112. 路径总和
题目来源:112. 路径总和
给你二叉树的根节点 root
和一个表示目标和的整数 targetSum
,判断该树中是否存在 根节点到叶子节点 的路径,这条路径上所有节点值相加等于目标和 targetSum
。
叶子节点 是指没有子节点的节点。
示例 1:
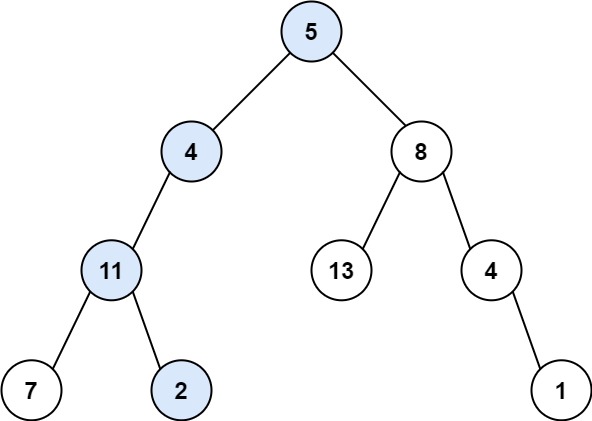
输入:root = [5,4,8,11,null,13,4,7,2,null,null,null,1], targetSum = 22 输出:true
示例 2:
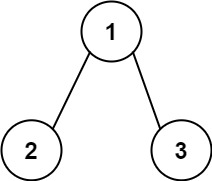
输入:root = [1,2,3], targetSum = 5 输出:false
示例 3:
输入:root = [1,2], targetSum = 0 输出:false
递归
/** * Definition for a binary tree node. * function TreeNode(val, left, right) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefined ? null : right) * } */ /** * @param {TreeNode} root * @param {number} targetSum * @return {boolean} */ var hasPathSum = function(root, targetSum) { if(!root){ return false } const sum = targetSum - root.val if(!root.left && !root.right){ return sum === 0 } return hasPathSum(root.left, sum) || hasPathSum(root.right, sum) };
广度优先
/** * Definition for a binary tree node. * function TreeNode(val, left, right) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefined ? null : right) * } */ /** * @param {TreeNode} root * @param {number} targetSum * @return {boolean} */ var hasPathSum = function(root, targetSum) { if(!root){ return false } var stack = [] var val = [] stack.push([root]) val.push([targetSum]) while(stack.length){ let level = stack.shift() let levelVal = val.shift() let len = level.length let stackTmp = [] let valTmp = [] for(let i = 0;i < len; i++){ let node = level[i] if(!node){ continue } let nodeVal = levelVal[i] if(!node.left && !node.right){ if((nodeVal - node.val) == 0 ){ return true; } continue } if(node.left){ stackTmp.push(node.left) valTmp.push(nodeVal - node.val) } if(node.right){ stackTmp.push(node.right) valTmp.push(nodeVal - node.val) } } if(stackTmp.length)stack.push(stackTmp) if(valTmp.length)val.push(valTmp) } return false };
Python3
# Definition for a binary tree node. # class TreeNode: # def __init__(self, val=0, left=None, right=None): # self.val = val # self.left = left # self.right = right class Solution: def hasPathSum(self, root: TreeNode, targetSum: int) -> bool: if not root: return False sum = targetSum - root.val if not root.left and not root.right: return sum == 0 left = self.hasPathSum(root.left , sum) right = self.hasPathSum(root.right, sum) return left or right
广度优先
class Solution: def hasPathSum(self, root: TreeNode, targetSum: int) -> bool: if not root: return False stack = list() stack.append([(targetSum, root)]) while stack: level = stack.pop(0) tmp = list() for node in level: if not node[1]: continue if not node[1].left and not node[1].right: if node[0] == node[1].val: return True if node[1].left: tmp.append((node[0] - node[1].val, node[1].left)) if node[1].right: tmp.append((node[0] - node[1].val, node[1].right)) if tmp: stack.append(tmp) return False
提示:
- 树中节点的数目在范围
[0, 5000]
内 -1000 <= Node.val <= 1000
-1000 <= targetSum <= 1000