app01_view 4 5 urlpatterns = [ 6 path('admin/', admin.site.urls), 7 path('', app01_view.index, name='home'), 8 path('dt/', app01_view.dt, name='dt'), 9 ]
view.py

1 from django.shortcuts import render 2 3 # Create your views here. 4 5 6 def read_student_from_file(path): 7 """ 8 从文件中读取学生信息 9 数据如下:[{} {} {}] 10 :param path: 11 :return: 12 """ 13 # 定义集合存储数据 14 students = [] 15 infos = ['sno', 'name', 'birthday', 'mobile', 'email', 'address'] 16 try: 17 with open(path, mode='r', encoding='utf-8') as fd: 18 current_line = fd.readline() 19 while current_line: 20 # 切割属性信息 21 student = current_line.strip().replace("\n", "").split(",") 22 # 定义临时集合 23 temp_student = {} 24 for i in range(len(infos)): 25 temp_student[infos[i]] = student[i] 26 # 附加到集合中 27 students.append(temp_student) 28 # 读取下一行 29 current_line = fd.readline() 30 # 返回 31 return students 32 33 except Exception as e: 34 print("读取文件出现异常,具体为: " + str(e)) 35 36 37 def index(request): 38 # 获取文件数据: 39 path = r"D:\Project\Django\Dj010603\app01\static\files\Student.txt" 40 students = read_student_from_file(path) 41 print(students) 42 # 加载HTML页面 43 return render(request, 'index.html', context={'students': students}) 44 45 46 def dt(request): 47 # 获取文件数据: 48 path = r"D:\Project\Django\Dj010603\app01\static\files\Student.txt" 49 students = read_student_from_file(path) 50 print(students) 51 # 加载HTML页面 52 return render(request, 'dt.html', context={'students': students})
index.html

1 {% load static %} 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <title>学生信息</title> 7 <!--添加bootstrap css 文件--> 8 <link type="text/css" rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}"> 9 <style> 10 body{ 11 margin: 0px; 12 padding: 0px; 13 } 14 #title{ 15 width:100%; 16 height: 100px; 17 background-color: yellowgreen; 18 font-size:36px; 19 line-height: 100px; 20 padding-left: 50px; 21 } 22 #content{ 23 width:1200px; 24 height: 800px; 25 margin:10px auto; 26 background-color: lightcyan; 27 28 } 29 table>thead>tr{ 30 background-color: #d58512; 31 } 32 </style> 33 </head> 34 <body> 35 <div id="title">学员相关信息</div> 36 <div id="content"> 37 <table class="table table-striped table-bordered table-hover"> 38 <thead> 39 <tr> 40 <th>序号</th> 41 <th>学号</th> 42 <th>姓名</th> 43 <th>性别</th> 44 <th>出生日期</th> 45 <th>手机号码</th> 46 <th>邮箱地址</th> 47 <th>家庭住址</th> 48 </tr> 49 </thead> 50 <tbody> 51 {% for student in students %} 52 <tr> 53 <!--单独设置序号这一列--> 54 <td style="background-color:skyblue;color:#FFF">{{ forloop.counter }}</td> 55 <td>{{ student.sno }}</td> 56 <td>{{ student.name }}</td> 57 <td>{{ student.gender }}</td> 58 <td>{{ student.birthday }}</td> 59 <td>{{ student.mobile }}</td> 60 <td>{{ student.email }}</td> 61 <td>{{ student.address }}</td> 62 </tr> 63 {% endfor %} 64 </tbody> 65 </table> 66 </div> 67 </body> 68 </html>
dt.html

1 {% load static %} 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <title>学生信息</title> 7 <link type="text/css" rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}"> 8 <!-- 导入DataTable的CSS文件 --> 9 <link type="text/css" rel="stylesheet" href="{% static 'extranal/datatables/css/jquery.dataTables.css' %}"> 10 <!-- 导入DataTable的js文件 --> 11 <script src="{% static 'extranal/datatables/js/jquery.js' %}"></script> 12 <script src="{% static 'extranal/datatables/js/jquery.dataTables.js' %}"></script> 13 14 <style> 15 body{ 16 margin: 0px; 17 padding: 0px; 18 } 19 #title{ 20 width:100%; 21 height: 100px; 22 background-color: yellowgreen; 23 font-size:36px; 24 line-height: 100px; 25 padding-left: 50px; 26 } 27 #content{ 28 width:1200px; 29 height: 800px; 30 margin:50px auto; 31 background-color: lightcyan; 32 33 } 34 table>thead>tr{ 35 background-color: yellowgreen; 36 } 37 </style> 38 <script> 39 $(document).ready( function () { 40 $('#student').DataTable({ 41 language: { 42 "sProcessing": "处理中...", 43 "sLengthMenu": "显示 _MENU_ 项结果", 44 "sZeroRecords": "没有匹配结果", 45 "sInfo": "显示第 _START_ 至 _END_ 项结果,共 _TOTAL_ 项", 46 "sInfoEmpty": "显示第 0 至 0 项结果,共 0 项", 47 "sInfoFiltered": "(由 _MAX_ 项结果过滤)", 48 "sInfoPostFix": "", 49 "sSearch": "搜索:", 50 "sUrl": "", 51 "sEmptyTable": "表中数据为空", 52 "sLoadingRecords": "载入中...", 53 "sInfoThousands": ",", 54 "oPaginate": { 55 "sFirst": "首页", 56 "sPrevious": "上页", 57 "sNext": "下页", 58 "sLast": "末页" 59 }, 60 "oAria": { 61 "sSortAscending": ": 以升序排列此列", 62 "sSortDescending": ": 以降序排列此列" 63 } 64 } 65 }); 66 } ); 67 </script> 68 </head> 69 <body> 70 <div id="title">使用DataTable展示学员信息</div> 71 <div id="content"> 72 <!--table class="table table-striped table-bordered table-hover"--> 73 <table class="table table-striped table-hover table-bordered" id="student"> 74 <thead> 75 <tr> 76 <th>序号</th> 77 <th>学号</th> 78 <th>姓名</th> 79 <th>性别</th> 80 <th>出生日期</th> 81 <th>手机号码</th> 82 <th>邮箱地址</th> 83 <th>家庭住址</th> 84 </tr> 85 </thead> 86 <tbody> 87 {% for student in students %} 88 <tr> 89 <td style="background-color: navy;color:#FFF">{{ forloop.counter }}</td> 90 <td>{{ student.sno }}</td> 91 <td>{{ student.name }}</td> 92 <td>{{ student.gender }}</td> 93 <td>{{ student.birthday }}</td> 94 <td>{{ student.mobile }}</td> 95 <td>{{ student.email }}</td> 96 <td>{{ student.address }}</td> 97 </tr> 98 {% endfor %} 99 </tbody> 100 </table> 101 </div> 102 </body> 103 </html>
使用bootstrap 展示访问:
使用datatable 访问:
【推荐】国内首个AI IDE,深度理解中文开发场景,立即下载体验Trae
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步