多功能的条码读取控件如何构建Web条形码阅读器
Dynamsoft Barcode Reader SDK一款多功能的条码读取控件,只需要几行代码就可以将条码读取功能嵌入到Web或桌面应用程序。这可以节省数月的开发时间和成本。能支持多种图像文件格式以及从摄像机或扫描仪获取的DIB格式。使用Dynamsoft Barcode Reader SDK,你可以创建强大且实用的条形码扫描仪软件,以满足你的业务需求。
Blazor是Microsoft开发的Web框架。它使开发人员可以使用C#和HTML创建Web应用程序。但是,在开发Blazor项目时不可避免地调用现有的JavaScript API。本文将为希望使用Blazor WebAssembly和Dynamsoft JavaScript Barcode SDK构建Web条形码阅读器应用程序的Web开发人员提供帮助。
Blazor提供了两个模板,Blazor WebAssembly和Blazor Server。
Blazor服务器
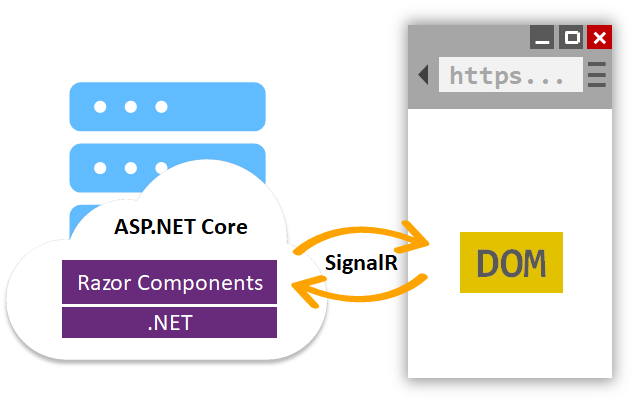
Blazor服务器在服务器端运行客户端UI逻辑,并通过Websocket发送UI更改。为了方便地处理JavaScript互操作调用,我选择Blazor WebAssembly来实现Web条形码读取器示例。
在Blazor WebAssembly项目中调用Dynamsoft JavaScript条形码API
使用Blazor WebAssembly模板创建Blazor应用程序:
dotnet new blazorwasm -o BlazorBarcodeSample
然后向项目添加一个新页面:
cd BlazorBarcodeSample dotnet new razorcomponent -n BarcodeReader -o Pages
之后,将BarcodeReader属性添加到Pages / Index.razor:
@page "/" <h1>Hello, world!</h1> Welcome to your new app. <SurveyPrompt Title="How is Blazor working for you?" /> <BarcodeReader />
到目前为止,我们可以运行该应用程序并查看主页:
dotnet run
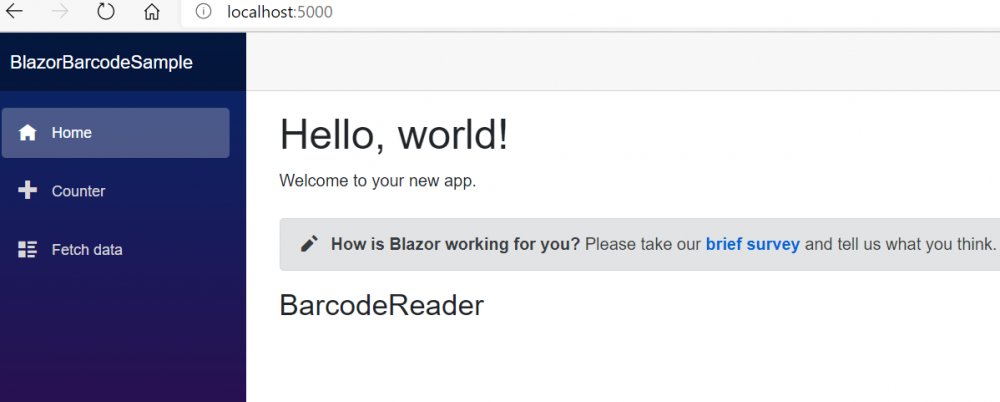
首次运行该应用程序时,我们可以打开开发人员控制台以观察发生了什么。

