python 操作数据库
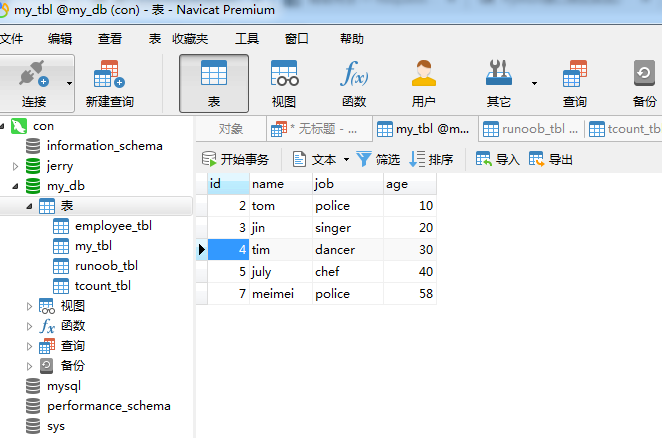
from pymysql import connect, cursors from pymysql.err import OperationalError class DB: def __init__(self): try: self.connection = connect(host=host, port=int(3306), db = db, user = user, password = passwd, charset = 'utf8', cursorclass=cursors.DictCursor) except OperationalError as e: print("Mysql Error %d: %s" % (e.args[0], e.args[1])) self.cur = self.connection.cursor() def insert(self, table_name, data): for key in data: data[key] = "'"+str(data[key])+"'" key = ','.join(data.keys()) value = ','.join(data.values()) sql = "INSERT INTO " + table_name + " (" + key + ") VALUES\ (" + value + ")" self.cur.execute(sql) self.connection.commit() def clear(self,table_name): sql = "delete from " + table_name + ";" self.cur.execute("SET FOREIGN_KEY_CHECKS=0;") self.cur.execute(sql) self.connection.commit() def query(self,sql): self.cur.execute(sql) return self.cur.fetchall() def check_data(self,table_name,name): sql="select * from {} where name='{}'".format(table_name,name) res=DB().query(sql) return True if res else False def delete(self,tabel_name,name): sql="delete from {} where name='{}'".format(table_name,id) self.cur.execute(sql) def close(self): self.connection.close() if __name__=="__main__": db=DB() table_name="my_tbl" name="jin" print(db.check_data(table_name,name))
2、读取配置文件:db_config.ini 与readConfig.py 同一级目录
[mysqlconf] host=**** port=*** user=root password=**** db_name=my_db
import configparser import os class ReadConfig: """定义一个读取配置文件的类""" def __init__(self, filepath=None): if filepath: configpath = filepath else: base_path = os.path.dirname(__file__) configpath = base_path + "/db_config.ini" self.cf = configparser.ConfigParser() self.cf.read(configpath) def get_db(self, param): value = self.cf.get("mysqlconf", param) return value if __name__ == '__main__': test = ReadConfig() t = test.get_db("host") print(t)