java-CCF+杂七杂八
1. 关于for的过程: 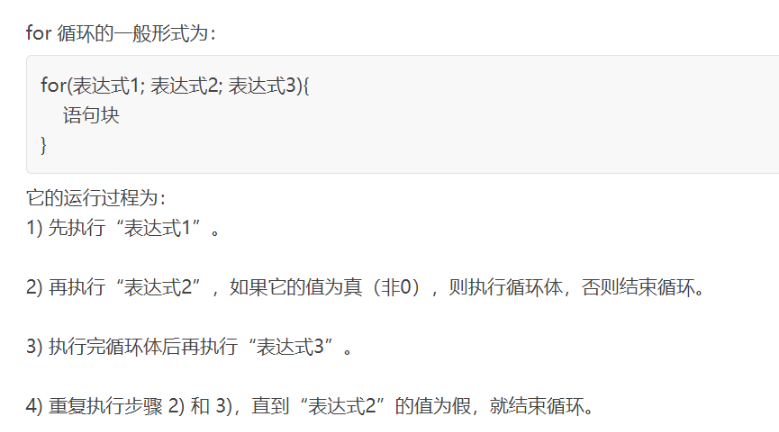 必须满足条件后才能进去循环
2. 遇到的一个形式的++i: https://blog.csdn.net/qq_42933123/article/details/100105858 还挺有意思的。
CCF-CSP考试 全用Java写的
要着重锻炼设计测试样例的能力,我们在考试的使用代码提交上去后当时是没有分数的,得第二天才有,所以我们并不知道我们提交的代码是否能拿满分,这就要我们自己测试好再提交代码。
机器判断输出和正确输出不一样就是0分。
https://blog.csdn.net/wl16wzl/article/details/79344292
https://blog.csdn.net/tigerisland45/article/details/78420636
java输入
Scanner scan_input = new Scanner(System.in);
//接收类型为int整型数字nextInt,并赋值给id,如果小数可以nextDouble/nextFloat、nextLong
int id = scan_input.nextInt();
1 import java.util.Scanner; 2 3 public class Main { 4 5 public static void main(String[] args) { 6 new Main().run(); 7 } 8 9 public void run() { 10 Scanner sc = new Scanner(System.in); 11 12 int n = sc.nextInt(); 13 int[] people = new int[4]; 14 15 for(int i = 0; i < n; i++) { 16 if((i+1) % 7 == 0 || String.valueOf(i+1).contains("7")) { 17 people[i%4] ++; 18 n++; 19 } 20 } 21 22 for(int i = 0; i < 4; i++) { 23 System.out.println(people[i]); 24 } 25 } 26 27 }
1 import java.util.*; 2 public class Main{ 3 public static void main(String[] args){ 4 new Main().run(); 5 } 6 public void run(){ 7 int n;//多少个数后游戏结束 8 int num=1; 9 int count=1; 10 int[] people = new int[4]; 11 Scanner input1 = new Scanner(System.in); 12 int in = input1.nextInt(); 13 while(count<=in){ 14 if(num%7==0 || String.valueOf(num).contains("7")){ 15 people[(num-1)%4]++;//数组是从0开始算的 如果是的话 该计数不动 16 }else{ 17 count++; 18 } 19 num++;//不管是不是 都要++ 20 } 21 for(int i=0;i<4;i++){ 22 System.out.println(people[i]); 23 } 24 } 25 } 26 //String.valueOf(int i) : 将 int 变量 i 转换成字符串 27 //String.contains()方法用法实例教程, 返回true,当且仅当此字符串包含指定的char值序列
//垃圾场选址
java Scanner中的hasNext()方法--判断输入(文件、字符串、键盘等输入流)是否还有下一个输入项,若有,返回true,反之false。
Scanner sc = new Scanner(new File("test.txt"``));
System.out.println(sc.hasNext());若test.txt为空(空格、tab、回车),则输出结果为false;若该文件不为空输出true。
当输入为键盘时,该方法出现阻塞(block),也即等待键盘输入。输入源没有结束,又读不到更多输入项。
Scanner sc = new Scanner(System.in);
System.out.println(sc.hasNext());运行上面的程序,控制台等待键盘输入,输入信息后输出结果为true。
输入:Hello
true由上面的知识,则如下代码则不难理解:
Scanner sc = new Scanner(System.in);
while(sc.hasNext()) {
System.out.println("Input from keyboard:"+ sc.next());
}sc.hasNext()始终等待键盘输入,输入信息后,sc.next()打印出该信息。
Scanner sc = new Scanner(System.in);
此句 表示从控制台获取数据, sc.hasNext() 表示你是否有输入数据, while语句块 表示当你输入数据的时候,就执行输出sc.next()(输出内容) 所以只要你输入数据了,它就可以执行,
所以后台只是开了一块内存,一直未关闭,不算死循环
2hasNext()这个方法是如果此扫描器的输入中有另一个标记,则返回 true。在等待要扫描的输入时,此方法可能阻塞。扫描器将不执行任何输入。所以循环会一直下去。 你可以设置一个终止符,调用hasNext()的重载方法hasNext(String patten):如果下一个标记与从指定字符串构造的模式匹配,则返回 true。扫描器不执行任何输入。 例:以输入"0",结束输出 Scanner sc = new Scanner(System.in); while (!sc.hasNext("0")) { System.out.println(sc.next()); }
Java中关于nextInt()、next()和nextLine()的理解
先看解释:
nextInt(): it only reads the int value, nextInt() places the cursor in the same line after reading the input.
next(): read the input only till the space. It can't read two words separated by space. Also, next() places the cursor in the same line after reading the input.
nextLine(): reads input including space between the words (that is, it reads till the end of line \n). Once the input is read, nextLine() positions the cursor in the next line.
next方法不能得到带空格的字符串而nextLine()方法的结束符只是Enter键
import java.util.Scanner; public class MaxMap { public static void main(String[] args){ Scanner cin = new Scanner(System.in); String n = cin.next(); //cin.nextLine(); String str = cin.nextLine(); System.out.println("END"); System.out.println("next()read:"+n); System.out.println("nextLine()read:"+str); } }
next()只读空格之前的数据,并且cursor指向本行,后面的nextLine()会继续读取前面留下的数据。
想要读取整行,就是用nextLine()。
读取数字也可以使用nextLine(),不过需要转换:Integer.parseInt(cin.nextLine())。
//回收站选址
package epistatic;
import java.util.*;
class points{
int x;
int y;
public points(int x,int y) {
this.x = x;
this.y = y;
}
}
public class Main {
//static:Cannot make a static reference to the non-static method solve(points[]) from the type Main
public static int[] solve(points[] p) { //传递过来的是整个points的数组 就是所有的垃圾点
int[] score = new int[5];
int returnscore = 0;
for(int i = 0;i<p.length;i++) {//上下左右都有垃圾
int count = 0;
returnscore = 0;//!!
for(int j = 0;j<p.length;j++) {//跟其他垃圾点挨个比对一下
if(i!=j) { //别是同一个垃圾点
if(p[i].x==p[j].x && (p[i].y-1)==p[j].y) {
count++;
}if(p[i].x==p[j].x && (p[i].y+1)==p[j].y) {
count++;
}if((p[i].x+1)==p[j].x && p[i].y==p[j].y) {
count++;