#include<iostream>
using namespace std;
int main(){
/*
算法--冒泡排序(实现升序)
比较相邻元素,若第一个>第二个,则交换
对每一组相邻元素执行上句操作,完成后,找到第一个最大值
重复以上步骤,每次比较次数-1,直到不需要再比较
*/
int arr[] = {4, 2, 8, 0, 5, 7, 1, 3, 9};
cout << "排序前数组:" << endl;
for (int i=0; i<9; i++){
cout << arr[i] << " ";
}
cout << endl;
// 排序总轮数=数组元素个数-1
// 每轮对比次数=数组元素个数-排序轮数-1
for (int i=0; i<9-1; i++){ // 排序轮数=8
for (int j=0; j<9-i-1; j++){ // 每轮对比次数
if (arr[j] > arr[j+1]){
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
cout << "排序后数组:" << endl;
for (int i=0; i<9; i++){
cout << arr[i] << " ";
}
cout << endl;
/*
二维数组
*/
// 二维数组4种定义方式
// 1 数据类型 数组名[行数][列数]
int arr2[2][3];
arr2[0][0] = 1;
arr2[0][1] = 2;
arr2[0][2] = 3;
arr2[1][0] = 4;
arr2[1][1] = 5;
arr2[1][2] = 6;
for (int i=0; i<2; i++){
for (int j=0; j<3; j++){
cout << arr2[i][j] << " ";
}
cout << endl;
}
cout << endl;
// 2 数据类型 数组名[行数][列数] = { {数据1, 数据2}, {数据2, 数据4}} -------推荐使用
int arr3[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
for (int i=0; i<2; i++){
for (int j=0; j<3; j++){
cout << arr3[i][j] << " ";
}
cout << endl;
}
cout << endl;
// 3 数据类型 数组名[行数][列数] = {数据1, 数据2, 数据2, 数据4}
int arr4[2][3] = {1, 2, 3, 4, 5, 6}; // 根据行数和列数会自动划分赋值数据
for (int i=0; i<2; i++){
for (int j=0; j<3; j++){
cout << arr4[i][j] << " ";
}
cout << endl;
}
cout << endl;
// 4 数据类型 数组名[][列数] = {数据1, 数据2, 数据2, 数据4}
int arr5[][3] = {1, 2, 3, 4, 5, 6}; // 只根据列数也能自动划分赋值数据
for (int i=0; i<2; i++){
for (int j=0; j<3; j++){
cout << arr5[i][j] << " ";
}
cout << endl;
}
cout << endl;
return 0;
}
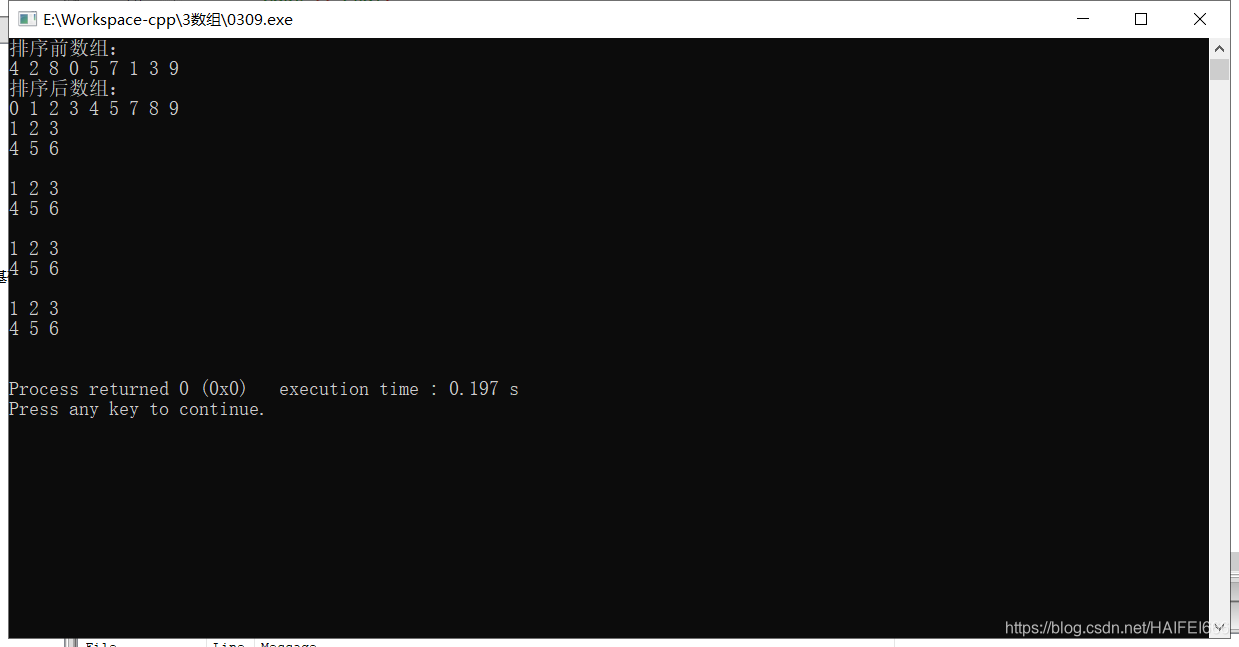