实验4
1、车辆类的实现
battery.h
#ifndef BATTERY_H
#define BATTERY_H
class Battery
{
public:
Battery(int a=70);
int getbatterysize();
private:
int batterysize;
};
#endif
battery.cpp
#include"battery.h"
#include<iostream>
Battery::Battery(int a=70):batterysize(a){};
int Battery::getbatterysize(){
return batterysize;
}
#include<iostream>
Battery::Battery(int a=70):batterysize(a){};
int Battery::getbatterysize(){
return batterysize;
}
car.h
#ifndef CAR_H
#define CAR_H
#include<iostream>
using std::string;
using std::ostream;
class Car{
public:
Car(string a,string b,int c,double d=0){};
friend ostream& operator<<(ostream &out,const Car &c);
void updateOdometer(double e);
string getmaker() const;
string getmodel() const;
int getyear() const;
double getodometer() const;
private:
string maker;
string model;
int year;
double odometer;
};
#endif
#define CAR_H
#include<iostream>
using std::string;
using std::ostream;
class Car{
public:
Car(string a,string b,int c,double d=0){};
friend ostream& operator<<(ostream &out,const Car &c);
void updateOdometer(double e);
string getmaker() const;
string getmodel() const;
int getyear() const;
double getodometer() const;
private:
string maker;
string model;
int year;
double odometer;
};
#endif
car.cpp
#include"car.h"
#include<iostream>
#include<string>
using namespace std;
Car::Car(string a,string b,int c,double d=0){
maker=a;
model=b;
year=c;
odometer=d;
}
ostream &operator<<(ostream &out,const Car &c){
out<<"maker:"<<c.maker<<endl<<"model:"<<c.model<<endl<<"year:"<<c.year<<endl<<"odometer:"<<c.odometer<<endl;
return out;
}
void Car::updateOdometer(double e){
if(e>=odometer)
odometer=e;
else
cout<<"wrong"<<endl;
}
string Car::getmaker() const
{
return maker;
}
#include<iostream>
#include<string>
using namespace std;
Car::Car(string a,string b,int c,double d=0){
maker=a;
model=b;
year=c;
odometer=d;
}
ostream &operator<<(ostream &out,const Car &c){
out<<"maker:"<<c.maker<<endl<<"model:"<<c.model<<endl<<"year:"<<c.year<<endl<<"odometer:"<<c.odometer<<endl;
return out;
}
void Car::updateOdometer(double e){
if(e>=odometer)
odometer=e;
else
cout<<"wrong"<<endl;
}
string Car::getmaker() const
{
return maker;
}
string Car::getmodel() const
{
return model;
}
{
return model;
}
int Car::getyear() const
{
return year;
}
{
return year;
}
double Car::getodometer() const
{
return odometer;
}
{
return odometer;
}
electriccar.h
#ifndef ELECTRICCAR_H
#define ELECTRICCAR_H
#include"car.h"
#include"battery.h"
#include<iostream>
using std::string;
using std::ostream;
class ElectricCar:public Car,public Battery{
public:
ElectricCar(string a,string b,int c,double d=0,int e=70){};
friend ostream& operator<<(ostream &out,const Car &c);
private:
Battery battery;
};
#endif
#define ELECTRICCAR_H
#include"car.h"
#include"battery.h"
#include<iostream>
using std::string;
using std::ostream;
class ElectricCar:public Car,public Battery{
public:
ElectricCar(string a,string b,int c,double d=0,int e=70){};
friend ostream& operator<<(ostream &out,const Car &c);
private:
Battery battery;
};
#endif
electriccar.cpp
#include"electricCar.h"
#include<iostream>
#include"battery.h"
#include"car.h"
using namespace std;
ostream &operator<<(ostream &out,const ElectricCar &c){
