【第2节 React面向组件编程】组件的三大属性:state、props、refs
组件三大属性
1、state:状态
//1、定义组件
class Like extends React.Component {
constructor(props) {
super(props);
//初始化状态
this.state = {
isLikeMe: false
};
//将handleClick()中的this强制绑定为组件对象
this.handleClick = this.handleClick.bind(this)
}
//更新状态(新添加的方法,内部的this不是组件对象,而是undefined)
handleClick() {
const isLikeMe = !this.state.isLikeMe;
this.setState({isLikeMe})
//ES6语法,相同名字可省略,也可写成:this.setState({isLikeMe:isLkeMe})
}
//更新状态,第二种写法,不需要绑定this了
handleClick = ()=>{
}
//(重写组件的方法)
render() {
//读取状态
const {isLikeMe} = this.state;//ES6的解构赋值,也可写成:const isLikeMe =this.state.isLikeMe
return <h2 onClick={this.handleClick}>{isLikeMe ? '你喜欢我' : '我喜欢你'}</h2>
}
}
//2、渲染组件标签
ReactDOM.render(<Like/>, document.getElementById("test1"))
2、props:属性
//1、定义组件
function Person(props) {
return (
<ul>
<li>姓名:{props.name}</li>
<li>年龄:{props.age}</li>
<li>性别:{props.sex}</li>
</ul>
)
}
//2、渲染组件标签
const p1 = {
name: 'Tom',
age: 12,
sex: '男'
};
ReactDOM.render(<Person name={p1.name} age={p1.age} sex={p1.sex}/>, document.getElementById("test1"))
//ReactDOM.render(<Person {...p1} />,document.getElementById("test1"))//扩展属性:将对象的所有属性通过props传递
//指定属性默认值
Person.defaultProps = {
sex: 'nan',
age: 18
};
//指定属性的类型限制和必要性限制
Person.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number,
};
const p2 = {
name: 'Jack',
};
ReactDOM.render(<Person name={p2.name}/>, document.getElementById("test2"))
3、refs
//1、定义组件
class MyComponent extends React.Component {
constructor(props, context) {
super(props, context);
this.showInput = this.showInput.bind(this);
this.handleBlur = this.handleBlur.bind(this)
}
showInput() {
//const input=this.refs.content;
//alert(input.value);
alert((this.input.value))
}
handleBlur(event) {
alert(event.target.value)
}
render() {
return (
<div>
<input type="text" ref="content"/>
<input type="text" ref={input => this.input = input}/>
<button onClick={this.showInput}>提示输入</button>
<input type="text" placeholder="失去焦点提示内容" onBlur={this.handleBlur}/>
</div>
)
}
}
//2、渲染组件标签
ReactDOM.render(<MyComponent/>, document.getElementById("test1"))
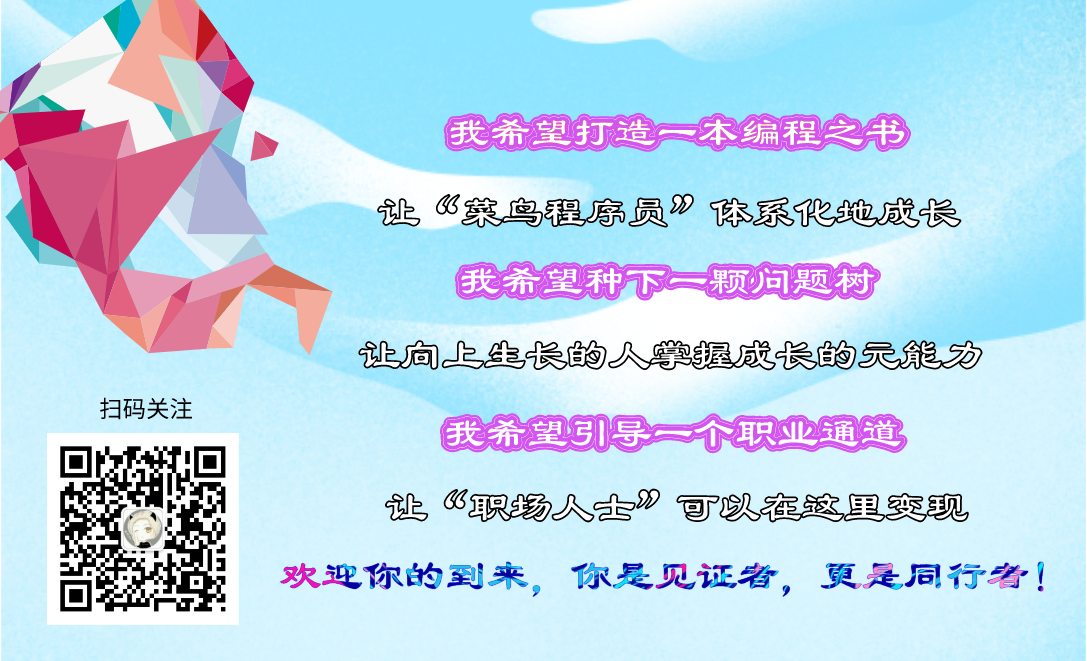
作者:-亚州Asu-
若标题中有“转载”字样,则本文版权归原作者所有。若无转载字样,本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。
tips:你的点赞我都当成了喜欢~