83. 删除排序链表中的重复元素
题目来源:83. 删除排序链表中的重复元素
存在一个按升序排列的链表,给你这个链表的头节点 head
,请你删除所有重复的元素,使每个元素 只出现一次 。
返回同样按升序排列的结果链表。
方法一:一次遍历
/** * Definition for singly-linked list. * function ListNode(val, next) { * this.val = (val===undefined ? 0 : val) * this.next = (next===undefined ? null : next) * } */ /** * @param {ListNode} head * @return {ListNode} */ var deleteDuplicates = function(head) { if(!head){ return head; } let cur = head; while(cur.next){ if(cur.val === cur.next.val){ cur.next = cur.next.next; }else{ cur = cur.next; } } return head; };
方法二:哈希
/** * Definition for singly-linked list. * function ListNode(val, next) { * this.val = (val===undefined ? 0 : val) * this.next = (next===undefined ? null : next) * } */ /** * @param {ListNode} head * @return {ListNode} */ var deleteDuplicates = function(head) { let cur = head; let pre = cur; let hash = new Map(); while(cur){ let val = cur.val; if(!hash.has(val)){ hash.set(val, 1); pre = cur; }else{ pre.next = cur.next; } cur = cur.next; } return head; };
示例 1:
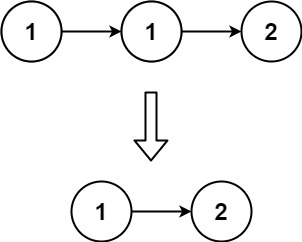
输入:head = [1,1,2] 输出:[1,2]
示例 2:
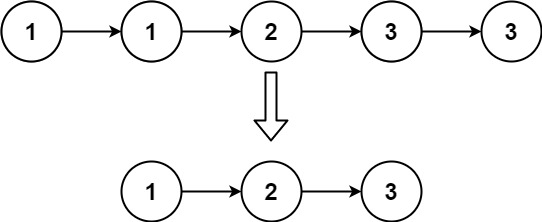
输入:head = [1,1,2,3,3] 输出:[1,2,3]
python3
# Definition for singly-linked list. class ListNode: def __init__(self, val=0, next=None): self.val = val self.next = next class Solution: def deleteDuplicates(self, head: ListNode) -> ListNode: if not head: return head cur = head while cur: if cur.val == cur.next.val: cur.next = cur.next.next else: cur = cur.next return head
提示:
- 链表中节点数目在范围
[0, 300]
内 -100 <= Node.val <= 100
- 题目数据保证链表已经按升序排列