Springboot中-全局异常处理类用法示例
使用springboot搭建web项目的时候,一般都会添加一个全局异常类,用来统一处理各种自定义异常信息,
和其他非自定义的异常信息,以便于统一返回错误信息。下面就是简单的示例代码,
自定义异常信息.
public class MyException extends RuntimeException{
private String errorCode = ResultEnum.OTHER_EXCEPTION.getCode();
private String errorMessage ;
public String getErrorCode() {
return errorCode;
}
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public MyException() {
super();
}
public MyException(String errorCode, String... errorParam) {
super();
setErrorMessage(errorCode, errorParam);
}
private void setErrorMessage(String code, String... param) {
this.errorCode = code;
if (param == null) {
this.errorMessage = "系统异常";
} else if (param.length == 1) {
this.errorMessage = param[0];
} else if (param.length > 1) {
this.errorMessage = "系统异常";
}
}
}
全局异常信息处理类.
@Slf4j
@RestControllerAdvice
public class GlobalExceptionHandler {
/*
* 处理自定义异常
*/
@ExceptionHandler(value = MyException.class)
public @ResponseBody JsonResult exceptionHandler (MyException myException) {
log.info("exceptionHandler--->{}", myException);
JsonResult jsonResult = new JsonResult();
jsonResult.setErrorCode(myException.getErrorCode());
jsonResult.setErrorMessage(myException.getErrorMessage());
return jsonResult;
}
/*
* 处理参数校验异常-1
* */
@ExceptionHandler(value = MethodArgumentNotValidException.class)
public @ResponseBody JsonResult methodArgumentNotValidExceptionHandler (MethodArgumentNotValidException mANValidException) {
log.info("参数校验异常1!");
JsonResult jsonResult = new JsonResult();
jsonResult.setErrorCode(ResultEnum.PARAMS_ERROE.getCode());
// 获取所有的校验错误信息
List<ObjectError> allErrors = mANValidException.getBindingResult().getAllErrors();
StringBuilder sb = new StringBuilder();
// 处理错误的校验信息
if (allErrors != null && allErrors.size() > 1) {
allErrors.forEach(item->sb.append(item.getDefaultMessage()).append("#"));
} else {
allErrors.forEach(item->sb.append(item.getDefaultMessage()));
}
jsonResult.setErrorMessage(sb.toString());
return jsonResult;
}
/*
* 处理系统异常
* */
@ExceptionHandler(value = Exception.class)
public @ResponseBody JsonResult constraintViolationExceptionHandler (Exception exception) {
log.info("exception--->{}", exception);
JsonResult jsonResult = new JsonResult();
jsonResult.setErrorCode(ResultEnum.SYSTEM_EXCEPTION.getCode());
jsonResult.setErrorMessage(ResultEnum.SYSTEM_EXCEPTION.getMsg());
return jsonResult;
}
}
返回信息处理类.
@Data
public class JsonResult<T> implements Serializable {
private static final long serialVersionUID = -7866290240453139258L;
//返回时间
private String responseDate = null;
//返回状态
private String status = null;
//错误码
private String errorCode = null;
//错误信息
private String errorMessage = null;
//接口返回对象
private T responseBody = null;
public JsonResult() {
this.status = "SUCCESS";
this.responseDate = (new Date()).toString();
}
public JsonResult(T responseBody) {
this();
this.status = "SUCCESS";
this.responseBody = responseBody;
this.errorCode = "";
this.errorMessage = "";
}
public JsonResult<T> declareSuccess(T responseBody) {
this.status = "SUCCESS";
this.responseBody = responseBody;
this.errorCode = "";
this.errorMessage = "";
return this;
}
public JsonResult<T> declareFailure(MyException e, T responseBody) {
return declareFailure(e.getMessage(), e.getMessage(), responseBody);
}
public JsonResult<T> declareFailure(String errorCode, String errorMessage, T responseBody) {
this.status = "FAILURE";
this.errorCode = errorCode;
this.errorMessage = errorMessage;
this.responseBody = responseBody;
return this;
}
}
错误信息返回的枚举类.
