#include<iostream>
#include<string>
using namespace std;
/*
4.1 封装
案例*2
*/
// ------------------------------------------------------------------------------------------------
class Cube{
private:
int lenth;
int width;
int hight;
public:
void set_lenth(int _lenth){
lenth = _lenth;
}
int get_lenth(){
return lenth;
}
void set_width(int _width){
width = _width;
}
int get_width(){
return width;
}
void set_hight(int _hight){
hight = _hight;
}
int get_hight(){
return hight;
}
int cal_s(){
return 2*lenth*width + 2*width*hight + 2*lenth*hight;
}
int cal_v(){
return lenth*width*hight;
}
bool is_same_byclass(Cube & c){ // 成员函数判断两个立方体是否相等 核心点1-1
if (lenth==c.get_lenth() &&
width==c.get_width() &&
hight==c.get_hight()){
return true;
}
return false;
}
};
bool is_same(Cube & c1, Cube & c2){ // 全局函数判断两个立方体是否相等 核心点1-2
if (c1.get_lenth()==c2.get_lenth() &&
c1.get_width()==c2.get_width() &&
c1.get_hight()==c2.get_hight()){
return true;
}
return false;
}
// ------------------------------------------------------------------------------------------------
// ================================================================================================
class Point{
private:
int x;
int y;
public:
void set_x(int _x){
x = _x;
}
int get_x(){
return x;
}
void set_y(int _y){
y = _y;
}
int get_y(){
return y;
}
};
class Circle{
private:
int r; // 半径
// 核心点2:在类中可以使用另一个类作为本类的成员
Point center; // 圆心
public:
void set_r(int _r){
r = _r;
}
int get_r(){
return r;
}
void set_center(Point _center){
center = _center;
}
Point get_center(){
return center;
}
};
void is_incircle(Circle & c, Point & p){ // 判断点和圆的关系
// 两点之间距离=[(x1-x2)^2 + (y1-y2)^2]的开平方
int p_distance =
(c.get_center().get_x() - p.get_x())*(c.get_center().get_x() - p.get_x()) +
(c.get_center().get_y() - p.get_y())*(c.get_center().get_y() - p.get_y());
int r_distance = c.get_r() * c.get_r();
if(p_distance == r_distance){
cout << "点在圆上" << endl;
}else if(p_distance > r_distance){
cout << "点在圆外" << endl;
}else{
cout << "点在圆内" << endl;
}
}
// ================================================================================================
int main(){
// 案例1 立方体
Cube c1;
c1.set_lenth(10);
c1.set_width(10);
c1.set_hight(10);
cout << c1.cal_s() << endl;
cout << c1.cal_v() << endl;
Cube c2;
c2.set_lenth(10);
c2.set_width(10);
c2.set_hight(20);
bool result = is_same(c1, c2); // 利用全局函数判断
if (result){
cout << "c1c2相等" << endl;
}else{
cout << "c1c2不等" << endl;
}
result = c1.is_same_byclass(c2); // 利用成员函数判断
if (result){
cout << "c1c2相等" << endl;
}else{
cout << "c1c2不等" << endl;
}
// 案例2 点圆关系
Point center;
center.set_x(10);
center.set_y(0);
Circle c;
c.set_center(center);
c.set_r(10);
Point p;
p.set_x(10);
p.set_y(10);
is_incircle(c, p); // 点在圆上
p.set_x(10);
p.set_y(11);
is_incircle(c, p); // 点在圆外
p.set_x(10);
p.set_y(9);
is_incircle(c, p); // 点在圆内
// 核心点3 大型项目分文件编写规范,见项目CirclePointClass
return 0;
}
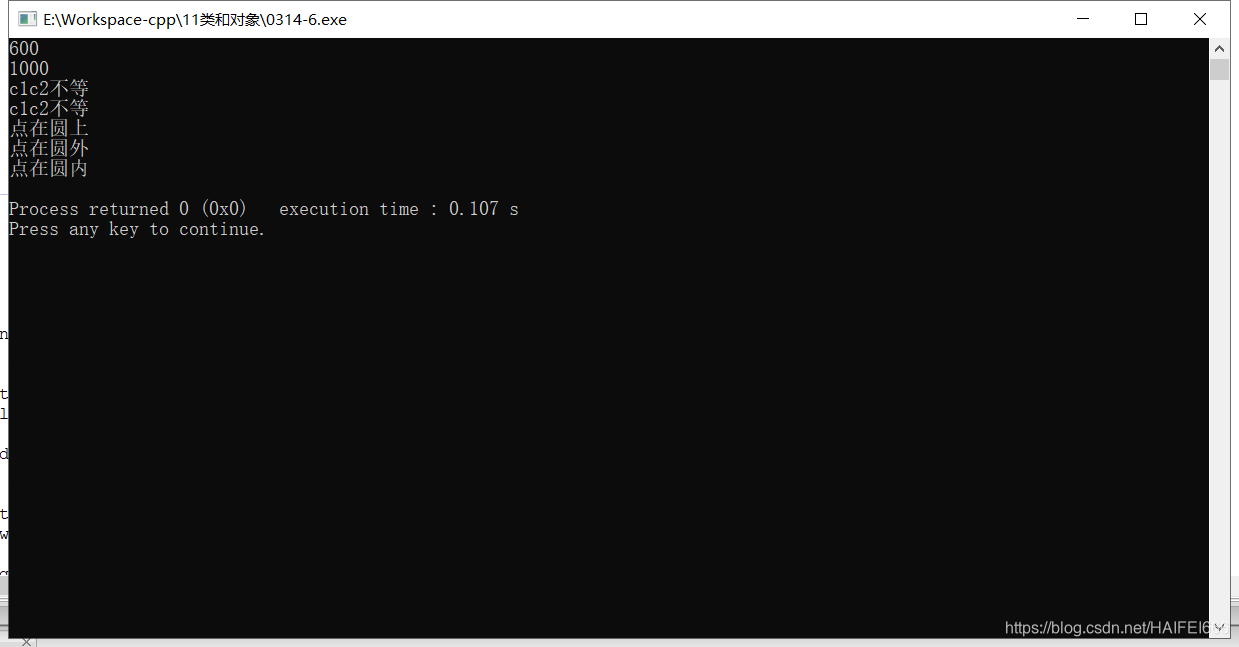