使用drf_yasg生成drf接口文档 会有参数类型,比drf自己的更完善
1.安装库
**pip install drf-yasg**
2.配置setting
INSTALLED_APPS = [
...
'drf_yasg',
...
]
3.配置全局路由文件urls.py
点击查看代码
from drf_yasg.views import get_schema_view
from drf_yasg import openapi
schema_view = get_schema_view(
openapi.Info(
title="API接口文档平台", # 必传
default_version='v1', # 必传
description="这是一个接口文档",
license=openapi.License(name="BSD License"),
),
public=True,
# permission_classes=(permissions.AllowAny,), # 权限类
)
urlpatterns = [
...
path('swagger/', schema_view.with_ui('swagger', cache_timeout=0), name='schema-swagger-ui'),
path('redoc/', schema_view.with_ui('redoc', cache_timeout=0), name='schema-redoc'),
]
4.查看
http://127.0.0.1:8000/swagger/
5.重点
对于没有使用drf序列化的一些高层接口,我们可以使用底层的APIView来创建视图集,并使用drf_yasg生成接口文档。
点击查看代码
from django.shortcuts import render, HttpResponse
from rest_framework.views import APIView
from drf_yasg.utils import swagger_auto_schema
from drf_yasg import openapi
from drf_yasg.openapi import Schema, Response
class NoneModelViewSet(APIView):
@swagger_auto_schema(
tags=['没模型测试'],
operation_description='没模型get',
operation_summary='没模型摘要get', )
def get(self, request, *args, **kwargs):
print(11111, request.GET)
return HttpResponse('ok')
@swagger_auto_schema(
tags=['没模型测试'],
operation_description='没模型post',
operation_summary='没模型摘要post',
request_body=Schema(type=openapi.TYPE_OBJECT,
required=['account_id'],
properties={
'account_id': Schema(
description='用户id example: 12354',
type=openapi.TYPE_INTEGER),
'telephone': Schema(
description='手机号码 example: 19811111111',
type=openapi.TYPE_STRING),
'code': Schema(
description='兑换码 example: 15462fd',
type=openapi.TYPE_STRING),
'disguise_change': Schema(
description='是否是伪变化 example: 0表示不是,1 表示是',
type=openapi.TYPE_INTEGER, enum=[0, 1]),
}),
responses={
400: Response(description='操作失败', examples={'json': {'code': -1, 'msg': '失败原因'}}),
200: Response(description='操作成功', examples={'json': {'code': 0, 'msg': '成功'}})
}
)
def post(self, request):
print(request.body)
return HttpResponse('ok')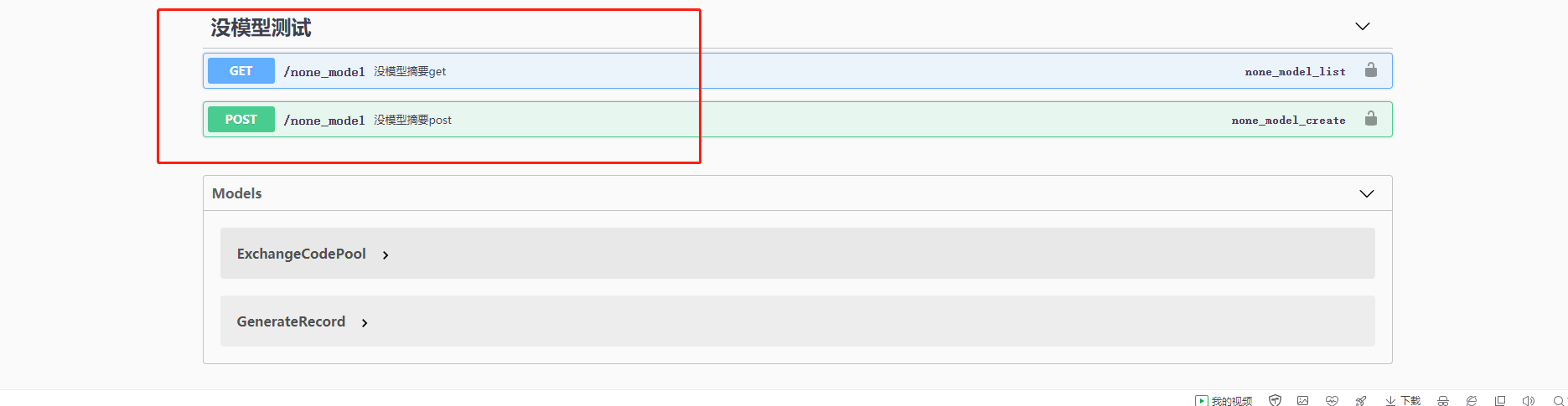
urls.py
点击查看代码
urlpatterns = [
path('index', index),
path('none_model', NoneModelViewSet.as_view())
]
查看接口文档
6.优化模型类视图集
如果直接生成模型类视图集,就会像4显示的一样,标签是路由名,不像5中,显示的是自定义的汉字。所以用重写父类方法,然后用swagger_auto_schema装饰器对标签进行命名。代码如下:
点击查看代码
class GenerateRecordViewSet(viewsets.ModelViewSet):
queryset = GenerateRecord.objects.all()
serializer_class = GenerateRecordSerializer
pagination_class = LargeResultsSetPagination
http_method_names = ['get', 'post', 'put']
tag = ['兑换码']
@swagger_auto_schema(tags=tag, operation_summary='获取所有兑换码', operation_description='获取所有兑换码')
def list(self, request, *args, **kwargs):
return super(GenerateRecordViewSet, self).list(request, *args, **kwargs)
@swagger_auto_schema(tags=tag, operation_summary='获取单个兑换码', operation_description='通过id获取单个兑换码信息')
def retrieve(self, request, *args, **kwargs):
return super(GenerateRecordViewSet, self).retrieve(request, *args, **kwargs)
@swagger_auto_schema(tags=tag, operation_summary='生成兑换码', operation_description='生成兑换码')
def create(self, request, *args, **kwargs):
return super(GenerateRecordViewSet, self).create(request, *args, **kwargs)
@swagger_auto_schema(tags=tag, operation_summary='兑换码上下架', operation_description='修改有效标志')
def update(self, request, *args, **kwargs):
return super(GenerateRecordViewSet, self).update(request, *args, **kwargs)