【C++类与对象】实验四(二)
- 实现画图类
-
1 #ifndef GRAPH_H 2 #define GRAPH_H 3 4 // 类Graph的声明 5 class Graph { 6 public: 7 Graph(char ch, int n); // 带有参数的构造函数 8 void draw(); // 绘制图形 9 void changecolor(); 10 private: 11 char symbol; 12 int size; 13 char selcolor; 14 }; 15 16 17 #endif
1 // 类graph的实现 2 #include<cstdio> 3 #include "graph.h" 4 #include <iostream> 5 using namespace std; 6 7 // 带参数的构造函数的实现 8 Graph::Graph(char ch, int n): symbol(ch), size(n) { 9 } 10 11 12 // 成员函数draw()的实现 13 // 功能:绘制size行,显示字符为symbol的指定图形样式 14 // size和symbol是类Graph的私有成员数据 15 void Graph::draw() { 16 int i,j,k; 17 for(i=1;i<=size;i++){ 18 19 k=size-i; 20 while(k--){ 21 cout<<' '; 22 } 23 k=0; 24 while(k<2*i-1){ 25 cout<<symbol; 26 k++; 27 } 28 k=size-i; 29 while(k--){ 30 cout<<' '; 31 } 32 cout<<endl; 33 } 34 }
-
1 #include <iostream> 2 #include "graph.h" 3 #include"graph.cpp" 4 5 using namespace std; 6 7 8 int main() { 9 Graph graph1('*',5), graph2('$',7) ; // 定义Graph类对象graph1, graph2 10 graph1.draw(); // 通过对象graph1调用公共接口draw()在屏幕上绘制图形 11 graph2.draw(); // 通过对象graph2调用公共接口draw()在屏幕上绘制图形 12 char a[10]; 13 system("color 19");//系统设置颜色 14 return 0; 15 }
- 运行截图:其中关于修改颜色,我用的函数库里面的函数system(“color背景色/前景色”)
-
- 实现分数类

1 #include <iostream> 2 #include<cmath> 3 #include"fraction.h" 4 using namespace std; 5 6 // 带参数的构造函数的实现 7 Fraction::Fraction(int t, int b): top(t), bottom(b) { 8 } 9 Fraction::Fraction(): top(0), bottom(1) { 10 } 11 Fraction::Fraction(int t): top(t), bottom(1) { 12 } 13 Fraction::Fraction(Fraction &f1): top(f1.top), bottom(f1.bottom) { 14 } 15 void Fraction::shuru(){ 16 cout<<"请输入分子,分母:"; 17 int a,b; 18 cin>>a>>b; 19 20 top=a; 21 bottom=b; 22 } 23 void Fraction::jia(Fraction &f1){ 24 if(bottom==f1.bottom){ 25 top+=f1.top; 26 } 27 else{ 28 top=top*f1.bottom+bottom*f1.top; 29 bottom=bottom*f1.bottom; 30 } 31 } 32 void Fraction::jian(Fraction &f1){ 33 if(bottom==f1.bottom){ 34 top-=f1.top; 35 } 36 else{ 37 top=top*f1.bottom-bottom*f1.top; 38 bottom=bottom*f1.bottom; 39 } 40 } 41 void Fraction::cheng(Fraction &f1){ 42 bottom*=f1.bottom; 43 top*=f1.top; 44 } 45 void Fraction::chu(Fraction &f1){ 46 bottom*=f1.top; 47 top*=f1.bottom; 48 } 49 void Fraction::printfraction(){ 50 int a,b,r; 51 a=top; 52 b=bottom; 53 r=a%b; 54 while(r){ 55 a=b; 56 b=r; 57 r=a%b; 58 } 59 top=top/b; 60 bottom=bottom/b; 61 if(top<0&&bottom>0||top>0&&bottom<0) 62 cout<<"-"<<abs(top)<<"/"<<abs(bottom)<<endl; 63 if(top>=0&&bottom>0||top<=0&&bottom<0) 64 cout<<abs(top)<<"/"<<abs(bottom)<<endl; 65 66 67 } 68 void Fraction::printinteger(){ 69 double k; 70 k=double(top)/(double(bottom)); 71 cout<<k<<endl; 72 } 73 74 75 #

1 #ifndef FRACTION_H 2 #define FRACTION_H 3 4 // 类Graph的声明 5 class Fraction { 6 public: 7 Fraction(int t,int b);//构造函数 8 Fraction(); 9 Fraction(int t); 10 Fraction(Fraction &f1); 11 void shuru();//输入 12 void jia(Fraction &f1);//加法 13 void jian(Fraction &f1);// 减法 14 void cheng(Fraction &f1);//乘法 15 void chu(Fraction &f1);//除法 16 void printfraction();//输出分数 17 void printinteger(); //输出整数 18 19 private: 20 int top; 21 int bottom; 22 }; 23 24 25 #endif

1 #include <iostream> 2 #include"fraction.h" 3 4 using namespace std; 5 6 int main() 7 { 8 Fraction a,b(3,4),c(5),d,f;//初始化任意两个对象 9 a.printfraction(); 10 b.printfraction(); 11 c.printfraction(); 12 //d(2,5); 为啥在定义一个普通变量,比如int a; a=1;但是定义类,fraction a; a(,) 是错的,因为a有默认的参数值。 13 14 15 a.shuru(); 16 b.shuru(); 17 cout<<"分数相加:"; 18 a.jia(b); 19 a.printfraction(); 20 cout<<"分数相减:"; 21 a.jian(b); 22 a.printfraction(); 23 cout<<"分数相乘:"; 24 a.cheng(b); 25 a.printfraction(); 26 cout<<"分数相除:"; 27 a.chu(b); 28 a.printfraction(); 29 a.printinteger(); 30 31 return 0; 32 }
测试截图
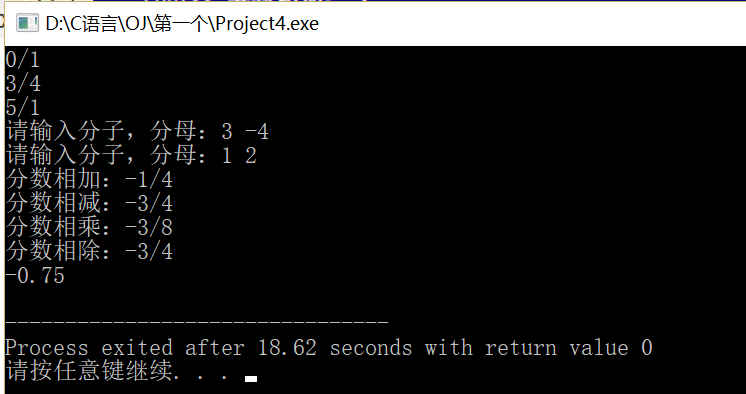
3.体会:
int a;
a=2;//对的
(class)Fraction a;
a(2,1);//出错
我认为原因是,1.a有默认构造函数,在定义时,就默认初始化a的值。2.a(,)在类中并没有定义这个函数。