合成模式中有可能存在二个对象,Composite对象和Leaf对象,Composite对象中能包含Composite对象和Leaf对象,但是Leaf却只能独立存在。在运用合成模式进行建模时候,必须要掌握两个思想,一,在设计对象组的时候,既应该可以包容单个的基本对象,也可以包容其它的对象组。二、单个的基本对象和对象组都应该定义公共的行为,并将这个对象组描述为包容了这个类型的对象的集合。
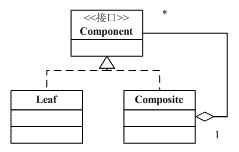
在合成模式的原型图中,涉及到三个角色:
1、抽象构件(Component),这是给出的一个公共接口,规定了参与组合的对象的默认行为。
2、合成构件(Composite),参加组合的有子对象的对象,并给出了合成构件的行为。
3、基本构件(Leaf),参加组合的没有子对象的对象。
合成模式根据所实现的接口的不同。分为了安全模式和透明模式,
安全模式是指在Composite构件中实现管理子类对象的方法,如Add,Remove,GetChild等方法,这样在Leaf中就无法实现这些方法,即使有实现也不能通过编译,这样就达到了代码的安全。不够透明,Composite和Leaf对象使用不同的接口
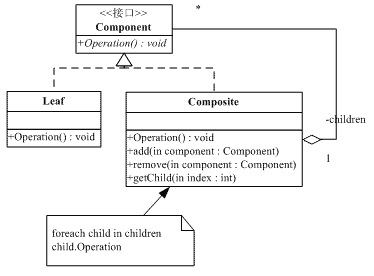
透明模式,是指在接口中给出所有管理子类的方法,在Composite和Leaf中都能实现这些方法,但是如果在Leaf类中不正确的实现了这些方法,将不能在编译中被检查出来,而在运行时报错。

以上全部是借的吕老师的代码,下面的就是我自己写的了。两点了,也来不及测试了。明天再看看。
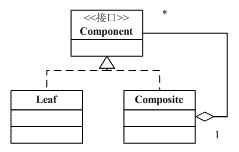
在合成模式的原型图中,涉及到三个角色:
1、抽象构件(Component),这是给出的一个公共接口,规定了参与组合的对象的默认行为。
2、合成构件(Composite),参加组合的有子对象的对象,并给出了合成构件的行为。
3、基本构件(Leaf),参加组合的没有子对象的对象。
合成模式根据所实现的接口的不同。分为了安全模式和透明模式,
安全模式是指在Composite构件中实现管理子类对象的方法,如Add,Remove,GetChild等方法,这样在Leaf中就无法实现这些方法,即使有实现也不能通过编译,这样就达到了代码的安全。不够透明,Composite和Leaf对象使用不同的接口
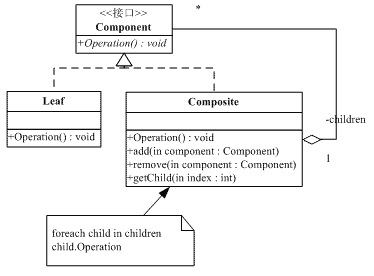
1
using System;
2
using System.Text;
3
using System.Collections;
4
5
// "Component"
6
abstract class Component
7
{
8
// Fields
9
protected string name;
10
11
// Constructors
12
public Component( string name )
13
{
14
this.name = name;
15
}
16
17
// Operation
18
public abstract void Display( int depth );
19
}
20
21
// "Composite"
22
class Composite : Component
23
{
24
// Fields
25
private ArrayList children = new ArrayList();
26
27
// Constructors
28
public Composite( string name ) : base( name ) {}
29
30
// Methods
31
public void Add( Component component )
32
{
33
children.Add( component );
34
}
35
public void Remove( Component component )
36
{
37
children.Remove( component );
38
}
39
public override void Display( int depth )
40
{
41
Console.WriteLine( new String( '-', depth ) + name );
42
43
// Display each of the node's children
44
foreach( Component component in children )
45
component.Display( depth + 2 );
46
}
47
}
48
49
// "Leaf"
50
class Leaf : Component
51
{
52
// Constructors
53
public Leaf( string name ) : base( name ) {}
54
55
// Methods
56
public override void Display( int depth )
57
{
58
Console.WriteLine( new String( '-', depth ) + name );
59
}
60
}
61
62
/**//// <summary>
63
/// Client test
64
/// </summary>
65
public class Client
66
{
67
public static void Main( string[] args )
68
{
69
// Create a tree structure
70
Composite root = new Composite( "root" );
71
root.Add( new Leaf( "Leaf A" ));
72
root.Add( new Leaf( "Leaf B" ));
73
Composite comp = new Composite( "Composite X" );
74
75
comp.Add( new Leaf( "Leaf XA" ) );
76
comp.Add( new Leaf( "Leaf XB" ) );
77
root.Add( comp );
78
79
root.Add( new Leaf( "Leaf C" ));
80
81
// Add and remove a leaf
82
Leaf l = new Leaf( "Leaf D" );
83
root.Add( l );
84
root.Remove( l );
85
86
// Recursively display nodes
87
root.Display( 1 );
88
}
89
}