out<<"maker:"<<c.getmaker<<endl<<"model:"<<c.getmodel<<endl<<"year:"<<c.getyear<<endl<<"odometer:"<<c.getodometer<<endl<<"battery:"<<c.getbatterysize<<endl;
return out;
}
ElectricCar::ElectricCar(string a, string b, int c, double d = 0,int e) :Car(a, b, c, d),battery(e) {};
#include<iostream>
#include"battery.h"
#include"car.h"
using namespace std;
ostream &operator<<(ostream &out,const ElectricCar &c){
out<<"maker:"<<c.getmaker<<endl<<"model:"<<c.getmodel<<endl<<"year:"<<c.getyear<<endl<<"odometer:"<<c.getodometer<<endl<<"battery:"<<c.getbatterysize<<endl;
return out;
}
ElectricCar::ElectricCar(string a, string b, int c, double d = 0,int e) :Car(a, b, c, d),battery(e) {};
main.cpp
#include <iostream>
using namespace std;
#include"car.h"
#include"electricCar.h"
using namespace std;
#include"car.h"
#include"electricCar.h"
int main() {
// 测试Car类
Car oldcar("Audi","a4",2016);
cout << "--------oldcar's info--------" << endl;
oldcar.updateOdometer(25000);
cout << oldcar << endl;
// 测试Car类
Car oldcar("Audi","a4",2016);
cout << "--------oldcar's info--------" << endl;
oldcar.updateOdometer(25000);
cout << oldcar << endl;
// 测试ElectricCar类
ElectricCar newcar("Tesla","model s",2016);
newcar.updateOdometer(2500);
cout << "\n--------newcar's info--------\n";
cout << newcar << endl;
ElectricCar newcar("Tesla","model s",2016);
newcar.updateOdometer(2500);
cout << "\n--------newcar's info--------\n";
cout << newcar << endl;
system("pause");
return 0;
}
return 0;
}
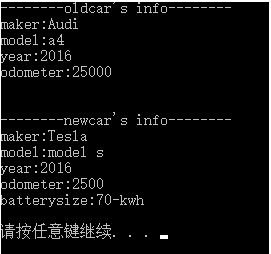
2、重载【】
arrayint.h
#ifndef ARRAY_INT_H
#define ARRAY_INT_H
#define ARRAY_INT_H
class ArrayInt{
public:
ArrayInt(int n, int value=0);
~ArrayInt();
int &operator[](int i);
void print();
private:
int *p;
int size;
};
public:
ArrayInt(int n, int value=0);
~ArrayInt();
int &operator[](int i);
void print();
private:
int *p;
int size;
};
#endif
arrayint.cpp
#include "arrayInt.h"
#include <iostream>
#include <cstdlib>
using std::cout;
using std::endl;
#include <iostream>
#include <cstdlib>
using std::cout;
using std::endl;
ArrayInt::ArrayInt(int n, int value): size(n) {
p = new int[size];
if (p == nullptr) {
cout << "fail to mallocate memory" << endl;
exit(0);
}
for(int i=0; i<size; i++)
p[i] = value;
}
p = new int[size];
if (p == nullptr) {
cout << "fail to mallocate memory" << endl;
exit(0);
}
for(int i=0; i<size; i++)
p[i] = value;
}
ArrayInt::~ArrayInt() {
delete[] p;
}
delete[] p;
}
void ArrayInt::print() {
for(int i=0; i<size; i++)
cout << p[i] << " ";
cout << endl;
}
for(int i=0; i<size; i++)
cout << p[i] << " ";
cout << endl;
}
int &ArrayInt::operator[](int i) {
return p[i];
}
return p[i];
}
main.cpp
#include <iostream>
using namespace std;
using namespace std;
#include "arrayInt.h"
int main() {
// 定义动态整型数组对象a,包含2个元素,初始值为0
ArrayInt a(2);
a.print();
// 定义动态整型数组对象b,包含3个元素,初始值为6
ArrayInt b(3, 6);
b.print();
// 定义动态整型数组对象a,包含2个元素,初始值为0
ArrayInt a(2);
a.print();
// 定义动态整型数组对象b,包含3个元素,初始值为6
ArrayInt b(3, 6);
b.print();
// 通过对象名和下标方式访问并修改对象元素
b[0] = 2;
cout << b[0] << endl;
b.print();
b[0] = 2;
cout << b[0] << endl;
b.print();
system("pause");
return 0;
}
}
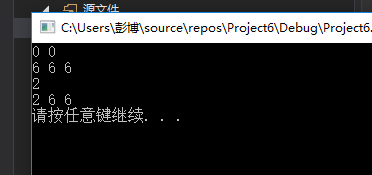
实验总结:第一个车辆类仍有许多未曾完善的地方,写的时候也花了很多不必要的时间。
第二个动态数组类理解起来更为容易,想较于以前的数组定义更简单明了。
总之还是需要多加强对运算符重载的练习以及对类的感觉。