pygame各类形状
代码:
#coding=utf-8 import os,sys,re,time,math import pygame import random from win32api import GetSystemMetrics from math import pi pygame.init() pygame.display.set_caption("各种形状测试") percent = 0.6 screen_width = GetSystemMetrics(0) screen_height = GetSystemMetrics(1) window_width = int(screen_width*percent) window_height = int(screen_height*percent) dt = 0 clock = pygame.time.Clock() screen = pygame.display.set_mode((window_width, window_height)) #停止处理输入法事件 pygame.key.stop_text_input() font_path = os.path.join(os.path.dirname(sys.argv[0]), 'simsun.ttc') font = pygame.font.Font(font_path, 15) #矩形 rect_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) rect_border_width = 0 rect_position = [window_width//4+10, window_height//5+10, 50, 50] #x,y,w,h rect_percent = 400 rect_text = font.render('矩形', 1, (255-rect_color[0], 255-rect_color[1], 255-rect_color[2])) #圆形 circle_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) circle_width = 25 circle_position = [window_width//4+140, window_height//5+35] #x,y circle_percent = 350 circle_text = font.render('圆形', 1, (255-circle_color[0], 255-circle_color[1], 255-circle_color[2])) #多边形 polygon_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) polygon_position = [[window_width//4+250,window_height//5+60], [window_width//4+350,window_height//5+15], [window_width//4+255,window_height//5+160], [window_width//4+225,window_height//5+150], [window_width//4+245,window_height//5+20]] polygon_border_width = 0 polygon_percent = 300 polygon_text = font.render('多边形', 1, (255-polygon_color[0], 255-polygon_color[1], 255-polygon_color[2])) #椭圆形 ellipse_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) ellipse_rect = [window_width//4+105, window_height//5+135, 80, 50] #x,y,w,h ellipse_width = 0 ellipse_percent = 250 ellipse_text = font.render('椭圆形', 1, (255-ellipse_color[0], 255-ellipse_color[1], 255-ellipse_color[2])) #直线 line_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) line_start = [window_width//4+411, window_height//5+10] line_end = [window_width//4+420, window_height//5+85] line_width = 2 line_percent = 150 line_text = font.render('直线', 1, (255-line_color[0], 255-line_color[1], 255-line_color[2])) #抗锯齿直线 aaline_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) aaline_start = [window_width//4+411, window_height//5+110] aaline_end = [window_width//4+420, window_height//5+185] aaline_blend = 2 aaline_percent = 50 aaline_text = font.render('抗锯齿直线', 1, (255-aaline_color[0], 255-aaline_color[1], 255-aaline_color[2])) #弧形 arc_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) arc_rect = [window_width//4+520, window_height//5+10, 50, 80] #x,y,w,h arc_start = 0 arc_stop = (400/639.)*pi*2. arc_width = 3 arc_percent = 200 arc_text = font.render('弧形', 1, (255-arc_color[0], 255-arc_color[1], 255-arc_color[2])) #多个连续的直线段 lines_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) lines_closed = False lines_points = [[window_width//4+100, window_height//5+280], [window_width//4+150, window_height//5+290], [window_width//4+300, window_height//5+280], [window_width//4+320, window_height//5+230]] lines_width = 2 lines_percent = 30 lines_text = font.render('多个连续的直线段', 1, (255-lines_color[0], 255-lines_color[1], 255-lines_color[2])) #多个连续的直线抗锯齿线段 aalines_color = (random.randint(1, 255), random.randint(1, 255), random.randint(1, 255)) aalines_closed = False aalines_points = [[window_width//4+100, window_height//5+380], [window_width//4+150, window_height//5+390], [window_width//4+300, window_height//5+380], [window_width//4+320, window_height//5+330]] aalines_width = 2 aalines_percent = 10 aalines_text = font.render('多个连续的直线抗锯齿线段', 1, (255-aalines_color[0], 255-aalines_color[1], 255-aalines_color[2])) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False keys_pressed = pygame.key.get_pressed() #ESC键 if keys_pressed[pygame.K_ESCAPE]: running = False if keys_pressed[pygame.K_a]: rect_position[0] -= rect_percent * dt circle_position[0] -= circle_percent * dt for p in polygon_position: p[0] -= polygon_percent * dt for p in lines_points: p[0] -= lines_percent * dt for p in aalines_points: p[0] -= aalines_percent * dt ellipse_rect[0] -= ellipse_percent * dt arc_rect[0] -= arc_percent * dt line_start[0] -= line_percent * dt line_end[0] -= line_percent * dt aaline_start[0] -= aaline_percent * dt aaline_end[0] -= aaline_percent * dt if keys_pressed[pygame.K_d]: rect_position[0] += rect_percent * dt circle_position[0] += circle_percent * dt for p in polygon_position: p[0] += polygon_percent * dt for p in lines_points: p[0] += lines_percent * dt for p in aalines_points: p[0] += aalines_percent * dt ellipse_rect[0] += ellipse_percent * dt arc_rect[0] += arc_percent * dt line_start[0] += line_percent * dt line_end[0] += line_percent * dt aaline_start[0] += aaline_percent * dt aaline_end[0] += aaline_percent * dt if keys_pressed[pygame.K_w]: rect_position[1] -= rect_percent * dt circle_position[1] -= circle_percent * dt for p in polygon_position: p[1] -= polygon_percent * dt for p in lines_points: p[1] -= lines_percent * dt for p in aalines_points: p[1] -= aalines_percent * dt ellipse_rect[1] -= ellipse_percent * dt arc_rect[1] -= arc_percent * dt line_start[1] -= line_percent * dt line_end[1] -= line_percent * dt aaline_start[1] -= aaline_percent * dt aaline_end[1] -= aaline_percent * dt if keys_pressed[pygame.K_s]: rect_position[1] += rect_percent * dt circle_position[1] += circle_percent * dt for p in polygon_position: p[1] += polygon_percent * dt for p in lines_points: p[1] += lines_percent * dt for p in aalines_points: p[1] += aalines_percent * dt ellipse_rect[1] += ellipse_percent * dt arc_rect[1] += arc_percent * dt line_start[1] += line_percent * dt line_end[1] += line_percent * dt aaline_start[1] += aaline_percent * dt aaline_end[1] += aaline_percent * dt screen.fill("purple") #绘制正方形 pygame.draw.rect(screen, rect_color, rect_position, rect_border_width) screen.blit(rect_text, (rect_position[0]+rect_position[2]//4, rect_position[1]+rect_position[3]//3)) #绘制圆形 pygame.draw.circle(screen, circle_color, circle_position, circle_width) screen.blit(circle_text, (circle_position[0]-circle_width//2, circle_position[1]-circle_width//5)) #绘制多边形 pygame.draw.polygon(screen, polygon_color, polygon_position, polygon_border_width) centerindex = int(math.ceil(len(polygon_position) / 2.0)) screen.blit(polygon_text, (polygon_position[centerindex][0]+15, polygon_position[centerindex][1]-60)) #绘制椭圆形 pygame.draw.ellipse(screen, ellipse_color, ellipse_rect, ellipse_width) screen.blit(ellipse_text, (ellipse_rect[0]+ellipse_rect[2]//5, ellipse_rect[1]+ellipse_rect[3]//3)) #绘制直线 pygame.draw.line(screen, line_color, line_start, line_end, line_width) screen.blit(line_text, ((line_start[0]+line_end[0])//2, (line_start[1]+line_end[1])//2)) #绘制一条抗锯齿直线 pygame.draw.aaline(screen, aaline_color, aaline_start, aaline_end, aaline_blend) screen.blit(aaline_text, ((aaline_start[0]+aaline_end[0])//2, (aaline_start[1]+aaline_end[1])//2)) #绘制弧线 pygame.draw.arc(screen, arc_color, arc_rect, arc_start, arc_stop, arc_width) screen.blit(arc_text, (arc_rect[0], arc_rect[1]//2)) #绘制多个连续的直线段 pygame.draw.lines(screen, lines_color, lines_closed, lines_points, lines_width) centerindex = int(math.ceil(len(lines_points) / 2.0)) screen.blit(lines_text, (lines_points[centerindex][0]-65, lines_points[centerindex][1]-60)) #绘制多个连续的直线抗锯齿线段 pygame.draw.aalines(screen, aalines_color, aalines_closed, aalines_points, aalines_width) centerindex = int(math.ceil(len(aalines_points) / 2.0)) screen.blit(aalines_text, (aalines_points[centerindex][0]-65, aalines_points[centerindex][1]-60)) #更新显示 pygame.display.flip() #pygame.display.update() dt = clock.tick(60) / 600 pygame.quit()
效果:
参考:https://www.osgeo.cn/pygame/ref/draw.html
本文来自博客园,作者:河北大学-徐小波,转载请注明原文链接:https://www.cnblogs.com/xuxiaobo/p/18375863
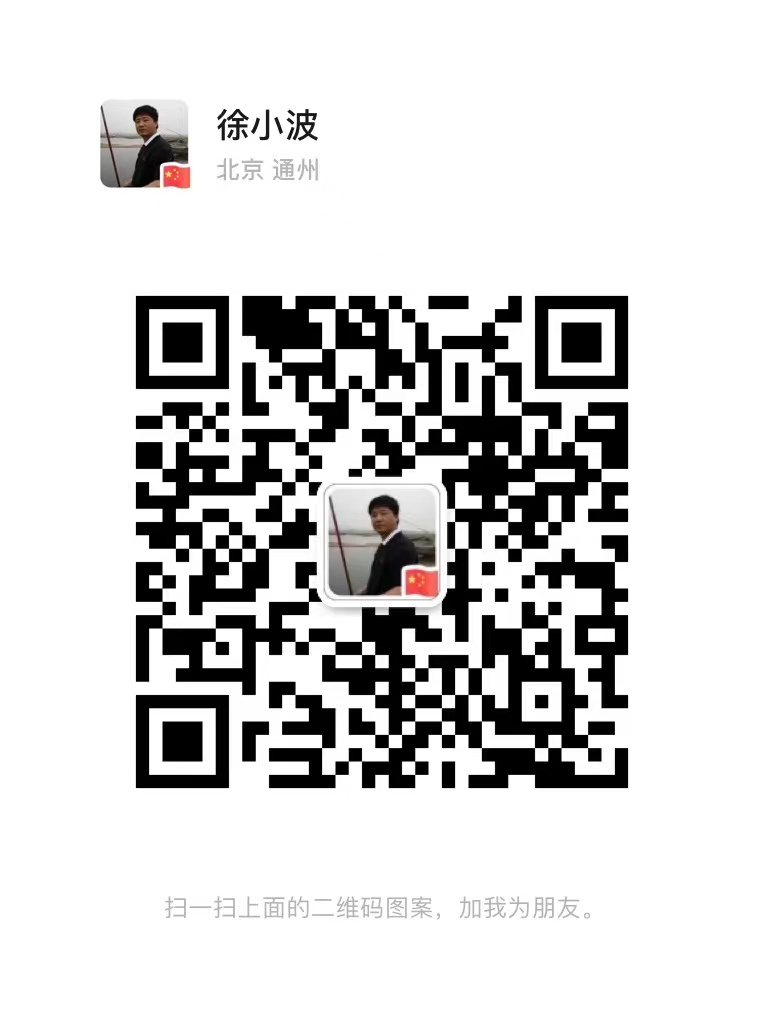