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

透明模式,是指在接口中给出所有管理子类的方法,在Composite和Leaf中都能实现这些方法,但是如果在Leaf类中不正确的实现了这些方法,将不能在编译中被检查出来,而在运行时报错。

1
using System;
2
using System.Text;
3
using System.Collections;
4
5
// "Component"
6
abstract class Component
7
{
8
// Fields
9
protected string name;
10
11
// Constructors
12
public Component( string name )
13
{ this.name = name; }
14
15
// Methods
16
abstract public void Add(Component c);
17
abstract public void Remove( Component c );
18
abstract public void Display( int depth );
19
}
20
21
// "Composite"
22
class Composite : Component
23
{
24
// Fields
25
private ArrayList children = new ArrayList();
26
27
// Constructors
28
public Composite( string name ) : base( name ) {}
29
30
// Methods
31
public override void Add( Component component )
32
{ children.Add( component ); }
33
34
public override void Remove( Component component )
35
{ children.Remove( component ); }
36
37
public override void Display( int depth )
38
{
39
Console.WriteLine( new String( '-', depth ) + name );
40
41
// Display each of the node's children
42
foreach( Component component in children )
43
component.Display( depth + 2 );
44
}
45
}
46
47
// "Leaf"
48
class Leaf : Component
49
{
50
// Constructors
51
public Leaf( string name ) : base( name ) {}
52
53
// Methods
54
public override void Add( Component c )
55
{ Console.WriteLine("Cannot add to a leaf"); }
56
57
public override void Remove( Component c )
58
{ Console.WriteLine("Cannot remove from a leaf"); }
59
60
public override void Display( int depth )
61
{ Console.WriteLine( new String( '-', depth ) + name ); }
62
}
63
64
/**//// <summary>
65
/// Client test
66
/// </summary>
67
public class Client
68
{
69
public static void Main( string[] args )
70
{
71
// Create a tree structure
72
Composite root = new Composite( "root" );
73
root.Add( new Leaf( "Leaf A" ));
74
root.Add( new Leaf( "Leaf B" ));
75
Composite comp = new Composite( "Composite X" );
76
77
comp.Add( new Leaf( "Leaf XA" ) );
78
comp.Add( new Leaf( "Leaf XB" ) );
79
root.Add( comp );
80
81
root.Add( new Leaf( "Leaf C" ));
82
83
// Add and remove a leaf
84
Leaf l = new Leaf( "Leaf D" );
85
root.Add( l );
86
root.Remove( l );
87
88
// Recursively display nodes
89
root.Display( 1 );
90
}
91
}

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

以上全部是借的吕老师的代码,下面的就是我自己写的了。两点了,也来不及测试了。明天再看看。
1
using System;
2
using Systme.Collection;
3
4
abstract class Component
5
{
6
abstract public void AddMatchine
7
abstract public int GetMatchineCount();
8
}
9
10
class Composite:Component
11
{
12
ArrayList List=new ArrayList();
13
public override void AddMatchine(Component c)
14
{
15
List.Add(c);
16
}
17
public override int GetMatchineCount()
18
{
19
int count=0;
20
foreach(Component o in List)
21
{
22
Count+= o.GetMatchineCount();
23
}
24
}
25
}
26
27
class MatchineComponent:Component
28
{
29
public override int GetMatchineCount()
30
{
31
return 1;
32
}
33
}
34

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34
