#include<stdlib.h>
#include<iostream>
#include<string>
using namespace std;
/*
4.7.6 多态案例3--电脑组装
案例描述:
电脑主要组成部件为 CPU(用于计算),显卡(用于显示),内存条(用于存储)
将每个零件封装出抽象基类,并且提供不同的厂商生产不同的零件,例如Intel厂商和Lenovo厂商
创建电脑类提供让电脑工作的函数,并且调用每个零件工作的接口
测试时组装三台不同的电脑进行工作
*/
// ---------------------------------------------------------
class CPU{ // 抽象CPU类
public:
virtual void calculate() = 0; // 抽象计算函数
};
class GPU{ // 抽象GPU类
public:
virtual void display() = 0; // 抽象显示函数
};
class RAM{ // 抽象RAM类
public:
virtual void storage() = 0; // 抽象存储函数
};
// ---------------------------------------------------------
class PC{
private:
// 零件指针
CPU * cpu;
GPU * gpu;
RAM * ram;
public:
PC(CPU * _cpu, GPU * _gpu, RAM * _ram){ // 构造
cpu = _cpu;
gpu = _gpu;
ram = _ram;
}
void dowork(){ // 自定义工作函数,零件调用工作接口
cpu->calculate();
gpu->display();
ram->storage();
}
// 提供析构 释放3种零件
~PC(){
if(cpu != NULL){
delete cpu;
cpu = NULL;
}
if(gpu != NULL){
delete gpu;
gpu = NULL;
}
if(ram != NULL){
delete ram;
ram = NULL;
}
}
};
// ---------------------------------------------------------
class InterCPU : public CPU{
public:
virtual void calculate(){
cout << "inter cpu 开始计算" << endl;
}
};
class InterGPU : public GPU{
public:
virtual void display(){
cout << "inter gpu 开始显示" << endl;
}
};
class InterRAM : public RAM{
public:
virtual void storage(){
cout << "inter ram 开始存储" << endl;
}
};
// ---------------------------------------------------------
class LenovoCPU : public CPU{
public:
virtual void calculate(){
cout << "Lenovo cpu 开始计算" << endl;
}
};
class LenovoGPU : public GPU{
public:
virtual void display(){
cout << "Lenovo gpu 开始显示" << endl;
}
};
class LenovoRAM : public RAM{
public:
virtual void storage(){
cout << "Lenovo ram 开始存储" << endl;
}
};
// ---------------------------------------------------------
void test1(){
// 通常说的多态就是动态多态
// 动态多态的使用条件:父类的指针或者引用 执行 子类对象
CPU * interCPU = new InterCPU; // 实现了多态
GPU * interGPU = new InterGPU;
RAM * interRAM = new InterRAM;
PC * pc1 = new PC(interCPU, interGPU, interRAM);
cout << "第1台电脑开始工作:" << endl;
pc1->dowork();
delete pc1;
cout << endl;
CPU * lenovoCPU = new LenovoCPU;
GPU * lenovoGPU = new LenovoGPU;
RAM * lenovoRAM = new LenovoRAM;
PC * pc2 = new PC(lenovoCPU, lenovoGPU, lenovoRAM);
cout << "第2台电脑开始工作:" << endl;
pc2->dowork();
delete pc2;
cout << endl;
PC * pc3 = new PC(new InterCPU, new LenovoGPU, new LenovoRAM);
cout << "第3台电脑开始工作:" << endl;
pc3->dowork();
delete pc3;
}
int main()
{
test1();
system("pause");
return 0;
}
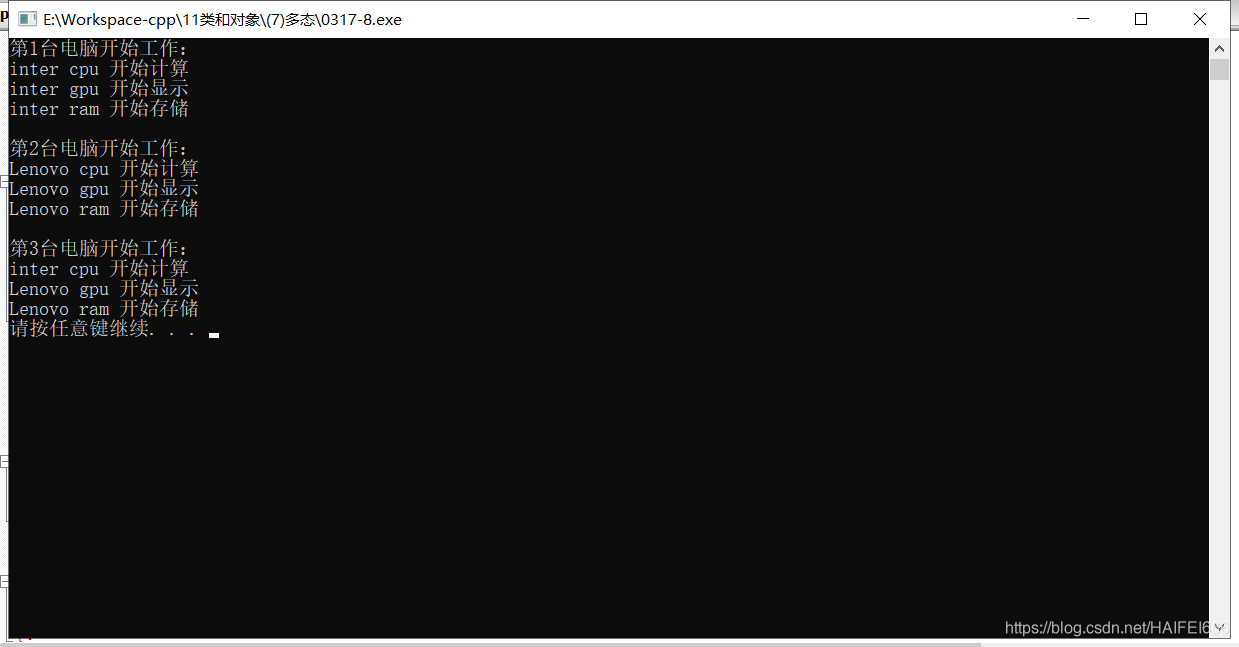