java学习笔记 多线程操作的相关方法
一、判断线程是否启动
isAlive()方法来确定一个线程是否启动
主线程(main)有可能先执行完,此时分线程不受影响
二、线程强制运行
join()方法可以让一个线程强制运行,在此期间,其他线程无法运行,必须等此线程执行完毕才能继续运行
public class Test { public static void main(String[] args) { MyThread mt1 = new MyThread(); Thread thread = new Thread(mt1,"t1"); System.out.println(thread.isAlive() + "+++++++++++++++++++++++++");//false thread.start(); System.out.println(thread.isAlive() + "+++++++++++++++++++++++++");//true for(int i = 1; i <= 10; i++) { if (i == 5) { try { thread.join();//强制运行,直到结束,其他线程才能运行 }catch(InterruptedException e) { e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + "运行" + i); } System.out.println(thread.isAlive() + "+++++++++++++++++++++++++"); } } class MyThread implements Runnable { public void run() { for(int i = 1; i <= 10; i++) { System.out.println(Thread.currentThread().getName() + "运行" + i); } } }
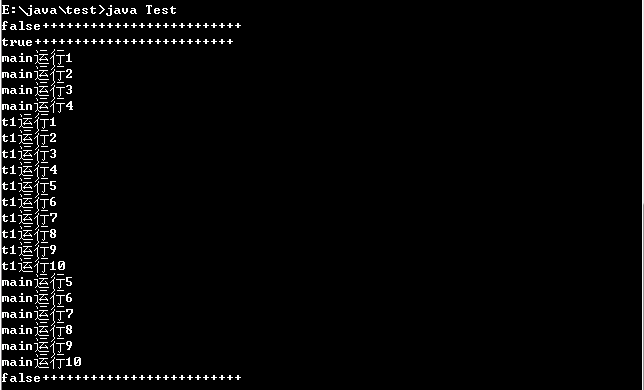
三、线程的休眠
在程序中允许线程暂时性休眠
静态方法 Thread.sleep()方法
四、当一个线程运行时,可以通过interrupter()中断其运行状态
interrupter()方法
一个线程使用sleep方法休眠后,用interrupt()方法打断,执行的是sleep后异常的代码
public class Test { public static void main(String[] args) { MyThread mt1 = new MyThread(); Thread thread = new Thread(mt1,"t1"); System.out.println(thread.isAlive() + "+++++++++++++++++++++++++"); thread.start(); try{ Thread.sleep(100);//为了确保thread方法已经在休眠 }catch(InterruptedException e) { System.out.println(Thread.currentThread().getName() + "睡眠中被打断"); } thread.interrupt(); } } class MyThread implements Runnable { public void run() { for(int i = 1; i <= 10; i++) { try { Thread.sleep(200);//静态方法,直接调用 }catch(InterruptedException e) { System.out.println(Thread.currentThread().getName() + "睡眠中被打断"); } System.out.println(Thread.currentThread().getName() + "运行" + i); } } }

五、线程的优先级
线程的优先级决定哪个线程会先执行,但并非线程的优先级越高就一定会先执行,哪个线程先执行是由CPU调度决定
方法:setPriority()
public static final int MIN_PRIORITY 常量1
public static final int NORM_PRIORITY 常量5
public static final int MAX_PRIORITY 常量5
六、线程礼让
yield()方法
在线程操作中,可以将一个线程的操作暂时让给其他线程执行
public class Test { public static void main(String[] args) { MyThread mt1 = new MyThread(); Thread t1 = new Thread(mt1,"t1"); Thread t2 = new Thread(mt1,"t2"); Thread t3 = new Thread(mt1,"t3"); t1.setPriority(Thread.MAX_PRIORITY); t1.setPriority(Thread.NORM_PRIORITY); t1.setPriority(1); t1.start(); t2.start(); t3.start(); } } class MyThread implements Runnable { public void run() { for (int i = 1;i <= 5;i++) { System.out.println(Thread.currentThread().getName() + "运行" + i); if( i == 3) { System.out.print(Thread.currentThread().getName() + "线程礼让: "); Thread.currentThread().yield(); } } } } /* 1.并不是线程的优先等级高就一定先执行,具体看CPU调度 */
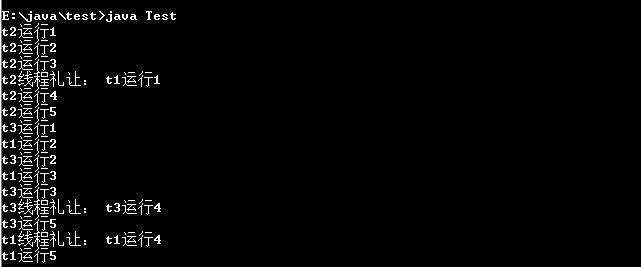