python网络编程之websocket
服务端:
import asyncio import websockets # 保存已连接的客户端列表 connected_clients = set() async def handle_websocket(websocket): # 将新的客户端添加到已连接客户端列表 remote_address = websocket.remote_address connected_clients.add(websocket) print(f"新的客户端已连接:{remote_address}") try: # 接收客户端消息并发送回复 async for message in websocket: print(f"收到客户端{remote_address}消息:{message}") # 广播发送消息给所有客户端 await broadcast(message) except websockets.ConnectionClosedError: print(f"客户端{remote_address}连接关闭") except Exception as e: print(f"处理客户端时出现错误:{e}") finally: # 从已连接客户端列表中移除客户端 connected_clients.remove(websocket) async def broadcast(message): # 遍历所有已连接的客户端,并发送消息 for client in connected_clients: await client.send(message) async def main(): # 创建WebSocket服务器并注册处理程序 server = await websockets.serve(handle_websocket, "127.0.0.1", 8888) # 运行服务器 await server.wait_closed() # 运行主函数 asyncio.run(main()) #https://blog.csdn.net/yuanfeidexin321/article/details/131482280
客户端:
import websocket def on_message(ws, message): print(f"收到服务端回复:{message}") def on_error(ws, error): print(f"Error: {error}") def on_close(ws): print("Connection closed") def on_open(ws): print("Connection opened") ws.send("测试一下,python版的同步客户端") websocket.enableTrace(False) # 启用调试日志 ws = websocket.WebSocketApp("ws://localhost:8888", on_message=on_message, on_error=on_error, on_close=on_close, on_open=on_open) ws.run_forever()
异步客户端:
import asyncio import websockets async def receive_msg(websocket): reply = await websocket.recv() print(f"收到服务端回复:{reply}") async def send_message(websocket): message = '测试消息,python版本的异步客户端' await websocket.send(message) print('发送消息:', message) await asyncio.sleep(2) async def asyncio_tasks(url): try: async with websockets.connect(url) as websocket: # 启动发送消息的任务 send_task = asyncio.create_task(send_message(websocket)) # 启动接收消息的任务 receive_task = asyncio.create_task(receive_msg(websocket)) # 等待两个任务完成 await asyncio.gather(send_task, receive_task) except websockets.ConnectionClosedError as e: print(f"WebSocket连接关闭: {e}") # 示例使用 asyncio.run(asyncio_tasks("ws://127.0.0.1:8888"))
输出:
本文来自博客园,作者:河北大学-徐小波,转载请注明原文链接:https://www.cnblogs.com/xuxiaobo/p/18624947
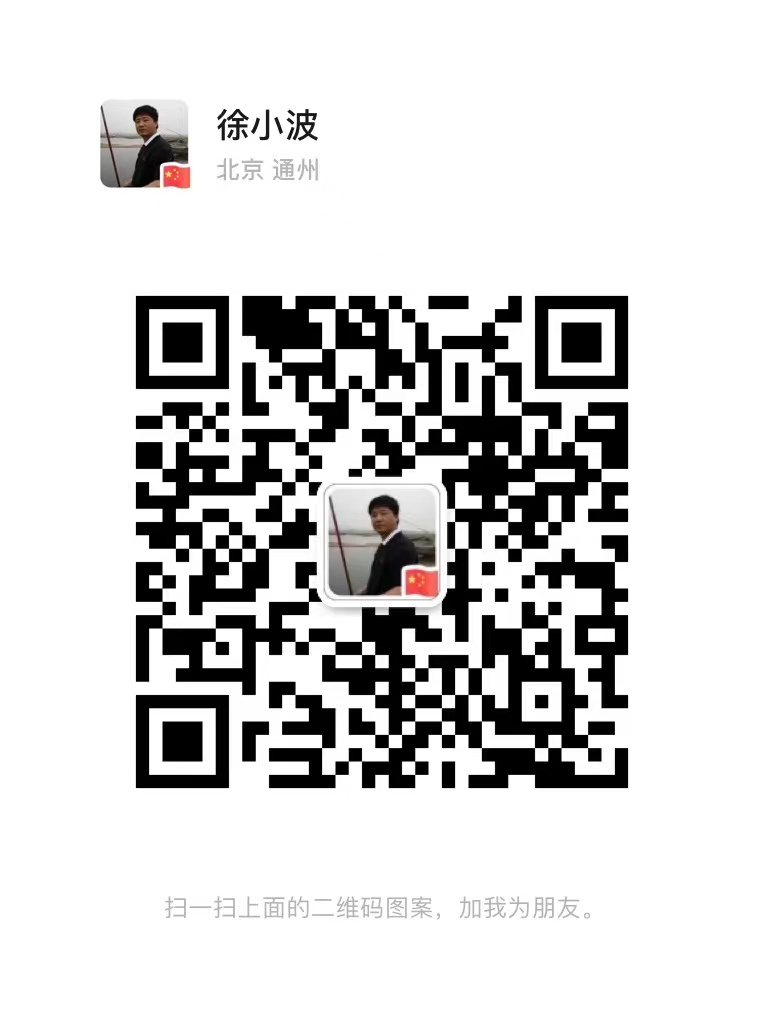