#include<iostream>
#include<string>
using namespace std;
/*
结构体
一些数据类型的集合
用户自定义的数据类型
允许存放不同的数据类型
*/
// 结构体定义
struct Student{
string name;
int age;
int score; // 注意,最后一项元素末尾也要加分号,不然报错
};
int main(){
// 结构体变量的三种创建方式
// 前两种方式用的较多
// 1
struct Student s1;
s1.name = "张三"; // 给结构体变量的属性赋值
s1.age = 18;
s1.score = 100;
cout << "姓名:" << s1.name << ",年龄:" << s1.age << ",分数:" << s1.score << endl;
// 2
struct Student s2 = {"李四", 19, 80};
cout << "姓名:" << s2.name << ",年龄:" << s2.age << ",分数:" << s2.score << endl;
// 3 在创建结构体的同时创建空变量
struct Student_1{
string name;
int age;
int score;
}s3;
s3.name = "王五";
s3.age = 20;
s3.score = 60;
cout << "姓名:" << s3.name << ",年龄:" << s3.age << ",分数:" << s3.score << endl;
// 注意,C++中创建结构体变量时struct关键字可以省略,例如:Student s1,Student s2
return 0;
}
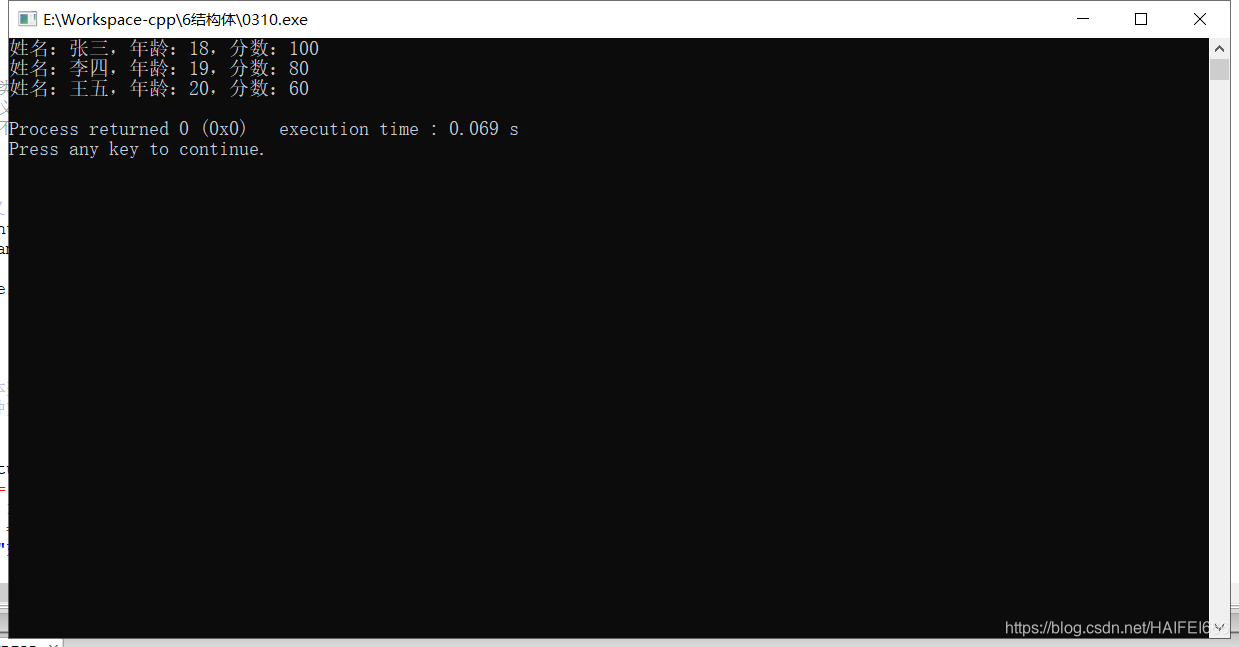