1. 单链表含头结点模型示意图如下:
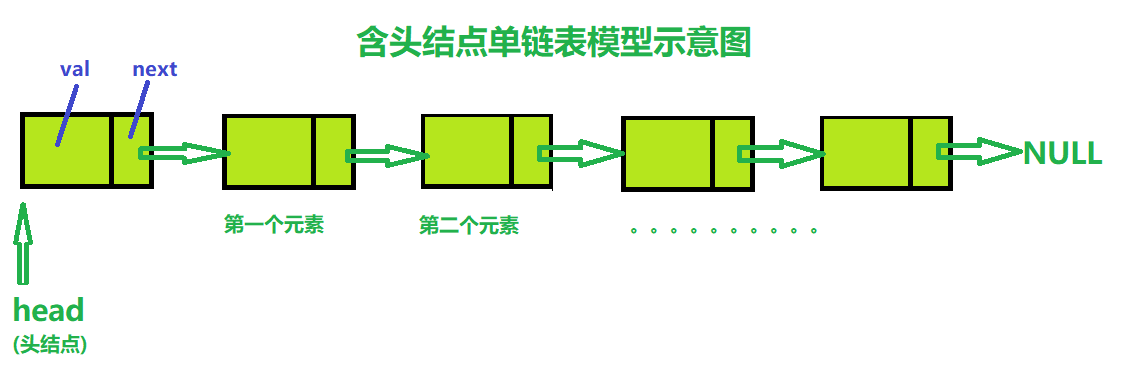
2. 单链表节点结构定义如下:
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
3. 单链表的基本操作函数如下:
- ListNode* createList(); // 手动输入创建一个链表
- void printList(ListNode* head); // 打印链表数据
- int getListLength(ListNode* head); // 获取链表长度
- ListNode* insertList(ListNode* head, int pos, int data); // 插入数据到链表里
- ListNode* deleteList(ListNode* head, int pos); // 删除链表节点数据
4. 具体代码实现如下:
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* createList() {
ListNode* head = new ListNode(0);
ListNode* p = head;
ListNode* node = NULL;
int flag = 1;
int data = 0;
while (flag) {
cout << "Please input the data: " << endl;
cin >> data;
if (data != 0) {
node = new ListNode(data);
p->next = node;
p = node;
}
else {
flag = 0;
}
}
return head;
}
void printList(ListNode* head) {
if (head == NULL || head->next == NULL) {
cout << "The list is empty!" << endl;
return;
}
ListNode* p = head->next;
cout << "The list values are as follows: " << endl;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int getListLength(ListNode* head) {
if (head == NULL || head->next == NULL) {
cout << "The list is empty!" << endl;
return 0;
}
ListNode* p = head->next;
int len = 0;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
ListNode* insertList(ListNode* head, int pos, int data) {
if (pos <= 0) {
cout << "The pos is error!" << endl;
return head;
}
if (head == NULL) {
head = new ListNode(0);
}
ListNode* p = head;
int index = 1;
while (p != NULL && index < pos) {
p = p->next;
index++;
}
ListNode* node = new ListNode(data);
if (p != NULL) {
node->next = p->next;
p->next = node;
}
return head;
}
ListNode* deleteList(ListNode* head, int pos) {
if (pos <= 0) {
cout << "The pos is error!" << endl;
return head;
}
if (head == NULL || head->next == NULL) {
cout << "The list is empty!" << endl;
return head;
}
ListNode* p = head;
int index = 1;
while (p != NULL && index < pos) {
p = p->next;
index++;
}
if (p != NULL && p->next != NULL) {
ListNode* temp = p->next;
p->next = temp->next;
delete temp;
}
return head;
}
int main() {
ListNode* head = NULL;
head = createList();
printList(head);
insertList(head, 3, 300);
printList(head);
deleteList(head, 5);
printList(head);
cout << "The length of the list is " << getListLength(head) << endl;
system("pause");
return 0;
}
5. 运行结果截图如下:
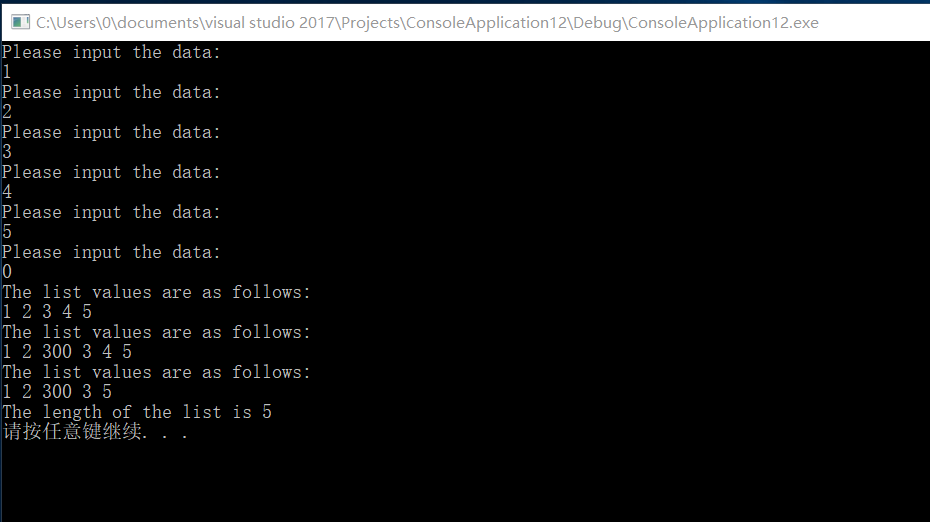