-
最长回文子序列
int lpsDp(char * str,int n){ int dp[n][n], tmp; memset(dp,0,sizeof(dp)); for(int i=0; i<n; i++) dp[i][i] = 1; // i 表示 当前长度为 i+1的 子序列 for(int i=1; i<n; i++){ tmp = 0; //考虑所有连续的长度为i+1的子串. 该串为 str[j, j+i] for(int j=0; j+i<n; j++){ //如果首尾相同 if(str[j] == str[j+i]){ tmp = dp[j+1][j+i-1] + 2; }else{ tmp = max(dp[j+1][j+i],dp[j][j+i-1]); } dp[j][j+i] = tmp; } } //返回串 str[0][n-1] 的结果 return dp[0][n-1]; }
-
最长递增子序列
public class Solution { public int lengthOfLIS(int[] nums) { if(nums==null || nums.length == 0) return 0; int len = nums.length; int[] dp = new int[len]; int[] ends = new int[len]; ends[0] = nums[0]; dp[0] = 1; int right = 0; int l=0; int r=0; int m=0; for(int i=0;i<len;i++){ l = 0; r = right; while(l<=r){ m = l + (r-l)/2; if(nums[i]>ends[m]){ l = m + 1; }else{ r = m - 1; } } right = Math.max(right,l); ends[l] = nums[i]; dp[i] = l + 1; } int res = 0; for(int i=0;i<len;i++){ res= Math.max(res,dp[i]); } return res; } }
-
Distinct Subsequences
public class Solution { public int numDistinct(String s, String t) { int len1 = s.length(); int len2 = t.length(); int[][] dp = new int[len2+1][len1+1]; for(int j=0;j<=len1;j++){dp[0][j] = 1;} for(int i=0;i<len2;i++){ for(int j=0;j<len1;j++){ if(t.charAt(i) == s.charAt(j)){ dp[i+1][j+1] = dp[i][j]+dp[i+1][j]; }else{ dp[i+1][j+1] = dp[i+1][j]; } } } return dp[len2][len1]; } }
-
Decode Ways
public class Solution { public int numDecodings(String s) { if(s==null || s.length()==0) return 0; char[] ca = s.toCharArray(); int n = ca.length; if(n==1) return (ca[0]=='0')?0:1; int[] dp = new int[n]; dp[0] = (ca[0]=='0')?0:1; dp[1] = ((ca[0]!='0' && ca[1]!='0')?1:0) + (((ca[0]!='0')&&(ca[0]-'0')*10+(ca[1]-'0')<=26)?1:0); if(n>2){ for(int i=2;i<n;i++){ dp[i] = 0; if(ca[i]!='0'){ dp[i]+=dp[i-1]; } if(ca[i-1]!='0' && ((ca[i-1]-'0')*10+(ca[i]-'0')<=26) ){ dp[i]+=dp[i-2]; } } } return dp[n-1]; } }
-
Edit Distance
public class Solution { public int minDistance(String word1, String word2) { int m = word1.length(); int n = word2.length(); int[][] dp = new int[m + 1][n + 1]; for(int i=0;i<=n;i++){dp[0][i] = i;} for(int i=0;i<=m;i++){dp[i][0] = i;} for(int i=0;i<m;i++){ for(int j=0;j<n;j++){ if(word1.charAt(i) == word2.charAt(j)){ dp[i+1][j+1] = dp[i][j]; }else{ dp[i+1][j+1] = Math.min(Math.min(dp[i+1][j],dp[i][j+1]),dp[i][j])+1; } } } return dp[m][n]; } }
-
Minimum Path Sum
public class Solution { public int minPathSum(int[][] grid) { int m = grid.length; int n = grid[0].length; int[][] dp = new int[m][n]; dp[0][0] = grid[0][0]; for(int i=1;i<m;i++){ dp[i][0] = dp[i-1][0]+grid[i][0]; } for(int j=1;j<n;j++){ dp[0][j] = dp[0][j-1]+grid[0][j]; } for(int i=1;i<m;i++){ for(int j=1;j<n;j++){ dp[i][j] = Math.min(dp[i-1][j],dp[i][j-1])+grid[i][j]; } } return dp[m-1][n-1]; } }
-
Maximum Subarray
public class Solution { public int maxSubArray(int[] nums) { int currMax=nums[0],max=nums[0]; for(int i=1;i<nums.length;i++){ currMax=Math.max(currMax+nums[i],nums[i]); max=Math.max(max,currMax); } return max; } }
-
Unique Paths II
public class Solution { public int uniquePathsWithObstacles(int[][] obstacleGrid) { int width = obstacleGrid[0].length; int[] dp = new int[width]; dp[0] = 1; for (int[] row : obstacleGrid) { for (int j = 0; j < width; j++) { if (row[j] == 1) dp[j] = 0; else if (j > 0) dp[j] += dp[j - 1]; } } return dp[width - 1]; } }
-
Wildcard Matching
boolean[] match = new boolean[m+1]; match[0] = true; for (int i = 0; i < m; i++) { match[i+1] = false; } for (int i = 0; i < n; i++) { if (p.charAt(i) == '*') { for (int j = 0; j < m; j++) { match[j+1] = match[j] || match[j+1]; } } else { for (int j = m-1; j >= 0; j--) { match[j+1] = (p.charAt(i) == '?' || p.charAt(i) == s.charAt(j)) && match[j]; } match[0] = false; } } return match[m];
-
Regular Expression Matching
public class Solution { public boolean isMatch(String s, String p) { boolean[] match = new boolean[s.length()+1]; Arrays.fill(match,false); match[s.length()] = true; for(int i=p.length()-1;i>=0;i--){ if(p.charAt(i) == '*'){ for(int j=s.length()-1;j>=0;j--){ match[j] = match[j] ||match[j+1]&&(p.charAt(i-1)=='.'||s.charAt(j)==p.charAt(i-1)); } i--; }else{ for(int j=0;j<s.length();j++){ match[j] = match[j+1]&&(p.charAt(i)=='.'||p.charAt(i)==s.charAt(j)); } match[s.length()] = false; } } return match[0]; } }
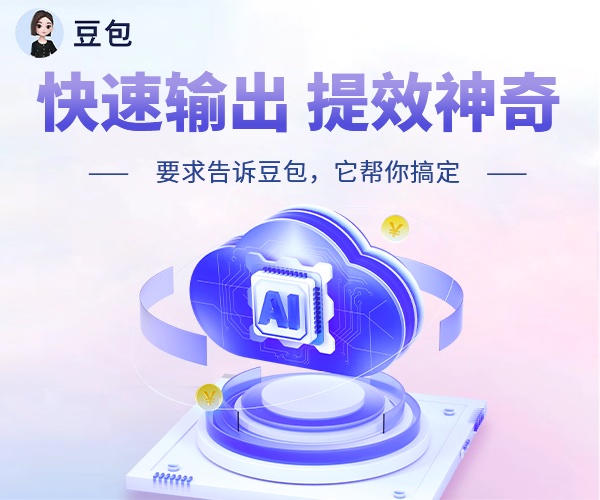