Web浏览器将获取并缓存一个dotnet.wasm文件和一些* .dll文件。
我们可以通过添加<button>和<p>元素对UI进行一些更改。
@page "/" <h1>Blazor Barcode Sample</h1> <BarcodeReader />
BarcodeReader.razor:
@page "/barcodereader" <button class="btn btn-primary" >Read Barcodes from Files</button> <p style="color:green;font-style:italic">@result</p> @code { private static String result = "No Barcode Found"; }
重新运行该应用以查看UI更改:

如果重新打开开发者控制台,我们将发现仅再次获取了BlazorBarcodeSample.dll。
下一步是为应用程序提供条形码扫描功能。
将Dynamsoft JavaScript条形码SDK添加到wwwroot / index.html,然后创建一个jsInterop.js文件以实现JavaScript和.NET之间的互操作:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no" /> <title>BlazorBarcodeSample</title> <base href="/" /> <link href="css/bootstrap/bootstrap.min.css" rel="stylesheet" /> <link href="css/app.css" rel="stylesheet" /> <script src="https://cdn.jsdelivr.net/npm/dynamsoft-javascript-barcode@7.6.0/dist/dbr.js" data-productKeys="LICENSE-KEY"></script> </head> <body> <script src="_framework/blazor.webassembly.js"></script> <script src="jsInterop.js"></script> </body> </html>
打开jsInterop.js。添加以下代码以初始化Dynamsoft Barcode Reader:
var barcodereader = null; (async () => { barcodereader = await Dynamsoft.BarcodeReader.createInstance(); await barcodereader.updateRuntimeSettings('balance'); let settings = await barcodereader.getRuntimeSettings(); barcodereader.updateRuntimeSettings(settings); })();
为了与.NET进行交互,我们可以使用JavaScript变量来存储.NET对象的引用:
var dotnetRef = null; window.jsFunctions = { init: function(obj) { dotnetRef = obj; }, };
为了将.NET对象引用传递给JavaScript,请注入JavaScript运行时并重写BarcodeReader.razor中的OnInitialized()方法 :
@inject IJSRuntime JSRuntime @code { private static String result = "No Barcode Found"; protected override void OnInitialized() { JSRuntime.InvokeVoidAsync("jsFunctions.init", DotNetObjectReference.Create(this)); } }
我们要做的是从磁盘驱动器中选择一个图像文件并从该图像中读取条形码。因此,添加一个按钮单击事件以调用JavaScript条形码解码方法:
<button class="btn btn-primary" @onclick="ReadBarcodes">Read Barcodes from Files</button> @code { private static String result = "No Barcode Found"; protected override void OnInitialized() { JSRuntime.InvokeVoidAsync("jsFunctions.init", DotNetObjectReference.Create(this)); } public async Task ReadBarcodes() { await JSRuntime.InvokeVoidAsync( "jsFunctions.selectFile"); } }
同时,创建一个.NET函数以从JavaScript接收条形码解码结果:
[JSInvokable] public void ReturnBarcodeResultsAsync(String text) { result = text; this.StateHasChanged(); }
不要忘记使用StateHasChanged()刷新UI。
这是JavaScript实现:
window.jsFunctions = { init: function(obj) { dotnetRef = obj; }, selectFile: function () { let input = document.createElement("input"); input.type = "file"; input.onchange = async function () { let file = input.files[0]; try { await barcodereader.decode(file).then((results) => { let txts = []; try { for (let i = 0; i < results.length; ++i) { txts.push(results[i].BarcodeText); } let barcoderesults = txts.join(', '); if (txts.length == 0) { barcoderesults = 'No barcode found'; } console.log(barcoderesults); if (dotnetRef) { dotnetRef.invokeMethodAsync('ReturnBarcodeResultsAsync', barcoderesults); } } catch (e) { } }); } catch (error) { alert(error); } }; input.click(); }, };
现在,我们可以使用一些条形码图像来测试Blazor WebAssembly应用程序。

最后,让我们删除FetchData.razor,Counter.razor,SurveyPrompt.razor及其相关代码以优化项目。
