#include<iostream>
#include<stdlib.h>
#include<string>
using namespace std;
/*
3.1.5 string查找和替换
int find(const string& str, int pos = 0) const; //查找str第一次出现位置,从pos开始查找
int find(const char* s, int pos = 0) const; //查找s第一次出现位置,从pos开始查找
int find(const char* s, int pos, int n) const; //从pos位置查找s的前n个字符第一次位置
int find(const char c, int pos = 0) const; //查找字符c第一次出现位置
int rfind(const string& str, int pos = npos) const; //查找str最后一次位置,从pos开始查找
int rfind(const char* s, int pos = npos) const; //查找s最后一次出现位置,从pos开始查找
int rfind(const char* s, int pos, int n) const; //从pos查找s的前n个字符最后一次位置
int rfind(const char c, int pos = 0) const; //查找字符c最后一次出现位置
string& replace(int pos, int n, const string& str); //替换从pos开始n个字符为字符串str
string& replace(int pos, int n,const char* s); //替换从pos开始的n个字符为字符串s
*/
void test1()
{
string s1 = "abcdefgde";
int position = s1.find("de"); //子串de第一次出现的位置为下标3
if(position == -1)
{
cout << "未找到指定子串";
}
else
{
cout << position << endl;
}
position = s1.find("dg"); //子串dg不存在,返回-1
if(position == -1)
{
cout << "未找到指定子串" << endl;
}
else
{
cout << position << endl;
}
//区别:find从左往右查找,rfind从右往左查找
int pos = s1.rfind("de");
cout << pos << endl;
}
void test2()
{
string s1 = "abcdefg";
s1.replace(1, 3, "666666"); //从下标1位置开始的含有3个字符的子串被替换为指定的新子串
cout << s1 << endl;
}
int main()
{
test1(); //查找
test2(); //替换
system("pause");
return 0;
}
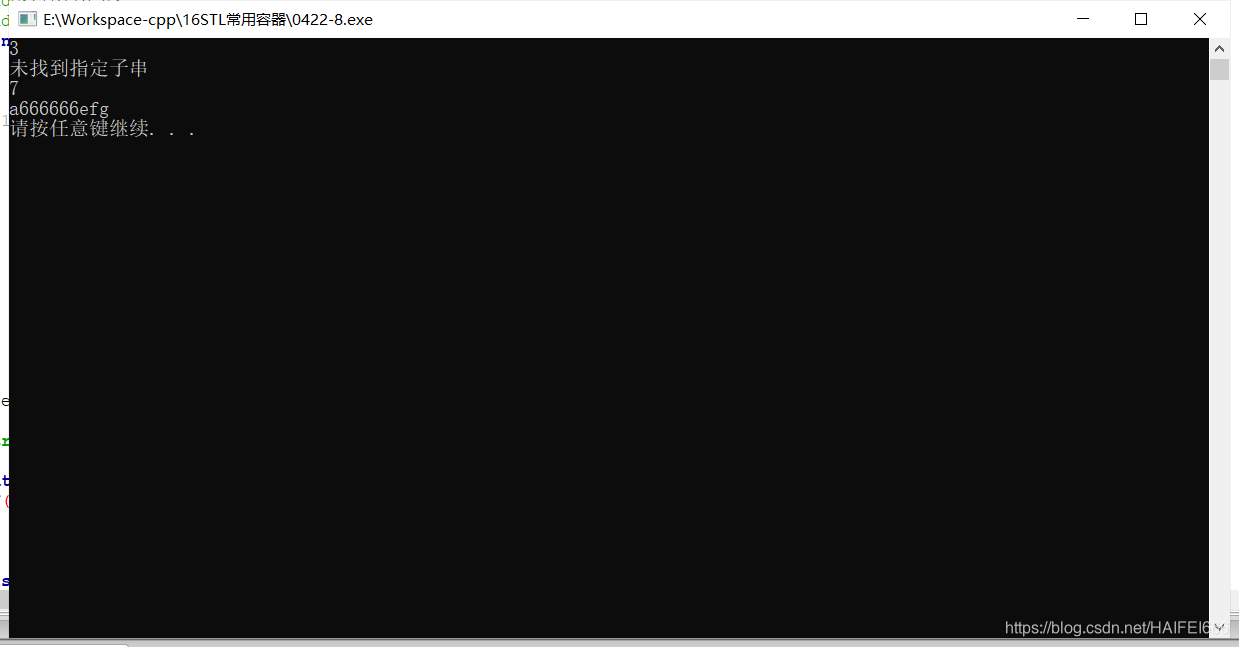