public enum ResultEnum {
SUCCESS("1000", "成功"),
FAILED("1001", "失败"),
PARAMS_ERROE("1002", "参数错误"),
OTHER_EXCEPTION("1003", "其他错误"),
SYSTEM_EXCEPTION("0000", "系统异常"),
;
/*
* 返回码
*/
private String code;
/*
* 返回消息
*/
private String msg;
ResultEnum(String code, String msg) {
this.code = code;
this.msg = msg;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
测试的controller如下
@Slf4j
@RestController
public class TestControlelr {
@Autowired
private TestMapper testMapper;
@PostMapping("/exception/test")
public JsonResult exceptionTest(@Validated @RequestBody ParamTest paramTest){
TestPO testPO = new TestPO();
testPO.setNewTable("app_goods_info");
int total = testMapper.selectTotal(testPO);
System.out.println("total--->" + total);
JsonResult jsonResult = new JsonResult();
jsonResult.declareSuccess("异常测试接口:" + total);
return jsonResult;
}
@GetMapping("/exception/test/two")
public JsonResult exceptionTestTwo(){
int total = 10;
System.out.println("total--->" + total);
int temp = 1 / 0;
JsonResult jsonResult = new JsonResult();
jsonResult.declareSuccess("异常测试接口:" + total);
return jsonResult;
}
@PostMapping("/exception/test/three")
public JsonResult exceptionTestThree(){
int total = 10;
System.out.println("total--->" + total);
if (total > 8) {
throw new MyException(ResultEnum.FAILED.getCode(), "测试自定义异常");
}
JsonResult jsonResult = new JsonResult();
jsonResult.declareSuccess("异常测试接口:" + total);
return jsonResult;
}
}
测试的入参类如下:
@Data
public class ParamTest {
/*
* 名称
*/
@NotNull(message = "名称不能为null")
private String name;
/*
* 简介
*/
@Length(max = 10, message = "简介长度最大为10")
private String abs;
}
测试一:填写错误的参数,返回信息如下。
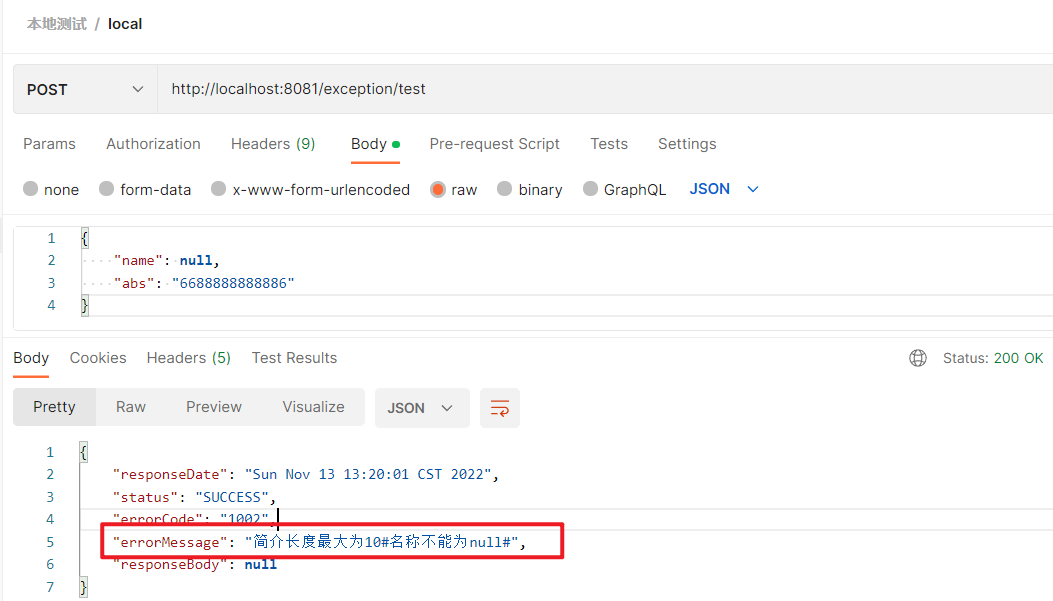
测试二:测试在代码中写1/0的返回结果。
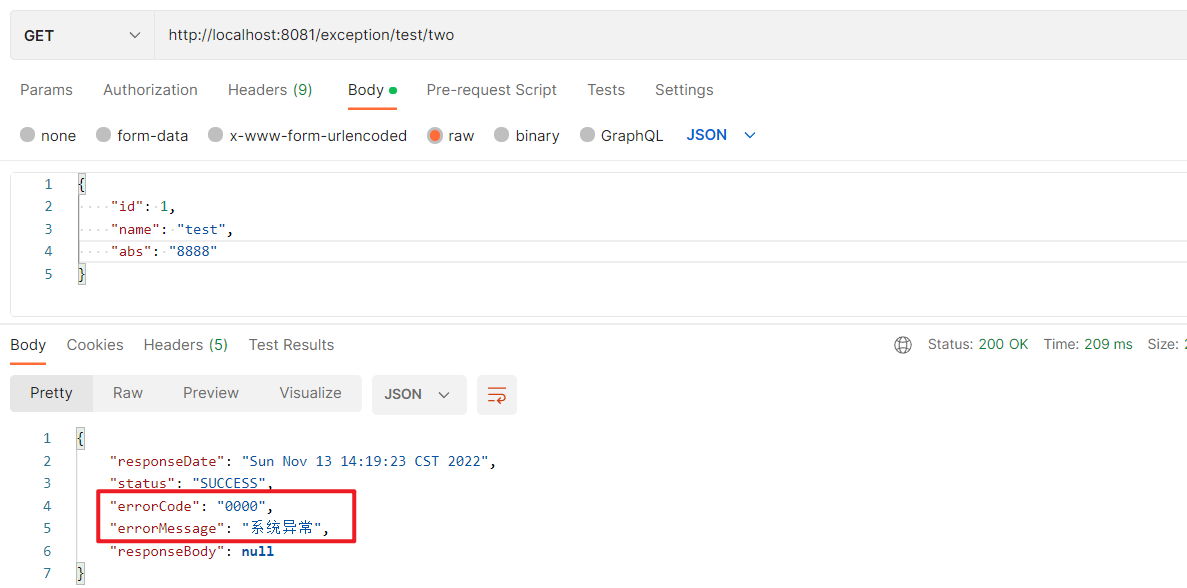
测试三:主动抛出一个自定义异常,返回结果如下。
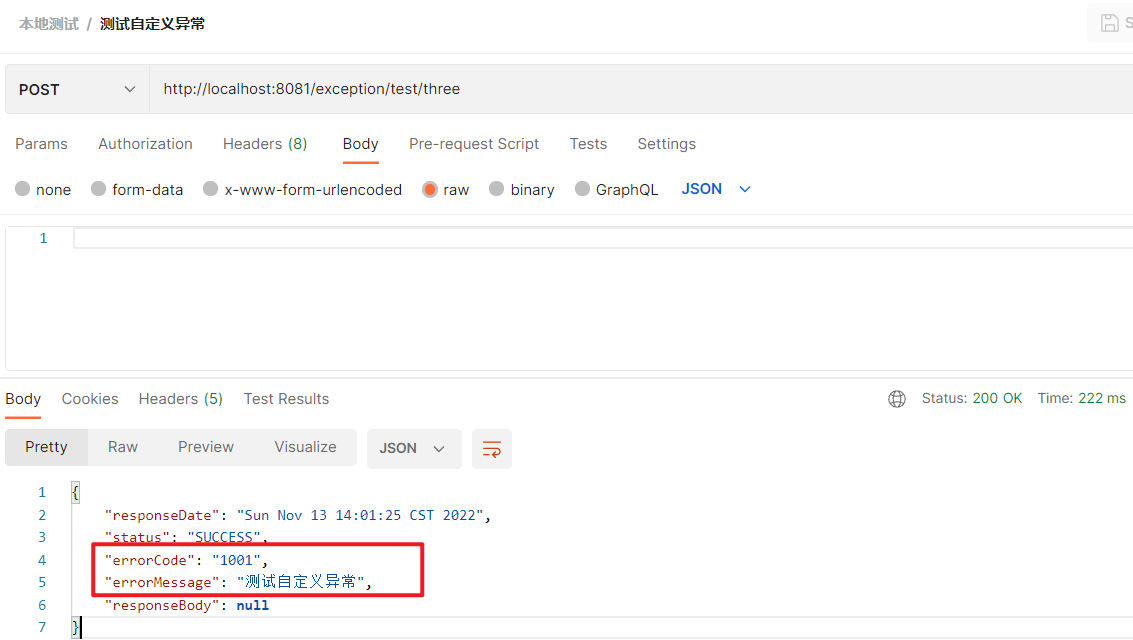
在全局异常处理类中添加了三个方法,一个方法用来处理自定义异常的错误返回信息,一个方法用法用来返回参数校验的错误返回信息,
最后一个方法用来处理系统异常,三个方法都可以正常执行。真实开发中可以根据实际需要去添加一些自定义
异常信息,根据需要使用全局异常类来进行统一处理,这样可以很方便的从错误信息中分辨出是什么地方出现问题,便于快速排查问题。