题目
创建一个长度可由用户输入的数组,存入10-99不重复的数,要求用户输入在另外一个线程实现,并按升序输出;
代码
Thread1类,负责输入
public class Thread1 implements Runnable {
@Override
public void run() {
Scanner scanner = new Scanner(System.in);
while (true) {
Test.num = scanner.nextInt();
if (Test.num >= 0 && Test.num <= 90) {
break;
} else {
System.out.println("您输入的数据有误,请输入0-90之间的数据:");
Test.num = scanner.nextInt();
}
}
}
}
Test类:
import java.util.Random;
public class Test {
static int num = 0;
public static void main(String[] args) throws InterruptedException {
System.out.println("请输入数组长度,最大值为90:");
//创建线程
Thread1 t = new Thread1();
Thread t1 = new Thread(t);
t1.start();
//等待用户输入正确的元素个数,如果未输入正确的数,则一直休眠主线程,避免主线程继续执行下去导致数组异常
while(true) {
if (t1.isAlive())
Thread.sleep(100);
else
break;
}
//定义数组
int[] array = new int[Test.num+1];
//存入数据
Random random = new Random();
int temp = 0;
array[1] = random.nextInt(90) + 10;
for (int i = 2; i <= Test.num; i++) {
for (int j = 1; j < i; j++) {
//判断随机数是否重复、是否为两位数
if (temp == array[j] || temp < 10 || temp > 99) {
j = 1;
temp = random.nextInt(90) + 10;
}
}
array[i] = temp;
}
System.out.println("排序前的数组元素:");
for (int i = 1; i <= Test.num; i++) {
if (i % 10 == 0 || i == Test.num)
System.out.println(array[i]);
else
System.out.print(array[i] + " ");
}
//直接插入法排序
for (int i = 2 ; i <= Test.num; i++) {
if (array[i] < array[i-1]){
array[0] = array[i];
for (int j = i-1 ; array[0] < array[j]; j--) {
array[j+1] = array[j];
array[j] = array[0];
}
}
}
System.out.println("排序后的数组元素:");
for (int i = 1; i <= Test.num; i++) {
if (i % 10 == 0)
System.out.println(array[i]);
else
System.out.print(array[i] + " ");
}
}
}
结果
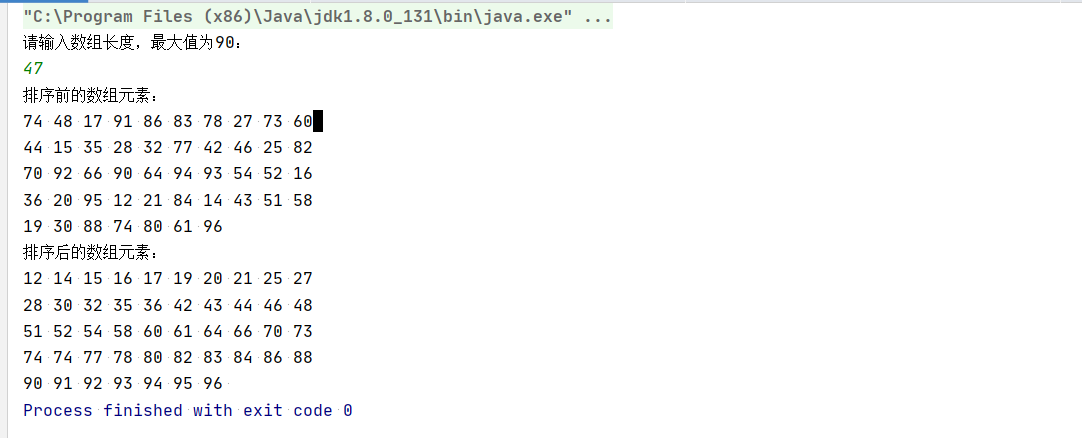
实现这个功能并不需要多线程,只是因为刚刚学习多线程,所以加进去练练手,可忽略。